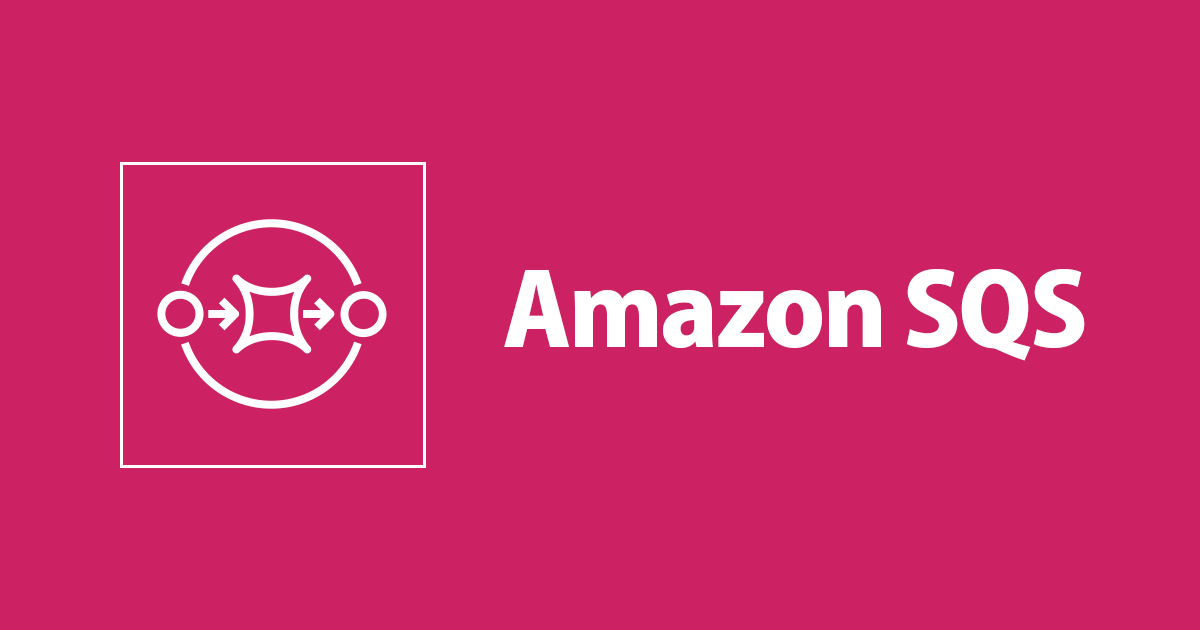
Sending, Receiving And Deleting Amazon SQS Messages Using Lambda Function
This blog describes how to send, receive and delete Amazon SQS messages using lambda function.
Preparation
To set up and run this task, you must create the Lambda function and SQS queue .
Create a lambda function. Refer this for creating lambda function, see Create lambda function(console)
Create an Amazon SQS queue. Refer this for creating an Amazon SQS queue, see Create a queue(Boto3), Create a queue(console).
Send Messages
Send Message to specific Amazon SQS queue by calling the AmazonSQS client’s send_Message method. Define a SendMessageRequest object that contains the queue URL and message body. you can also assign delay value (in seconds) and Attributes(it is optional).
import boto3 import json def lambda_handler(event, context): sqs = boto3.client('sqs') message = {"key": "value"} response = sqs.send_message( QueueUrl='****', MessageBody=json.dumps(message) ) print(response) return message
Parameters
QueueUrl (string) - The URL of the Amazon SQS queue to which a message is sent. Queue URLs and names are case-sensitive.
MessageBody (string) - The message to send. The minimum size is one character. The maximum size is 256 KB.
Receive Messages
Retrieve any messages that are present in the queue by calling the AmazonSQS client’s receive_Message method and Pass queue URL, AttributeNames and MaxNumberOfMessages. This code will return a received message and count of messages in a queue.
import boto3 import json def lambda_handler(event, context): sqs_client = boto3.client('sqs') response = sqs_client.receive_message( QueueUrl='****', AttributeNames=['All'], MaxNumberOfMessages=10) print(f"Number of messages received: {len(response.get('Messages', []))}") message = json.loads(response['Messages'][0]['Body']) print(message)
Parameters
QueueUrl (string) - The URL of the Amazon SQS queue from which messages are received.
AttributeNames (list) - A list of attributes that need to be returned along with each message. These attributes include: All – Returns all values.
MaxNumberOfMessages (integer) - The maximum number of messages to return. Amazon SQS never returns more messages than this value (however, fewer messages might be returned). Valid values: 1 to 10. Default: 1.
VisibilityTimeout (integer) - [optional] The duration (in seconds) that the received messages are hidden from subsequent retrieve requests after being retrieved by a ReceiveMessage request.
WaitTimeSeconds (integer) - [optional] The duration (in seconds) for which the call waits for a message to arrive in the queue before returning.
Delete Messages
Messages can be deleted after receiving and processing the message. Delete the message by passing the message’s receipt handle and queue URL to the AmazonSQS client’s delete_Message method. This code will first check the number of messages in queue. If the message is received in a queue then that message will be deleted. If the number of messages received in a queue is 0 (means queue is empty) then it will break the code.
import boto3 import json def lambda_handler(event, context): sqs = boto3.client('sqs') while True: QueueUrl='****' response = sqs.receive_message( QueueUrl= QueueUrl, AttributeNames=['ALL'], MaxNumberOfMessages=10) print(f"Number of messages received: {len(response.get('Messages', []))}") if len(response.get('Messages', [])) == 0: break for msg in response['Messages']: message=json.loads(response['Messages'][0]['Body']) print('message', message) delete_message= sqs.delete_message(QueueUrl=QueueUrl, ReceiptHandle=msg["ReceiptHandle"]) print('delete_message', delete_message)
Parameters
QueueUrl (string) - The URL of the Amazon SQS queue from which messages are deleted. Queue URLs and names are case-sensitive.
ReceiptHandle (string) - The receipt handle associated with the message to delete.
Conclusion
Lambda function is used to send received and delete messages from sqs. The code uses the Boto3 to send and receive messages by using AWS.SQS client class: send_message, receive_message, delete_message.