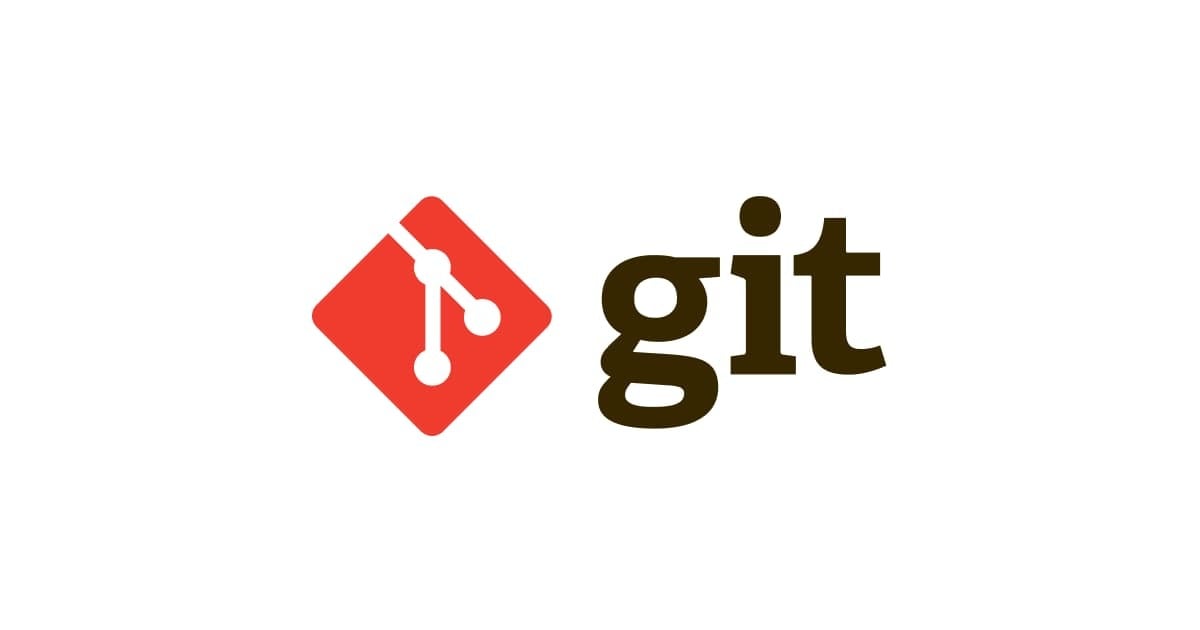
Mastering Version Control: Git Best Practices for Seamless Collaboration.
Introduction.
Version control systems are essential tools for developers, allowing teams to track changes, revert to previous states, and collaborate on software projects. Git, one of the most popular version control systems, is highly valued for its flexibility, speed, and efficiency in handling small to large projects. This blog discusses best practices for using Git to ensure seamless collaboration and maintain a clean, efficient workflow.
Introduction to Git.
Git is a distributed version control system that allows multiple developers to work on the same project without being connected to a central server. It is designed to handle projects with speed and efficiency, providing robust tools for branching, merging, and rewriting project history.
Setting Up a Repository.
Step 1: Initialize a New Repository.
- Open your terminal.
- Navigate to your project directory using
cd /path/to/your/project
. - Initialize the repository with
git init
.
Step 2: Create a .gitignore
File
- In your project directory, create a
.gitignore file
. - Add file patterns for files you don’t want to track (e.g.,
*.log
,node_modules/
). - Save and close the file.
- Run git add
.gitignore
andgit commit -m "Add .gitignore".
Branching Strategies.
Why Use Branching?
Branching allows you to diverge from the main line of development and continue to work independently without affecting the main line.
Popular Branching Strategies:
- Git Flow: Involves having a master, develop, feature, release, and hotfix branches.
- Feature Branch Workflow: Each new feature is built on its own branch and merged into the main branch upon completion.
Creating and Using Branches:
- Create a new branch with
git branch new-feature
. - Switch to your branch with
git checkout new-feature
. - After development, merge the branch with
git checkout main
andgit merge new-feature
.
Committing Best Practices.
Writing Meaningful Commit Messages:
- A good commit message should be clear and descriptive.
- Example:
git commit -m "Implement user authentication system"
Structuring Good Commits:
- Each commit should represent a single logical change.
- Test your changes before committing.
Commit Frequency:
- Commit often. Small, frequent commits are easier to understand and roll back if something goes wrong.
Pull Requests and Code Reviews.
Creating a Pull Request (PR):
- Push your branch to a remote repository with
git push origin new-feature
. - On GitHub, navigate to your repository and click on "Compare & pull request."
- Add a title, description, and select reviewers before creating the pull request.
Conducting Code Reviews:
- Review code for clarity, standards, and errors.
- Provide constructive feedback and request changes if necessary.
Merging vs. Rebasing:
- Merging: keeps the history of feature branches. Use
git merge
. - Rebasing: rewrites history to create a linear progression. Use
git rebase
.
Handling Merge Conflicts.
Avoiding Merge Conflicts:
- Keep your branches short-lived.
- Regularly merge changes from the main branch into your feature branch using
git merge main
.
Resolving Conflicts:
- Git will alert you to conflicts during a merge.
- Open the conflicting files and make the necessary changes.
- After resolving, mark as resolved with
git add <file-name>
, and complete the merge withgit commit
.
Security Practices.
Keep Sensitive Data Out:
- Never commit sensitive data like passwords or API keys.
- Use environment variables and config files that are not tracked by Git.
Using Git Hooks:
- Implement pre-commit hooks to run tests or check for sensitive data automatically.
For further reading you can visit the link below. https://docs.github.com/en/issues/planning-and-tracking-with-projects/learning-about-projects/best-practices-for-projects
Conclusion.
Adopting these Git best practices will not only improve your workflow but also enhance collaboration across your development team. Remember, the key to mastering Git is consistent practice and continuous learning. Happy coding!