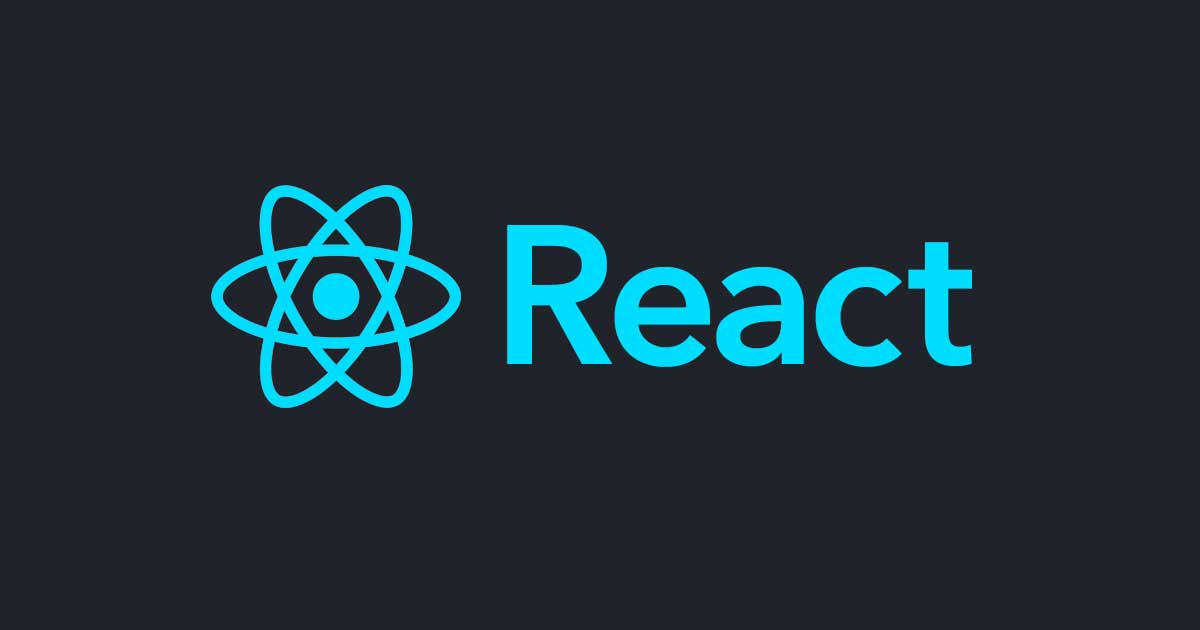
Material UI – Components & Implementation
Introduction
React is a popular open-source, front-end JavaScript library used for building single-page web applications. This includes building user interfaces, handling the UI for web and mobile apps. React also can be used to create reusable UI components. The main purpose of React is to be fast, scalable, and simple.
Material UI is an open-source, front-end framework for these React components. It is based on Google's material design to provide the best layouts while developing front-end graphics. The advantages of designing with Material UI include :
- Material UI has detailed documented information that makes it easy to navigate through the framework.
- It is actively maintained.
- The components of Material UI are consistent in design, which allows the developed web application to look structured.
Getting started with Material UI
To begin with Material UI, we first create a new React App using the create-react-app command as shown below:
npx create-react-app materialui-test-app --template typescript or yarn create-react-app materialui-test-app --template typescript
The next step is to add Material UI to the React project.
yarn add @material-ui/core
We can now proceed with importing different components of Material UI into the code and implementing it.
Material UI - Components & Implementation
Firstly, the Material UI is structured into different categories based on the developer's needs. For designing the layout, the options include Box, Container, Grid, Hidden, Image List. For example, to implement the Box Component of the Material UI, the code can be written as :
import * as React from "react" import Box from "@material-ui/core/Box" export const BoxExample: VFC = () => { return ( Components inside the box ) }
In the above example, by adding padding to the style of the Box Component, we get the output as below. The procedure can be followed for adding the other layout design options.
The next category of Material UI Components includes the types of Inputs: Button, Button Group, Checkbox, Floating Action Button, Date/Time, Radio, Select, Slider, Switch, Text Field, Transfer List.
For example, to implement the Button the following command can be written :
import * as React from "react" import { Button } from "@material-ui/core" export const ButtonExample: VFC = () => { return ( <div><button> {" "} Click Me!{" "} </button></div> ) }
The above code snippet gives the output of a Button with "Click!" on it. We can use props to style buttons, as well as other components. Props are essential properties that are passed down to an element. The variant variable of the component helps to define how the Button should appear.
There are many components of Material UI that help displaying data : Text Field, Divider, Icon, List, Table, Typography, etc. To implement the TextField, we can first import from @material-ui/core library and the following command can be written :
<TextField id="employee" label="Name" variant="outlined" />
We can also implement many Material UI components in a nested procedure as shown below.
import * as React from "react" import { AppBar, Toolbar, Typography } from "@material-ui/core" export const MaterialuiExample: VFC = () => { return ( Navigation Bar Example using React ) }
The above example displays a Navigation Bar displaying "Navigation Bar Example using React". The AppBar, Toolbar, and Typography are all part of the Material-UI widgets. The Typography widget helps highlight the items that appear in the toolbar.
Conclusion
The blog begins with the Introduction to Material UI. Later, the different types of Material UI components are being discussed. This information can be used to build complex applications that adhere to material design principles. By using the Material-UI component library, its easy to enhance the output of the React application with Google’s Material Design.
Happy Coding!