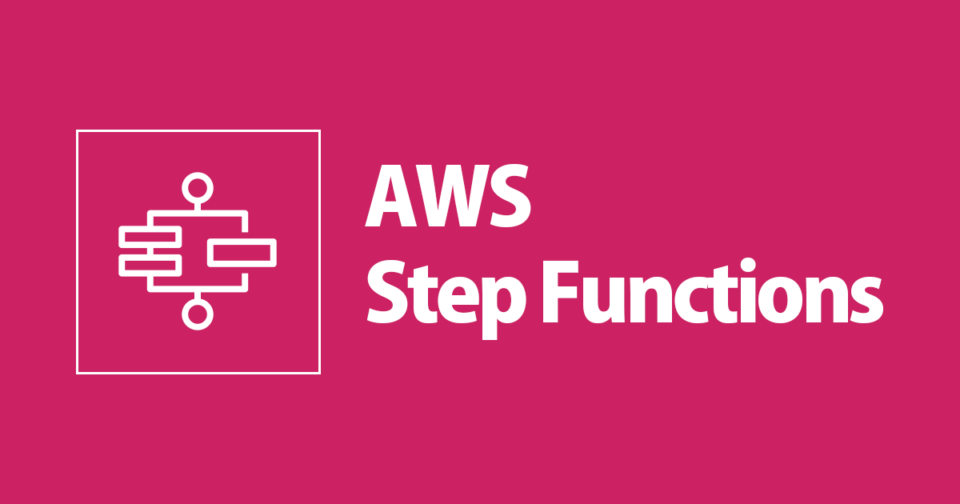
Create A Serverless Workflow Using AWS Step Functions And AWS Lambda
In this blog, you will learn how to use AWS Step Functions to design and run a serverless workflow that coordinates multiple AWS Lambda functions.
Introduction
AWS Step Functions is a low-code, visual workflow service that developers use to build distributed applications, automate IT and business processes, and build data and machine learning pipelines using AWS services. Workflows manage failures, retries, parallelization, service integrations, and observability so developers can focus on higher-value business logic.
Recently i have studied Step Functions from the following articles :
- https://aws.amazon.com/step-functions/?step-functions.sort-by=item.additionalFields.postDateTime&step-functions.sort-order=desc
- https://aws.amazon.com/jp/getting-started/hands-on/create-a-serverless-workflow-step-functions-lambda/?nc1=h_ls
- https://lumigo.io/aws-serverless-ecosystem/aws-step-functions-limits-use-cases-best-practices/
What is a Step Functions
AWS Step Functions provides serverless orchestration for modern applications. You can define and manage the workflow of your application independently using the business logic.
- It makes Application development faster and more intuitive.
- You can easily coordinate multiple Lambda functions into flexible workflows that are easy to debug and easy to change. step function can easily trigger and track each step of your application Without using Additional logic.
- Step Functions console provides a graphical representation of state machine which helps to help Visualise your application logic.
- The workflows you build with Step Functions are called State Machines and each step of your workflow is called a State.
How do Step Functions work
- Define your visual workflow in Workflow Studio. Console will auto-generate the code for you. You can also code it by yourself using code snippets in the console or locally with VS Code.
- Specify the resources that you want to trigger in your workflow.
- Start an execution to visualise and verify that the steps of your workflow are operating as intended. Inspect and debug your execution history from the console directly.
Working with Step Functions using AWS Lambda
As an example, let's create a workflow for DrinksOrderApplication. First, Visualise the workflow. It consists of three Lambda functions, a Choice state and a Fallback state.
- Order – It is the Task that use to send Order details as an input value.
- Drinks – It is the Choice State that uses the order details as the input value to select the next step (coffee or juice).
- Coffee – It is the Task implemented by the Lambda function.
- Juice – It is the Task implemented by the Lambda function.
- Fallback - To catch States.Runtime errors.
Step 1: Create a State Machine and Serverless Workflow
Open the AWSStepFunctions console. Open the AWSStepFunctions console. Click on [State Machines]. Click on [Create State Machine]. Select [Write Workflow in Code]. Click Next.
Type OrderStateMachine in the Name text box.
Select an existing IAM role that has Lambda invoke permission.
You can also create a new IAM role. Follow this step to create new IAM Role.
- Go to the Identity and Access Management (IAM) console.
- Click on [Create Role]. Select AWS Service as [Lambda]. Click Next.
- Click on [Create Policy].
Select the details as shown in the image.
- Next:Tags (It is Optional)
- Review policy: Enter the name of the policy and Click on [Create policy.]
Step 2: Create an AWS Lambda Function
Now that you have created a State Machine, you can design the workflow of your choice. For this task, you need to create three Lambda functions. Go to the AWS lambda console. Click on Create Lambda function.
Select the details as shown in the image and create a Lambda function.
Similarly you can create other lambda functions(Coffee_Function, Juice_Function)
OrderLambdaFunction
This is a Lambda function that takes the order details as an input and process it.
import json def lambda_handler(event, context): Order = { "OrderType" : "Drinks", "DrinksCategory" : event['DrinksCategory'], "DrinksType" : event['DrinksType'] } return Order
Coffee_Function
Drink_State is Choice_State. If Drink Category is coffee then the Coffee_Lambda function will get invoked. lambda function will compare the coffee details and provide the coffee price.
import json def lambda_handler(event, context): OrderType = event['OrderType'] DrinksCategory = event['DrinksCategory'] DrinksType = event['DrinksType'] Price = getPrice(OrderType, DrinksCategory, DrinksType) print("Price : ", Price) return { 'OrderType' : OrderType, 'DrinksCategory': DrinksCategory, 'DrinkType': DrinksType, 'Price': Price } def getPrice(OrderType, DrinksCategory, DrinksType): print(OrderType, " ", DrinksCategory, " " , DrinksType) if (OrderType == "Drinks" and DrinksCategory == "Coffee" and str(DrinksType) == "Cappuccino"): return "¥300" elif (OrderType == "Drinks" and DrinksCategory == "Coffee" and str(DrinksType) == "Espresso"): return "¥500" else: return "This drink is not available!"
Juice_Function
If the Drink Category is juice, the Juice_Lambda function will get invoked. lambda function will compare the juice details and provide the juice price.
import json def lambda_handler(event, context): OrderType = event['OrderType'] DrinksCategory = event['DrinksCategory'] DrinksType = event['DrinksType'] Price = getPrice(OrderType, DrinksCategory, DrinksType) print("Price : ", Price) return { 'OrderType' : OrderType, 'DrinksCategory': DrinksCategory, 'DrinksType': DrinksType, 'Price': Price } def getPrice(OrderType, DrinksCategory, DrinksType): print(OrderType, " ", DrinksCategory, " " , DrinksType) if (OrderType == "Drinks" and DrinksCategory == "Juice" and str(DrinksType) == "Orange"): return "¥300" elif (OrderType == "Drinks" and DrinksCategory == "Juice" and str(DrinksType) == "Strawberry"): return "¥500" else: return "This drink is not available!"
Step 3: Write State Machine Definition
Open the AWS Step Function console. Click on the edit. Replace the contents of the State machine definition window with the OderStateMachine definition below.
Workflow Definition
{ "Comment": "Order Process State Machine", "StartAt": "Order", "States": { "Order": { "Type": "Task", "Resource": "arn:aws:lambda:XXXX:function:orderfunction", "Next": "Drinks" }, "Drinks": { "Type": "Choice", "Choices": [ { "Variable": "$.DrinksCategory", "StringEquals": "Coffee", "Next": "Coffee" }, { "Variable": "$.DrinksCategory", "StringEquals": "Juice", "Next": "Juice" } ] }, "Coffee": { "Type": "Task", "Resource": "arn:aws:lambda:XXXX:function:coffee_function", "Catch": [ { "ErrorEquals": [ "States.TaskFailed" ], "Next": "fallback" } ], "End": true }, "Juice": { "Type": "Task", "Resource": "arn:aws:lambda:XXXX:function:juice_function", "Catch": [ { "ErrorEquals": [ "States.TaskFailed" ], "Next": "fallback" } ], "End": true }, "fallback": { "Type": "Pass", "Result": "Hello, AWS Step Functions!", "End": true } } }
Workflow Visualisation
Step 4: Test the State Machine
Start the execution to visualise and verify the steps in your workflow are working as expected. Click [Start Execution]. Provide the input value in JSON.
In the console, the Graph Inspector shows the current status of the execution. You can see the details of the state by clicking the state in the workflow diagram. You can see the execution input, execution output and state exceptions.
You can check the Execution History Log and the Status of each state. You can also check the Cloudwatch Logs for more details.
Summary
In this blog, I have introduced the basics of the AWS step function to built the sample workflow. I have created an OrderStepFunction workflow that calls a different Lambda function depending on the input provided. With AWS Step Functions, you can define your workflow as a state machine and transform complex code into easy-to-understand statements and diagrams. This makes it easy to build a multi-step system. I hope you find this blog useful. Try using Step Functions.