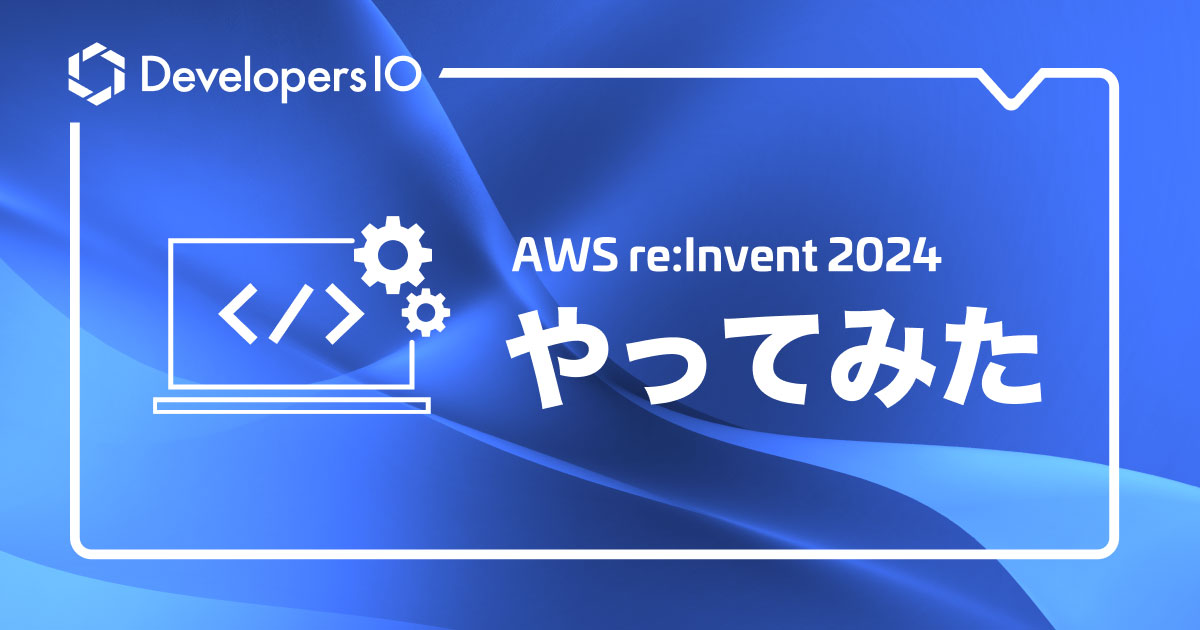
AWS Console-to-Code を試してみた
re:invent2024に参加した際に、AWS Console-to-Code というサービスを知ったので試してみました。
やってみた
EC2インスタンスの作成をしてみます。
EC2コンソール -> 右上のナビゲーションからConsole-to-Code icon -> Start recording をクリックします。
これで、AWSコンソールでの操作が記録され、作成されたAWSリソースのCLIやIaCの生成ができるようになりました。
Launch Instance wizardからEC2インスタンスを起動させます。
起動が成功したら、右上のナビゲーションからConsole-to-Codeを開き、Stop をクリックして記録を停止します。
3つのAWSリソースの作成が記録されていることが確認できます。
RunInstances を選択し、Copy CLI をクリックしてAWS CLIコマンドをコピーできます。
aws ec2 run-instances --image-id "ami-0123456789xxxxxxx" --instance-type "t2.micro" --network-interfaces '{"AssociatePublicIpAddress":true,"DeviceIndex":0,"Groups":["sg-0ecf56e92f1e2a8da"]}' --credit-specification '{"CpuCredits":"standard"}' --tag-specifications '{"ResourceType":"instance","Tags":[{"Key":"Name","Value":"My Web Server"}]}' --metadata-options '{"HttpEndpoint":"enabled","HttpPutResponseHopLimit":2,"HttpTokens":"required"}' --private-dns-name-options '{"HostnameType":"ip-name","EnableResourceNameDnsARecord":true,"EnableResourceNameDnsAAAARecord":false}' --count "1"
AWS CDK Pythonコードの生成
Generate CDK Python をクリックし CDK Pythonコードの生成します。
To generate code, Console-to-Code requires your consent to make cross-Region calls within this AWS account. You can update cross-Region preferences in your Console-to-Code settings.
コードを生成するために、Console-to-Codeは、このAWSアカウント内でクロスリージョン呼び出しを行うための同意を必要とします。Console-to-Codeの設定でクロスリージョンの設定を更新することができます。
CDK Pythonコードが生成されました。
from aws_cdk import (
aws_ec2 as ec2,
Stack,
CfnOutput
)
from constructs import Construct
class MyStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
# Create a security group
security_group = ec2.SecurityGroup(
self, "WebServerSG",
vpc=ec2.Vpc.from_lookup(self, "VPC", is_default=True),
description="Allow HTTP traffic",
allow_all_outbound=True
)
security_group.add_ingress_rule(
ec2.Peer.any_ipv4(),
ec2.Port.tcp(80),
"Allow HTTP traffic"
)
# Create an EC2 instance
instance = ec2.Instance(
self, "WebServerInstance",
instance_type=ec2.InstanceType("t2.micro"),
machine_image=ec2.MachineImage.lookup(
name="ami-0123456789xxxxxxx"
),
vpc=ec2.Vpc.from_lookup(self, "VPC", is_default=True),
security_group=security_group,
instance_initiated_shutdown_behavior=ec2.InitiatedShutdownBehavior.STOP,
credit_specification=ec2.CreditSpecification(
cpu_credits=ec2.CpuCredits.STANDARD
),
metadata_options=ec2.InstanceMetadataOptions(
http_endpoint=ec2.InstanceMetadataEndpointService.ENABLED,
http_put_response_hop_limit=2,
http_tokens=ec2.InstanceMetadataHttpTokens.REQUIRED
),
private_dns_name_options=ec2.PrivateDnsNameOptions(
hostname_type=ec2.HostnameType.IP_NAME,
enable_resource_name_dns_a_record=True,
enable_resource_name_dns_aaaa_record=False
)
)
instance.instance.add_user_data(
"#!/bin/bash\n"
"yum install -y httpd\n"
"systemctl start httpd\n"
"systemctl enable httpd\n"
)
instance.instance.instance.add_tag("Name", "My Web Server")
CfnOutput(
self, "InstancePublicIp",
value=instance.instance_public_ip,
description="Public IP of EC2 instance",
export_name="InstancePublicIp"
)
# Reasoning: This code creates an EC2 instance with the specified configuration from the provided AWS CLI commands. It creates a security group that allows inbound HTTP traffic, and then creates an EC2 instance with the specified AMI, instance type, network interface, credit specification, tags, metadata options, and private DNS name options. The instance is configured to install and start the Apache HTTP server, and its public IP address is exported as a CloudFormation output. The code follows best practices for infrastructure as code by using the AWS CDK to define the resources in a declarative and reusable way.
CloudFormationテンプレートの生成
同様に、Generate CFN YAML をクリックし、CloudFormationテンプレートを生成します。
CloudFormationテンプレートが生成されました。
Resources:
EC2Instance:
Type: AWS::EC2::Instance
Properties:
ImageId: ami-0123456789xxxxxxx
InstanceType: t2.micro
NetworkInterfaces:
- AssociatePublicIpAddress: true
DeviceIndex: 0
GroupSet:
- sg-0ecf56e92f1e2a8da
CreditSpecification:
CPUCredits: standard
Tags:
- Key: Name
Value: My Web Server
MetadataOptions:
HttpEndpoint: enabled
HttpPutResponseHopLimit: 2
HttpTokens: required
PrivateDNSNameOptions:
HostnameType: ip-name
EnableResourceNameDnsARecord: true
EnableResourceNameDnsAAAARecord: false
Reasoning: {The provided AWS CLI command runs an EC2 instance with the specified properties. The generated CloudFormation YAML code creates an EC2 instance resource with the same properties as specified in the CLI command. The ImageId, InstanceType, NetworkInterfaces, CreditSpecification, Tags, MetadataOptions, and PrivateDNSNameOptions properties are mapped directly from the CLI command to the CloudFormation resource properties. The count parameter is not included as CloudFormation creates a single resource by default.}
プレビューコード
先ほどは、EC2インスタンスを起動しするコンソール操作をレコーディングしてコードに変更しましたが、EC2インスタンスと Auto Scalingグループの起動ウィザードに Preview code 機能があります。実際にリソースを作成しなくてもコードを生成することが可能です。
EC2起動ウィザード
EC2起動ウィザードで Launch instance の下にある Preview code をクリックします。
Console-to-Code が表示されコードを確認することができます。
Auto Scalingグループの起動ウィザード
Auto Scalingグループの起動ウィザードも同様に、Review のページで、Preview code をクリックします。
Console-to-Code が表示されコードを確認することができます。
まとめ
AWS Console-to-Codeを使用して、AWSマネジメントコンソールの操作から、CLIやCDK、CloudFormationテンプレートを作成してみました。現在はEC2、RDS、VPCで使用が可能のようです。またプレビューコード機能によりリソースを作成せずに、コードを生成することができました。