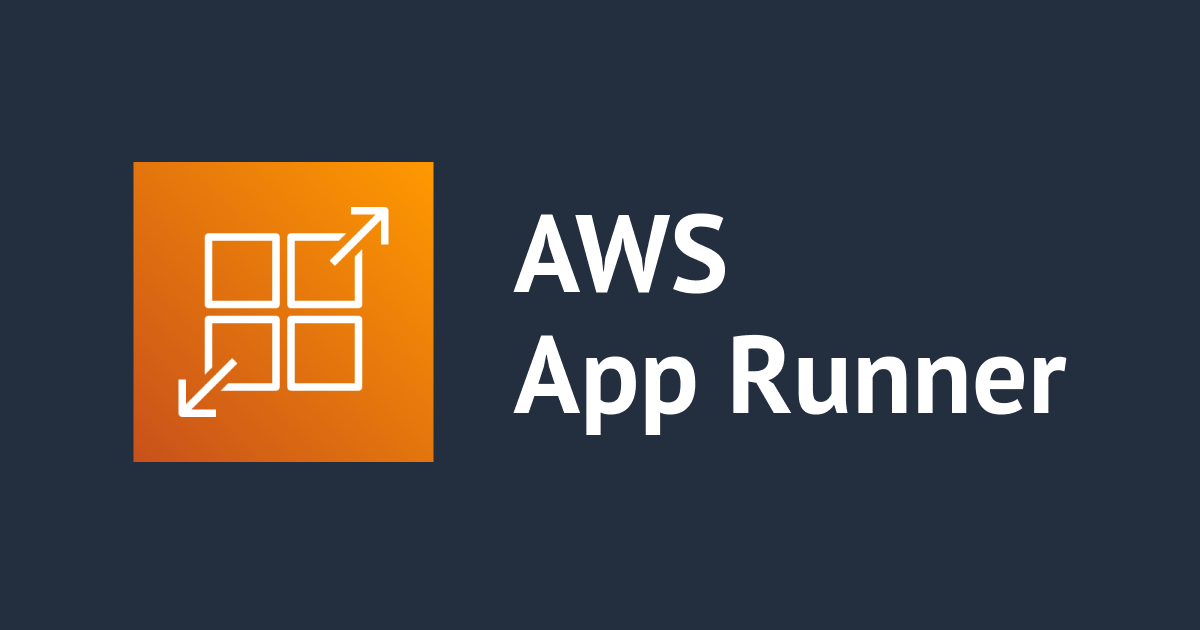
Sample Next.js Liff App deployed on AWS AppRunner
In this blog, I’m walking you through the steps to deploy a sample Next.js project with the LIFF integration on AWS AppRunner.
AWS AppRunner
AWS AppRunner is a fully managed service that makes it simple and easy to deploy containerised web applications or APIs.
Here are some features:
Step 1: Sample Next.js project set-up
npx create-next-app@latest or yarn create next-app
Refer official documentation for more information here.
Step 2: Line Login integration
For enabling LINE login, we shall call the LIFF API.
To install the LIFF SDK via npm and import it into your app, follow these steps:
npm install --save @line/liff or yarn add @line/liff
Implementation:
Import the SDK into your app and Include the following code in your JavaScript or TypeScript file:
pages/_app.js
import '../styles/globals.css'; import { useEffect } from 'react'; const liffId = process.env.NEXT_PUBLIC_LIFF_ID; //add your own LiffID function MyApp({ Component, pageProps }) { useEffect(() => { async function liffLogin() { const liff = (await import('@line/liff')).default; try { await liff.init({ liffId }); } catch (err) { console.error('liff init error', err.message); } if (!liff.isLoggedIn()) { liff.login(); } } liffLogin(); }, []); return <Component {...pageProps} />; } export default MyApp;
Also, check the official documentation of Line developers for more information here.
For the LiffID:
This blogs aims at sample Nest.js project with Line intergration which is also called LIFF applications that run on LIFF browser and LINE mini-app. In the above piece of code, we have called liff.init ()
API with the LiffID to enable the LINE Login authentication.
To get the LiffId, we should create a provider and channel in the LINE Developers Console. For detailed step-by-step explanation please see here.
About environment variables:
Since LiffID is a secret key, I stored the key-value in an .env file. Next 9.4 has built-in support for environment variables i.e. .env
files. For Next.js's environment variables specification make sure to proceed your keys with NEXT_PUBLIC_
.
As an example for local development, create a .env.local
file. Add the key-value pair.
NEXT_PUBLIC_LIFF_ID=16*********k
While referring the key-value in the code, do as the following:
const liffId = process.env.NEXT_PUBLIC_LIFF_ID;
For more information about environment variables here .
Step 3: Deploy on AppRunner
Login to your AWS account
Search for AppRunner. Create a new App Runner service.
Source and Deployment
Add the repository type and accordingly add the source code provider. If the repository type is Container registry, add the Type of ECR provider and add the container image URI. In our case the repository type is a source code repository, hence I linked my Github. Make sure you specify the correct repository and branch.
To link your Github account
Click on add new and it'll now create a connection with GitHub using AWS CodeStar.
Deployment Settings
Set it to automatic or manual. I set it to automatic, so on every push to the branch specified a new version is launched automatically.
Build Configure Settings
Here, you can either configure all settings in the console manually or attach a file.
- If configuring in the console, simply fill in the right commands.
-
If attaching a file.
Add
apprunner.yaml
file to the root of your repository as shown below.
version: 1.0 runtime: nodejs12 build: commands: build: - yarn - yarn build run: command: yarn dev network: port: 3000
Configure Service
We can configure things like computing CPU, memory, environmental variables, auto-scaling, health checks, and tags. For the current example, I have kept all the settings as default. Except for naming the service and setting environment variables.
Review and Create
This step takes a few minutes as App Runner dockerizes our app and runs it on the server. If you are using a docker image already then this step will be instant.
Congratulations, its Deployed!
Our application has been deployed and running successfully. We can access our service via the default domain URL given by the App Runner.
Thanks for reading, I hope it was helpful.