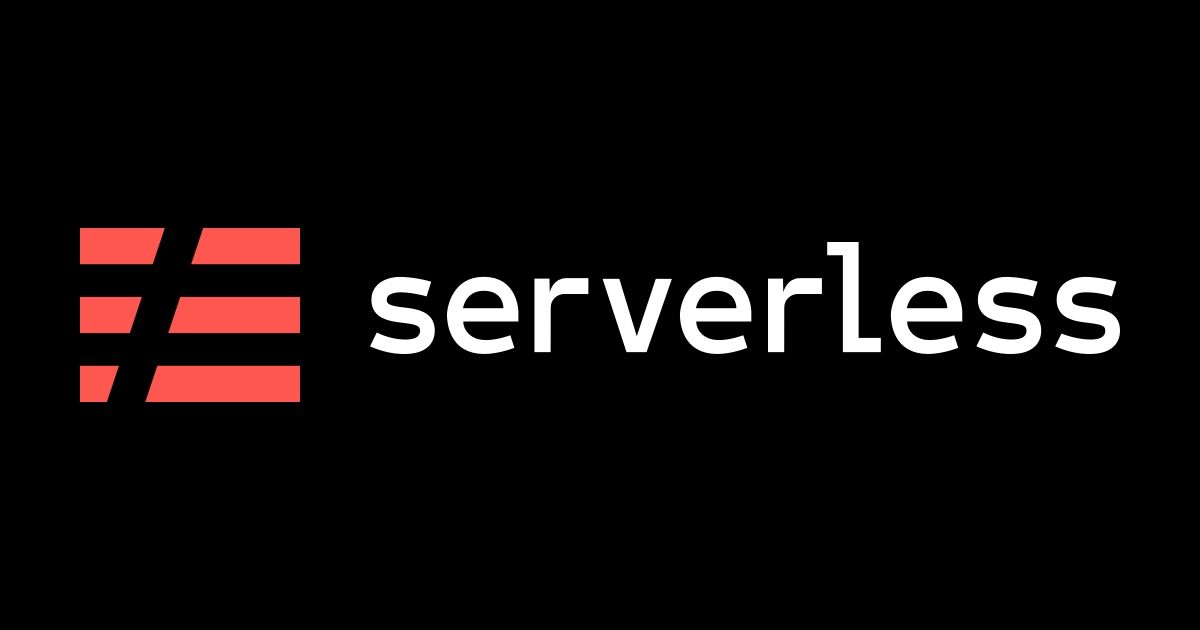
Creating API in AWS Lambda with Flask and Serverless
Introduction to Serverless
If there is a need to quickly deploy an API or have chosen to migrate your development to take advantage of the benefits of AWS Lambda, you may look to make use of Flask and the Serverless framework.
Setting Up Serverless Framework With AWS
Install the serverless
CLI via NPM:
npm install -g serverless
To create your first project, run the command below and follow the prompts:
Create a new serverless project
serverless
Move into the newly created directory
cd your-service-name
The serverless
command will guide you to:
- create a new project
- configure AWS credentials
Building the Flask App
cd to the directory of your flask app
install flask
pip install Flask
pip freeze > requirements.txt
Let's have a simple hello world Flask app
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/hello', methods=['GET'])
def helloworld():
if(request.method == 'GET'):
data = {"data": "Hello World"}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
Create a serverless.yml file in your new serverless project. This file specifies what will be deployed to Amazon, such as functions, events, and resources.
service: hello_world #name this whatever you want
provider:
name: aws
runtime: python3.9
region: ap-northeast-1
memorySize: 128
Let's begin by installing two Serverless plugins. The first step is to teach Lambda about WSGI (the interface Flask/Django uses), and the second is to have Serverless include our Python dependencies in our deployment package.
sls plugin install -n serverless-wsgi
sls plugin install -n serverless-python-requirements
The two lines are necessary, and we can see that Serverless has registered them in our serverless.yml file.
service: hello_world
provider:
name: aws
runtime: python3.9
stage: dev
region: ap-northeast-1
memorySize: 128
plugins:
- serverless-wsgi
- serverless-python-requirements
custom:
wsgi:
app: app.app
packRequirements: false
functions:
app:
handler: wsgi_handler.handler
events:
- http: ANY /
- http: 'ANY {proxy+}'
Deployment
It's as simple as entering one command. Serverless will perform the work if you run serverless deploy
in your terminal. Because of the initial preparation, the first deployment will take a little longer, but subsequent ones will be faster.