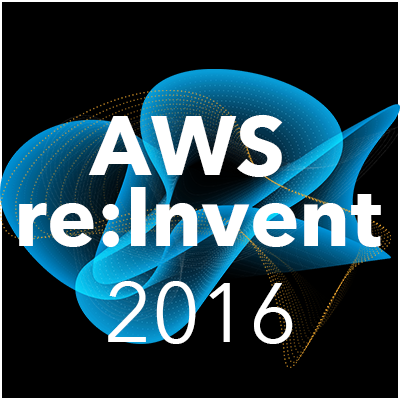
AWS Health APIを AWS SDK For Pythonから使ってみる #reinvent
Amazon 管理コンソールのパーソナルヘルスダッシュボードで表示されている AWS Health Information は AWS Health API 経由でも取得可能になりました。
今回は AWS SDK For Python からこの API を使う方法を紹介します。
AWS SDK For Java から使う方法は次の公式ドキュメントを参照して下さい。
Sample Java Code for the AWS Health API - AWS Health
AWS Health API の注意事項
最初に AWS Health API の注意事項を説明します。 SDK の言語、AWS CLI に関係なく影響を受けます。
エンドポイントは us-east-1 固定
エンドポイントは us-east-1、つまり、US East (N. Virginia)固定です。 このエンドポイントですべてのリージョンのイベントデータを提供しています。
通常は 日本リージョン(ap-northeast-1) を使っている場合も、Health API を呼び出すときだけは us-east-1 に向けてリクエストを投げて下さい。
試しに日本リージョンに向けて API を叩くと、次のようにエンドポイントに関するエラーが発生します。
$ aws health describe-events \ --region ap-northeast-1 Could not connect to the endpoint URL: "https://health.ap-northeast-1.amazonaws.com/"
ビジネス、または、エンタープライズサポートに加入したアカウントでのみ利用可能
ビジネスにもエンタープライズにも加入していないアカウントに対して API を叩くと、次のように SubscriptionRequiredException
エラーが発生します。
$ aws health describe-events \ --profile test \ --region us-east-1 An error occurred (SubscriptionRequiredException) when calling the DescribeEvents operation:
ロケールについて
- DescribeEventDetails API
- DescribeAffectedEntities API
ではロケールを指定する事ができます。
現時点ではデフォルトでもある英語(ロケールとしては en-EN
, en-US
, en-UK
など)にしか対応していないため、ロケールを指定することに意味はありません。
試しに日本語の ja-JP
や英語の de-DE
を指定すると、次のようにロケールに関するエラーが発生します。
$ aws health describe-event-details \ --event-arns "arn:aws:health:us-west-2::event/AWS_EC2_OPERATIONAL_ISSUE_1234567890" \ --locale ja-JP An error occurred (UnsupportedLocale) when calling the DescribeEventDetails operation: Locale ja-JP is not supported
対応ロケールが不明瞭な件について、ドキュメントページからフィードバックすると、次のコメントをいただきました。(2016/12/06)
We do plan to provide support for Japanese as well, but I am not certain of the timeline for completing that.
AWS Health API の使い方
以下では AWS Health API の使い方を紹介します。
API の種類
API 一覧を紹介します。現時点では6種類の API が存在します。
AWSのリソースに影響を与えるイベントについての情報を取得するために、以下のAPI を使います
- DescribeEvents:イベントに関する要約情報。
- DescribeEventDetails:1つ以上のイベントについての詳細情報。
- DescribeAffectedEntities:1つ以上のイベントの影響を受けているAWSリソースに関する情報。
イベントのタイプおよびイベントのサマリーカウントまたは影響を受けたエンティティに関する情報を取得するために、以下の API を使います
- DescribeEventTypes:AWS Health が追跡するイベントの種類に関する情報。
- DescribeEventAggregates:指定された基準を満たすイベント数のカウント。
- DescribeEntityAggregates:指定された基準を満たす影響を受けたエンティティ数のカウント。
AWS Health API の 初期化
エンドポイントが N.バージニア(us-east-1) となるように初期化します。
import boto3 health = boto3.client('health', region_name='us-east-1')
DescribeEvents:イベントに関する要約情報
for event in health.describe_events()['events']: print event
DescribeEventDetails:1つ以上のイベントについての詳細情報
event_arns = [event['arn'] for event in health.describe_events()['events']] for event_detail in health.describe_event_details(eventArns = event_arns, locale='en-US')['successfulSet']: print event_detail
eventArns にイベントの ARN をリストまたはタプルで渡します。 locale はデフォルトでは英語です。
DescribeAffectedEntities:1つ以上のイベントの影響を受けているAWSリソースに関する情報
event_arns = [event['arn'] for event in health.describe_events()['events']] for event_detail in health.describe_affected_entities(**{'filter':{'eventArns' : event_arns}, 'locale':'en-US'})['entities']: print event_detail
eventArns にイベントの ARN をリストまたはタプルで渡します。
locale はデフォルトでは英語です。
describe_affected_entities
メソッドへの引数の渡し方で注意が必要です。
health.describe_affected_entities(filter = {'eventArns':...})
のように書きたいところですが、filter
は Python の予約語のためシンタックスエラーになります。
紹介したサンプルコードのように、引数を {'filter':{'eventArns':...}}
と辞書にし、その辞書をメソッドに渡して回避します。
DescribeEventTypes:AWS Health が追跡するイベントの種類に関する情報
next_token = None while True: if next_token: result = health.describe_event_types(nextToken = next_token) else: result = health.describe_event_types() for event_type in result['eventTypes']: print event_type try: next_token = result['nextToken'] except: break
nextToken
をつかったページネーションが必要です。
DescribeEventAggregates:指定された基準を満たすイベント数のカウント
for aggregate in health.describe_event_aggregates(aggregateField='eventTypeCategory')['eventAggregates']: print aggregate
aggregateField
の指定が必要です。現時点では eventTypeCategory
だけに対応しています。
DescribeEntityAggregates:指定された基準を満たす影響を受けたエンティティ数のカウント
event_arns = [event['arn'] for event in health.describe_events()['events']] for aggregate in health.describe_entity_aggregates(eventArns = event_arns)['entityAggregates']: print aggregate
eventArns にイベントの ARN をリストまたはタプルで渡します。
まとめ
今回は AWS SDK For Python から AWS Health API を呼び出す方法を紹介しました。
AWS Health API のエンドポイントは N. Virginia リージョン固定です。
Lambda などで一つのアプリケーションから AWS Health をふくめた複数の AWS サービスを利用する場合は、サービスごとに正しいエンドポイントに対してリクエストするように気をつけて下さい。