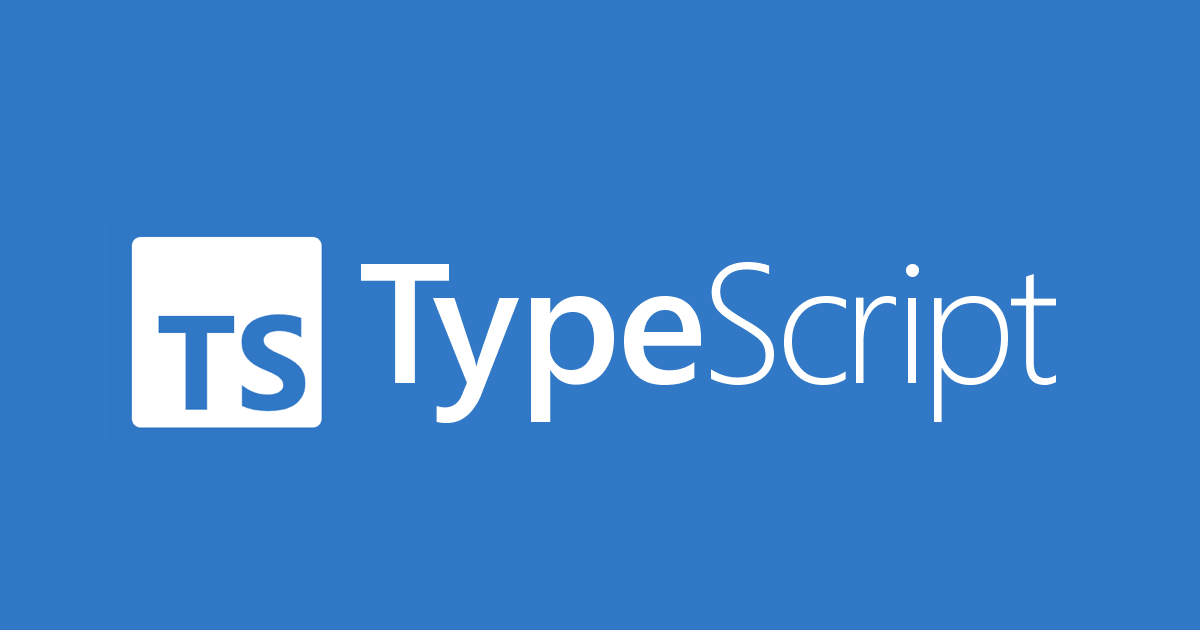
Begin with TypeScript – If statements and loops!
Introduction : The Basics
TypeScript being one of the world’s most used tools is an open-source language, that builds on JavaScript and adds static type definitions. In this blog, we start with the basics of TypeScript with its object-oriented functionality. We later go through the looping features using TypeScript and its applications. Let's Begin!
TypeScript provides all of JavaScript’s features, with an extra feature : TypeScript’s type system. TypeScript checks consistently if the JavaScript provided language primitives like string and number are assigned correctly or not.
On the aspect of types, in JavaScript, there are already a set of primitive types that are readily available which include boolean, bigint, null, number, string, symbol, and undefined which can be used in an interface. TypeScript adds a few more, such as any (allows everything), unknown (ensures type is declared) , never (possibility of this type not happening ), and void (a function which has no return value or returns undefined). With TypeScript, we can create complex types by combining simple ones. There are two popular ways to do so: with Unions, and with Generics.
TypeScript mainly highlights the unnatural behavior in the code that helps in lowering the chance of bugs. Typescript is also very flexible for editing codes, and can help with error messages and in the completion of code (Tooling). Also, Typescript is an ES6 version of Javascript with additional features. Firstly, for the usage of Typescript, it should be installed on the local computer. Typescript can be installed with NPM using the following command on the terminal.
npm install -g typescript
Currently, there are various text editors and IDE available through plugins, which supports Typescript. Some of the text editors are Vim, Visual Studio Code, Atom.
TypeScript : Object-Oriented
A very important feature of Typescript is that its follows object-oriented programming. In JavaScript, sometimes while using function-objects, there is a lot of repetitive code. Therefore, class was added to ES6 In Typescript, we can define classes and interfaces, For example:
class Employee { name : string = ' ' //for default code: number = 0 //for default getEmployeeDetails() { return this.name + ' ' + this.code } } //To create a new instance for the class Employee const employee1 = new Employee() employee1.name = 'Deekshita Savanur' employee1.code = '1234' console.log(employee1.getEmployeeDetails())
Here, a class Employee has been created. Once the Employee() object is created, it is not necessary to declare its type once again. This is automatically done by TypeScript.
TypeScript : if - else statements
By taking examples of items being ordered by users and stocks that are available in a warehouse, the following statements are explained with code.
If Statement :
In TypeScript, for if statements, one or more expressions can be used which returns boolean. If the boolean expression item evaluates to be true, a set of statements is then executed.
let item: number = 50, stock = 100; if (item<stock) { console.log(' proceed to purchase ') }
In the above example, the if condition item< stock expression is evaluated to be true and so it executes the statement within the block of curly { } brackets.
If-else Statements :
An if-else condition includes two segments - if segment and an else segment. If the IF condition evaluates to be true, then the IF segment is executed. Otherwise, the ELSE segment is executed.
let item: number = 50, stock = 100; if (item<stock) { console.log(' proceed to purchase '); } else { console.log(' stock is unavailable '); }
In the above example, the else block will be executed.
else-if Statements :
The else if statement can be used after the if statement.
let item: number = 100, stock = 50; if (item<stock) { console.log('proceed to purchase'); } else if (item>stock) { console.log('stock is unavailable'); //This will be executed } else if (item == stock) { console.log('stock is available'); }
Ternary operator :
A ternary operator is denoted with '?' and is used as a shorter code for an if-else statement. It first checks for a boolean condition and executes one of the two statements, depending on the result of the boolean condition.
let item: number = 100, stock = 50; item>stock? console.log('stock is unavailable'): console.log('proceed to purchase')
In the above example, condition item > stock is true, so the first statement will be executed.
TypeScript : switch statements
The switch statement is used to check for multiple values and executes sets of statements for each of those values. A switch statement has one segment of code corresponding to each value and can have any number of such segments. When the match to a value is found, the corresponding segment of code is executed. Let us go through some of the rules for switch statements. The switch statement can include constant or variable expressions which can return a value of any data type. There can be any number of case statements within a switch. The case can include a constant or an expression. The default block is optional.
let day : number = 3; switch (day) { case 0: console.log("It is a Sunday."); break; case 1: console.log("It is a Monday."); break; case 2: console.log("It is a Tuesday."); break; case 3: console.log("It is a Wednesday."); break; case 4: console.log("It is a Thursday."); break; case 5: console.log("It is a Friday."); break; case 6: console.log("It is a Saturday."); break; default: console.log("No such day exists!"); break; }
Result: It is a Wednesday.
In the above example, we have a variable, day whose type is number. This variable has been initialized with a value of 3. This value corresponds to a day of the week. A switch statement checks the value and executes the segment of code corresponding to that value. This results in the code block for value 4 being executed, giving the result, 'It is a Wednesday.'
Looping with TypeScript
With the basic understanding of classes, if-else, and switch statements, we can begin with looping using TypeScript.
TypeScript - for Loops :
The for loop is used to execute a block of code a given number of times, which is specified by a condition. In Typescript, for loop has the same syntax as other programming languages:
for(initial_value, specific_condition, step_increment)
The Parameters:
- intial_value: This parameter is used to specify the initial count value for the declared variable to start the iteration count and keep the track of the iteration.
- specific_condition: This parameter is used to specify the logical condition where if this condition is true then it executes the code within the loop.
- step_increment: This parameter is used to specify the count to repeat the set of code when the condition is true.
for (let i = 0; i < 5; i++) { console.log ("Execution of statement no." + i); }
Output:
Execution of statement no.0 Execution of statement no.1 Execution of statement no.2 Execution of statement no.3 Execution of statement no.4
In the above example, the first statement let i = 0 declares and initializes the variable. The second conditional statement i < 5 checks whether the value of i is less than 5 or not, and if it is not then it exits the loop. The third statement i++ increases the value of i by 1. Therefore, the above loop will execute the block of code five times, until the value of i becomes 5.
How does “for” loop work in Typescript?
In typescript, the “for” loop is a control-flow statement that is used in iterations to execute a particular block of code that is under the “for” loop. The for loop's first statement consists of 3 different expressions which is used to define the count of iterations to start, from which it initializes the variable and this is executed only once; the second part is used to specify the condition to execute the code within the loop if the condition is true, else the loop does not enter the loop segment and if the condition as a whole is false then the “for” loop gets terminated, and the third part is used for setting the count of increment of the iteration. This condition is executed on loop, where the “for” loop continues to execute the set of code until the condition fails and the “for” loop exits the iteration and the loop gets terminated.
TypeScript - while loop
while loop statement :
The while loop is another type of loop that checks for a specified condition before beginning to execute the block of statements. The loop runs until the condition is true.
let item: number = 5; while (item < 8) { console.log( "Execution of statement no." + item ) i++; }
Output:
Execution of statement no.5 Execution of statement no.6 Execution of statement no.7
In the above example, we declared a variable i with the value 5. The while loop checks for the value of i before executing the while code block. If i < 8, then it executes the block. Within the block of code, we have a statement to increment the value of i by 1. This results in the while loop runs three times, for i = 5, i = 6 and i = 7.
do-while loop statement:
The do-while loop is similar to the while loop, where the condition is given at the end of the loop. The do-while loop runs the block of code at least once before checking for the given condition. For the rest of the iterations, it runs the block of code only if the specified condition is true.
let item: number = 2; do { console.log("Execution of statement no." + item ) i++; } while ( item < 4)
Output :
Execution of statement no.2 Execution of statement no.3
In the above code, we have a variable item initialized with a value 2. The loop runs the block of code once, prints "Execution of statement no.2" and increments the item to value 3. The loop then checks for the condition item < 4. Since item < 4, it runs the loop again, this time printing "Execution of statement no.3" and then incrementing the value of the item to 4. After increment, the condition item < 4 is executed as false, which terminates the do-while loop.
Here, we end our learning on looping with Typescript.
Conclusion
In this blog, we began with learning the basics of TypeScript and the Object-oriented feature of TypeScript. We further continued with the if-else and switch statements usage with code implementation. Further, the looping using for and while statements were introduced. Hope this helps you to start programming with TypeScript!