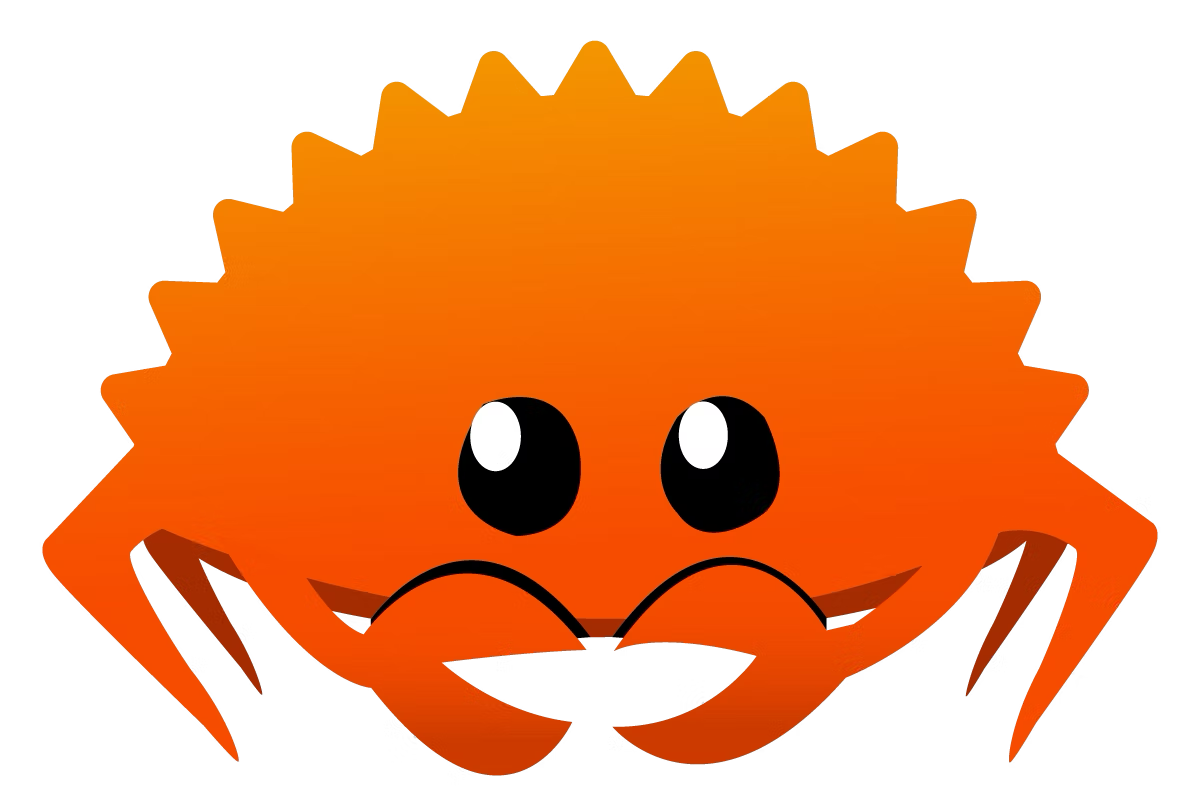
Rust – Strings
Strings
A string in Rust is a sequence of Unicode characters. In Rust, there are two types of strings: "&str" and "String".
"&str" is an immutable reference to a string slice. "&str" is stored as a constant value in memory, and you can use it by referencing a string literal or by slicing an existing String type.
On the other hand, "String" is a growable, mutable string type. It is stored on the heap, and you can use it when you need to modify the string's content.
Creating strings in Rust
There are several ways to create strings in Rust. Here are a few of them:
String literals
The most straightforward way to create a string in Rust is by using string literals. String literals are written between double quotes (").
let string = "Hello, World!";
String::from
You can also use the String::from method to create a String type from a string literal.
let string = String::from("Hello, World!");
to_string method
Another way to create a String type is by using the to_string method. This method can be used with any type that implements the ToString trait.
let string = 42.to_string();
Manipulating strings in Rust
Once you have created a string, you can manipulate it in several ways. Here are a few of them:
Concatenation
You can concatenate two strings by using the + operator.
let string1 = "Hello, "; let string2 = "World!"; let string = string1 + &string2;
Appending
You can append a string to another string by using the push_str method.
let mut string = "Hello, ".to_string(); string.push_str("World!");
Slicing
You can extract a portion of a string by using slicing. Slicing is done using square brackets ([]).
let string = "Hello, World!".to_string(); let slice = &string[0..5];
Indexing
- using chars()
You can use the chars() method to retrieve an iterator that enables unicode scalar iteration.
let hello = "Hello, world!".to_string(); for b in hello.chars() { //prints individual Chars println!("{}", b); }
- using unicode-segmentation
unicode-segmentation
is a crate that provides Unicode string-segmentation algorithms. It provides a way to split a string into its constituent Unicode grapheme clusters, which are the user-perceived characters. This can be useful when working with text that can contain complex character combinations, such as emoji or accented characters.
use unicode_segmentation::UnicodeSegmentation; let hello = "Hello, world! ?"; for grapheme in hello.graphemes(true) { println!("{}", grapheme); }
- using nth()
The nth()
method is part of the std::str and std::str::Chars traits which can retrieve the (n+1)th element in a string. nth
value acceptance starts from 0. The method takes one argument which is the index of the element to be retrieved, and returns Some(element)
on existence or None
if the index is out of bounds.
let hello = "Hello, world!"; //extracts certain character match hello.chars().nth(2) { Some(c) => println!("The 3rd character is: {}", c), None => println!("The string is too short."), }
Thank you, happy learning.