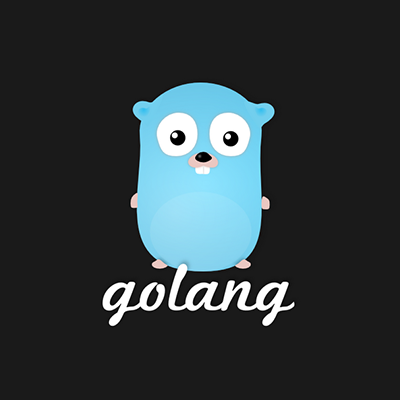
Golangでini形式のファイルを読み書きしてみる
はじめに
以下のようなini形式で書かれているファイルをGolangで読み書きできたらいいと思うケースがあり、やってみました。AWS CLIのクレデンシャルファイルなどでもお馴染みの形式だと思います。
[connections] accountname = your.account.name username = your_user_name
作成したもの
プログラムと動作について
ini形式のファイル(ini.config)を読み書きするGolangのプログラムを作成しました。それぞれ以下となります。
[connections] accountname = your.account.name username = your_user_name password = your_password port = 3000 [options] parallel = true
package main import ( "fmt" "log" "gopkg.in/ini.v1" ) type Connections struct { AccountName string UserName string Password string Port int } type Options struct { Parallel bool } func main() { cfg, err := ini.Load("ini.config") if err != nil { log.Fatal(err) } cons := Connections{ AccountName: cfg.Section("connections").Key("accountname").String(), UserName: cfg.Section("connections").Key("username").String(), Password: cfg.Section("connections").Key("password").String(), Port: cfg.Section("connections").Key("port").MustInt(), } fmt.Printf("cons = %v\n", cons) options := Options{ Parallel: cfg.Section("options").Key("parallel").MustBool(), } fmt.Printf("options = %v\n", options) cfg.Section("add").Key("first").SetValue("first value") cfg.Section("add").Key("second").SetValue("12345") cfg.SaveTo("ini.config") }
「main.go」を「$ go run main.go」などで実行すると、以下のように実行結果として読み込んだ値が表示され、「ini.config」には「add」セクションが追加されます。
$ go run main.go cons = {your.account.name your_user_name your_password 3000} options = {true}
[add] first = first value second = 12345
プログラムについて解説
ini形式のファイルを読み書きするのに、 gopkg.in/ini.v1 を使用しています。上記のソースを実行する前に、以下のコマンドでインストールしておく必要があります。
$ go get gopkg.in/ini.v1
「ini.config」には「connections」「options」という2つのセクションを定義してありますが、それぞれの値を格納するための構造体を10〜19行目で宣言し、 26〜37行目で読み込み・ターミナルへの表示をしています。
39行目からは、読み込んだとの同じ「ini.config」に「add」セクションを追加で出力しています。
このようにini形式のファイルへの読み書きができました。
まとめ
先にも書きましたが、ini形式のファイルはクレデンシャルの定義などで良くある形式だと思います。特定製品に対応したライブラリなどで(ini形式の)クレデンシャルの読み書きを行う機能がない場合など、独自で読み書きをすることもあるかと思います。そのような場合などに、本記事が役に立てば幸いです。