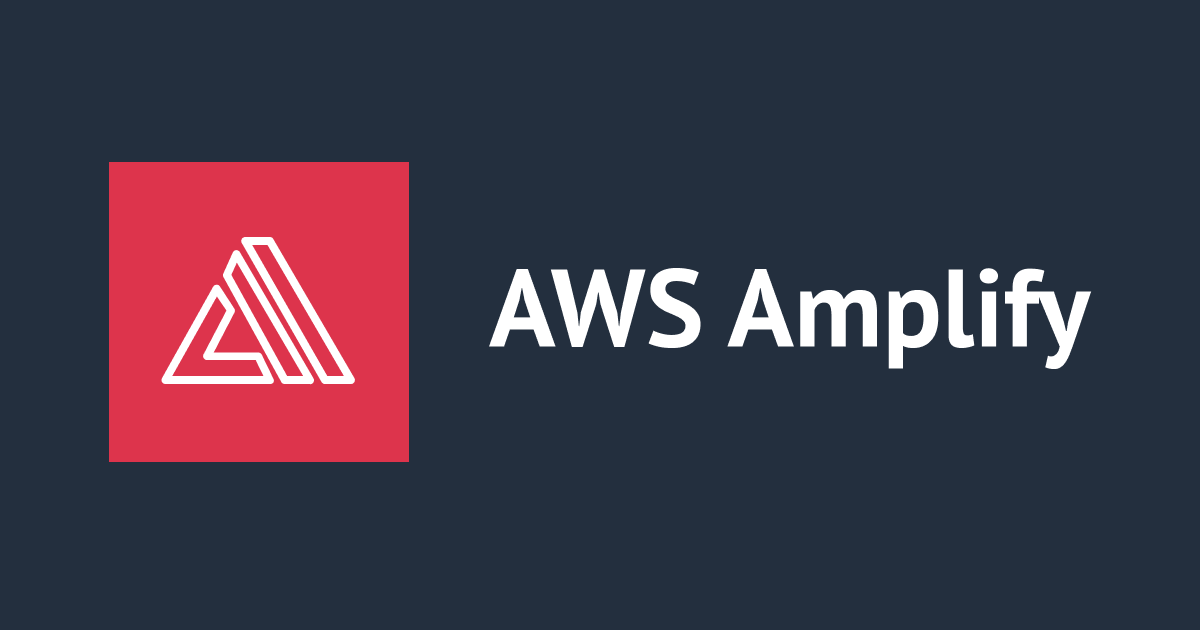
React에서 Amplify UI 기본 회원가입 폼 커스텀하기
이번에 Amplify UI를 이용해 기본 회원가입 폼을 커스텀 하는 방법에 대해서 간단히 알아보았습니다.
준비
먼저 React 프로젝트를 생성 후 필요한 라이브러리를 설치합니다.
npm create vite@latest [project-name] -- --template react-ts npm install @aws-amplify/ui-react aws-amplify
초기 세팅
초기 세팅입니다. App.tsx 에 아래의 코드를 적용하는 것으로 끝입니다.
import "@aws-amplify/ui-react/styles.css"; import { Amplify } from "aws-amplify"; import { Authenticator } from "@aws-amplify/ui-react"; Amplify.configure({ // ... }); function App() { return ( <Authenticator> {({ signOut, user }) => ( ... )} </Authenticator> ); } export default App;
단, 회원 가입 기능에는 Amazon cognito의 생성이 필요합니다. 생성 후 아래의 지정을 통해 실제 회원가입의 기능을 확인해 볼 수 있습니다.
이번에는 Amazon cognito의 설정에 관해서는 빠르게 스킵하고 다음으로 UI의 커스텀에 대해서 알아보겠습니다.
Amplify.configure({ aws_project_region: your product region, aws_cognito_region: your cognito region, aws_user_pools_id: your cognito user pools id, aws_user_pools_web_client_id: your cognito user pools web client id, });
상세히 알고 싶으신 분들은 아래의 링크로 부터 설정 방법을 찾아보시는 것도 좋을 것 같습니다.
회원가입 폼 커스텀
처음으로는 외부 UI의 커스텀 방법입니다. 이 경우에는 "components" 옵션을 통해 아래와 같이 사용할 component를 지정하기만 하면 끝입니다.
단, 회원가입이나 로그인 폼 등 해당 전용 페이지에만 적용하고 싶은 경우에는 아래의 코드와 같이 "SignUp" 을 별도 지정할 필요가 있습니다.
... import { Authenticator, Text } from "@aws-amplify/ui-react"; Amplify.configure({ // ... }); function DefaultHeader() { return <Text>DefaultHeader</Text>; } function SignUpHeader() { return <Text>SignUpHeader</Text>; } function DefaultFooter() { return <Text>DefaultFooter</Text>; } function SignUpFooter() { return <Text>SignUpFooter</Text>; } const amplifyUiCustomComponents = { Header() { return <DefaultHeader />; }, Footer() { return <DefaultFooter />; }, SignUp: { Header() { return <SignUpHeader />; }, Footer() { return <SignUpFooter />; }, }, }; function App() { return ( <Authenticator components={amplifyUiCustomComponents}> {({ signOut, user }) => ( ... )} </Authenticator> ); } ...
다음으로 만약 내부 UI의 커스텀을 하고 싶다면, "formFields" 옵션을 이용하면 편리합니다.
"isRquired" 옵션 등을 이용하면 기본적인 validation 지정도 가능합니다.
... const amplifyCustomFields = { signUp: { "custom:customField": { label: "Custom field", placeholder: "custom field placeholder", isRequired: true, }, }, }; function App() { return ( <Authenticator components={amplifyCustomComponents} formFields={amplifyCustomFields} > ... </Authenticator> ); } ...
마지막으로, 추가한 필드의 입력데이터를 실제로 확인해 보겠습니다.
이용할 옵션은 "services" 입니다.
아래와 같이 지정 후 "attributes"의 내부를 확인하면 실제로 입력한 값을 확인할 수 있습니다.
... function App() { const amplifyCustomServices = { async handleSignUp(formData) { const { ..., attributes } = formData ... }, }; return ( <Authenticator components={amplifyCustomComponents} formFields={amplifyCustomFields} services={amplifyCustomServices} > ... </Authenticator> ); } ...
이상으로 기본 회원가입 폼의 UI 커스텀에 대해서 알아보았습니다.
참고로 생략된 코드의 구현 방법이 궁금하시다면 이하의 링크를 참고하시면 좋을 것 같습니다.
Amplify UI for react - Customization Override Function Calls
아쉬운점
Amplify UI에는 다양한 폼이 제공되며 폼에 따라 일부 제약이 있을 수 있습니다.
예를 들면 로그인 폼의 경우 마지막 입력데이터의 확인이 어려울 수 있습니다.
실제 문서를 확인해 보면 회원가입 폼과 달리 로그인 폼의 경우에는 "username, password" 만 "formData"에서 제공하는 듯합니다.