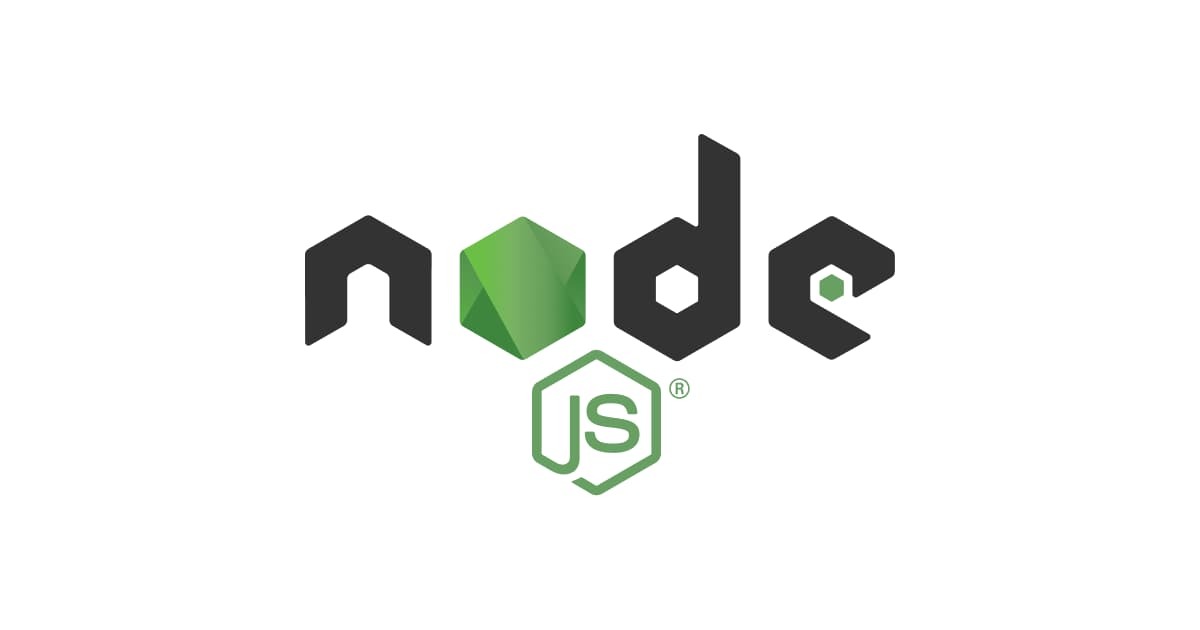
I solved ‘Cannot find module ‘aws-serverless-express” error
Hi, this is Charu from Classmethod. In this short blog, we will talk about how to solve 'Cannot find module 'aws-serverless-express'' error.
Recently I was working with the making of AWS serverless application. I thought of using express.js as the framework. Since I was new to express, I read it's official documentation and implemented it.
To start with it, I installed express using the following few commands-
npm install aws-serverless-express
npm install express
I made the other necessary changes in my code, and deployed the CDK. But I got the error saying 'Cannot find module 'aws-serverless-express''. I was wondering what might be the reason, because I have correctly installed the packages and it was up-to-date.
After doing some research and analysing, I got to know the reason.
I will show you my project structure-
- node_modules
-src
- express
- app.js
- lambda.js
- web
- index.html
- main.js
- package.json
- package-lock.json
And this error usually happens when the module is not installed or is not included in the deployment package.
When you run cdk deploy, AWS CDK packages your Lambda function code and any necessary node_modules into a .zip file and uploads this to AWS Lambda. However, by default, AWS CDK only includes the node_modules that are in the same directory as your Lambda function code or in a parent directory.
If you ran npm install aws-serverless-express in the root directory of your project, but your Lambda function code is in a subdirectory, the aws-serverless-express module might not be included in the deployment package.
To fix this issue, you can install the aws-serverless-express module in the same directory as your Lambda function code.
To solve the issue, navigate to the directory containing your Lambda function code using the cd command. In my case, it will be-
cd src/express
Install the aws-serverless-express module-
npm install aws-serverless-express
Navigate back to the root directory of your project-
cd ../..
And deploy your CDK.
This ensures that the aws-serverless-express module is in the node_modules directory that's in the same directory as your Lambda function code, so it gets included in the deployment package.
Now, your project structure should be like this-
- node_modules
-src
- express
- node_modules
- app.js
- lambda.js
- package-lock.json
- package.json
- web
- index.html
- main.js
- package-lock.json
- package.json
If the node_modules directory is not being created in the src/express directory, it's possible that you have a package-lock.json file in a parent directory that's causing npm to install the modules there instead.
Now, to solve this issue, you need to remove your node_modules folder and package-lock.json file and install it again. Like this,
cd src/express
rm -rf ../../node_modules ../../package-lock.json
Install the aws-serverless-express module again,
npm install aws-serverless-express
Navigate back to the root directory of your project and deploy.
Update:
Here is an update regarding aws-serverless-express package. The aws-serverless-express package has been replaced by @vendia/serverless-express. You can know more about it here.
To install it, you can run-
npm install @vendia/serverless-express
And to use it in your lambda function, you can write your function like this,
const serverlessExpress = require('@vendia/serverless-express'); const app = require('./app'); const server = serverlessExpress.createServer(app); exports.handler = (event, context) => { console.log(`EVENT: ${JSON.stringify(event)}`); return serverlessExpress.proxy(server, event, context); };
Hope you found this blog helpful. Thank you for reading!
Happy Learning:)