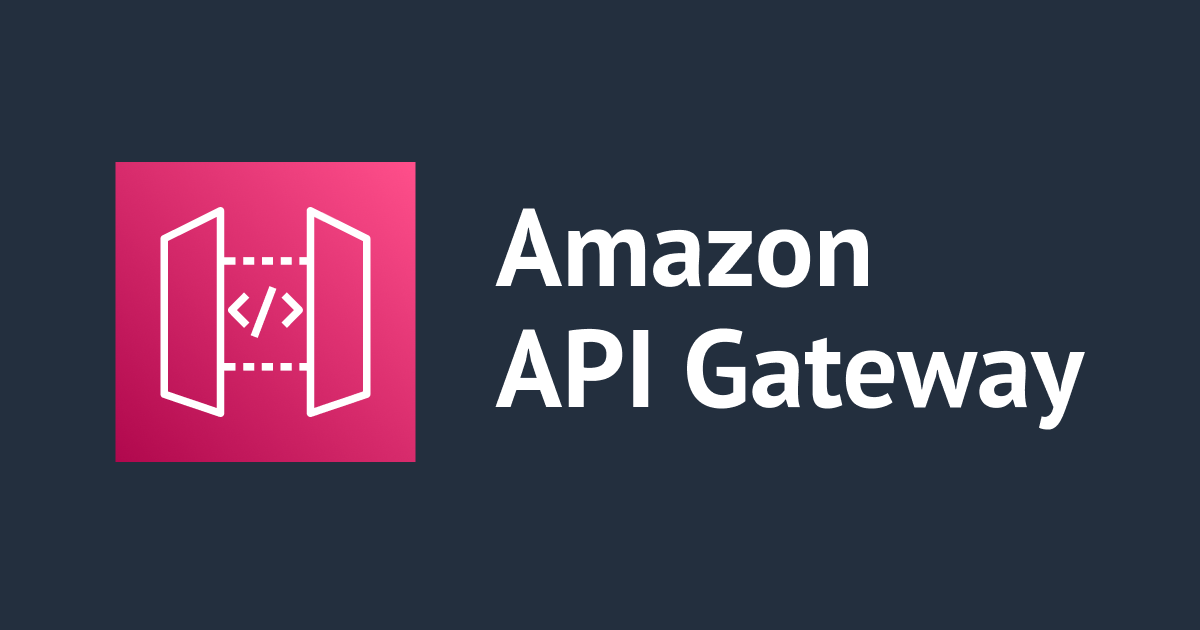
Streamlining Serverless Architecture with Lambda and API Gateway Integration
Hi. In my recent work, I tried connecting lambda with API gateway for the first time. So I thought there must be many wanting to do hands-on on these services just like me. Here is the blog in which we will explore the integration of Lambda functions with API Gateway, highlighting its benefits and providing a step-by-step guide to implement this integration.
What is AWS Lambda-
AWS Lambda is a serverless computing service that allows you to run your code without provisioning or managing servers. With Lambda, you can focus solely on writing your application logic, while AWS takes care of all the operational aspects, such as scaling, patching, and monitoring. Lambda functions are triggered by events and can be written in various programming languages, making it highly flexible and accessible.
What is API Gateway-
AWS API Gateway is a fully managed service that makes it easy to create, publish, and manage APIs at any scale. It acts as a front door for applications to access backend services or functions. API Gateway provides features like authentication, throttling, and request/response transformations, making it a robust and versatile tool for building RESTful APIs.
Let's get started-
Step 1: Create a Lambda Function-
Start by creating a Lambda function in the AWS Management Console or using the AWS CLI. Write the code for your function in the desired programming language and configure any required dependencies.
For your reference, here is my python sample code,
import json def lambda_handler(event, context): try: # Extract request parameters http_method = event['httpMethod'] path = event['path'] query_string_parameters = event['queryStringParameters'] request_body = event['body'] # Process the request if http_method == 'GET': # Handle GET request response_body = {'message': 'This is a GET request'} elif http_method == 'POST': # Handle POST request if request_body: parsed_body = json.loads(request_body) response_body = {'message': 'This is a POST request', 'data': parsed_body} else: response_body = {'message': 'Invalid request body'} else: response_body = {'message': 'Invalid HTTP method'} # Create response response = { 'statusCode': 200, 'body': json.dumps(response_body) } return response except KeyError as e: error_message = f"Missing required key: {str(e)}" response = { 'statusCode': 400, 'body': json.dumps({'error': error_message}) } return response except Exception as e: response = { 'statusCode': 500, 'body': json.dumps({'error': str(e)}) } return response
This Lambda function demonstrates basic request handling based on the HTTP method received. It supports GET and POST methods, returning different responses accordingly. For GET requests, it provides a simple message, and for POST requests, it expects a JSON payload in the request body and includes it in the response.
Step 2: Configure API Gateway-
Next, create an API Gateway REST API in the AWS Management Console. Define the desired resources, methods, and endpoints etc that will map to your Lambda function.
Within the API Gateway console, configure the integration settings for each endpoint. Select the Lambda function you created in Step 1 as the integration target. You can also define request/response mappings and configure error handling.
To create the method, you can select Get/Post from the 'Actions' drop down and select 'Create Method' option.
Step 3: Deploy API-
Once you have defined your API and integrated it with Lambda, deploy the API to make it publicly accessible. AWS will provide a unique API endpoint that you can use to invoke your Lambda function through API Gateway.
Step 4: Test and Monitor-
Test your API by making requests to the provided endpoint.
Now you can try the integration of AWS Lambda and API Gateway and build robust applications without worrying about underlying infrastructure concerns.
Thank you!
Happy Learning :)