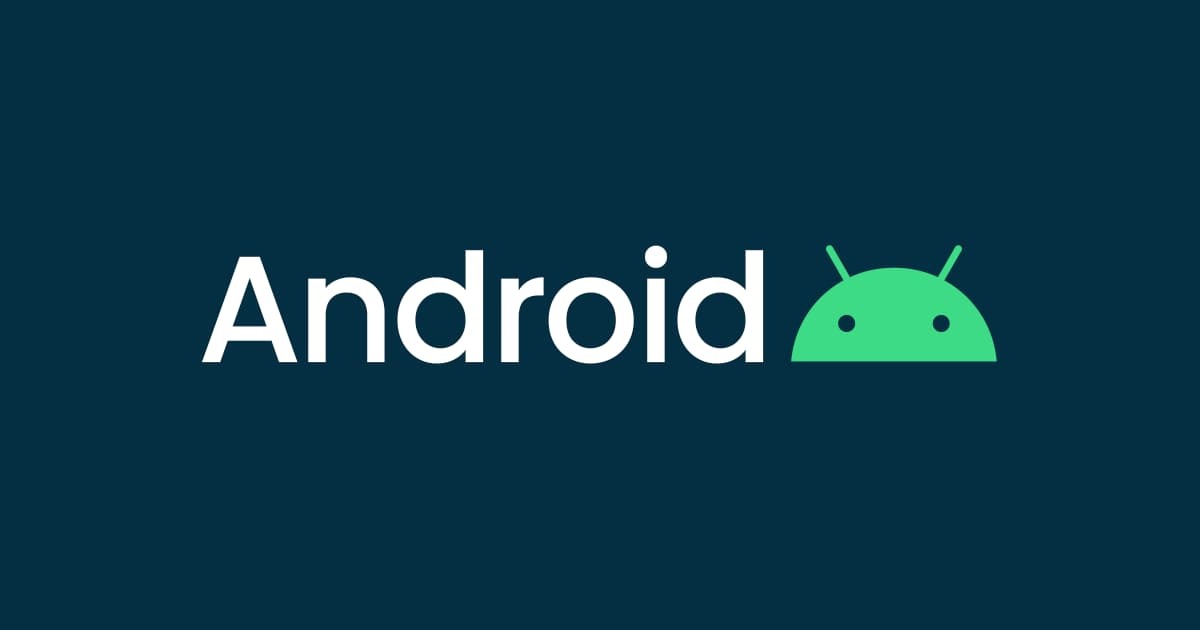
Androidアプリ開発(Kotlin)に入門してみた
こんにちは、ゲームソリューション部のsoraです。
今回は、Androidアプリ開発(Kotlin)に入門してみたことについて書いていきます。
私はFlutterを少し使用していることもあり、Android Studioのインストールなどは今回割愛します。
以下にWindowsでFlutterの環境構築をした記事があるので、必要であればご参照ください
生成されるディレクトリについて
プロジェクトを作成すると生成されるディレクトリ・ファイルは以下です。
それぞれどのようなディレクト・ファイルなのかは、コメントに記載しています。
特に見た目部分を記述するactivity_main.xml
、処理を記述するMainActivity.kt
、アプリの設定情報を定義するAndroidManifest.xml
あたりが主に触っていくファイルかなと思いました。
. ├── app # アプリのビルド設定を定義、依存関係/ビルドタスク/プラグインなどを設定 │ ├── build.gradle.kts # 外部ライブラリを格納するディレクトリ │ ├── libs # アプリのコード最適化に使用されるProguardのルールを定義 │ ├── proguard-rules.pro # Proguard:Androidアプリのバイトコードを最適化するツール │ └── src # Android端末を使ったエミュレータ上で行うテストのためのディレクトリ │ ├── androidTest │ │ └── java │ │ └── com │ │ └── example │ │ └── kotlin_firsttest │ │ └── ExampleInstrumentedTest.kt # アプリの主要なソースコード/ファイルを格納するディレクトリ │ ├── main # アプリの設定情報を定義 │ │ ├── AndroidManifest.xml # ソースコードを格納するディレクトリ(使用言語がKotlinでもjavaディレクトリになる) │ │ ├── java │ │ │ └── com │ │ │ └── example │ │ │ └── kotlin_firsttest │ │ │ └── MainActivity.kt # アプリの見た目部分のリソース(画像、レイアウト、文字列など)を格納するディレクトリ │ │ └── res # アプリで使用する画像ファイル(PNG、JPEG、SVGなど)を格納するディレクトリ │ │ ├── drawable │ │ │ ├── ic_launcher_background.xml │ │ │ └── ic_launcher_foreground.xml # ★アプリの画面レイアウトを定義するXMLファイル │ │ ├── layout │ │ │ └── activity_main.xml # アプリのアイコンファイルを解像度ごとに格納するディレクトリ │ │ ├── mipmap-... │ │ │ ├── ic_launcher.xml │ │ │ └── ic_launcher_round.xml # アプリで使用する文字列、色、スタイルなどの定義 │ │ ├── values # 色の定義、例えばblackは文字コードの何を指すかなど │ │ │ ├── colors.xml # 文字列の定義、アプリの名前などの定義、アプリ内で使用する固定の文字列もここに記述 │ │ │ ├── strings.xml │ │ │ └── themes.xml #文字のスタイルなどのテーマの定義 │ │ ├── values-night #ダークモード対応の定義 │ │ │ └── themes.xml │ │ └── xml #アプリの設定ファイルやリソース定義ファイルを格納するディレクトリ │ │ ├── backup_rules.xml │ │ └── data_extraction_rules.xml │ └── test #ユニットテスト │ └── java │ └── com │ └── example │ └── kotlin_firsttest │ └── ExampleUnitTest.kt ├── build.gradle.kts #プロジェクト全体のビルド設定を定義 ├── gradle │ └── wrapper #Gradleラッパーを格納するディレクトリ │ ├── gradle-wrapper.jar │ └── gradle-wrapper.properties ├── gradle.properties #Gradleの設定プロパティを定義 ├── gradlew #Unixベースのシステム用のGradleラッパースクリプト ├── gradlew.bat #Windowsシステム用のGradleラッパースクリプト ├── local.properties #ローカル開発環境の設定(Android SDKの場所など)を定義するファイル └── settings.gradle.kts #マルチモジュールプロジェクトの設定を定義するファイル
UI(activity_main.xml)
まずUI部分のactivity_main.xml
を見ていきます。
Android Studioでファイルを開くと、GUIのみでの操作・GUIとコードでの操作・コードのみでの操作の3つのモードにより編集が可能です。
GUIで単純に要素を配置してもlayout周りで警告が出て、実行は可能なものの意図していない場所に表示されたため、結局私はコードで記述しました。
GUIでもlayoutの詳細設定もできると思うので、そのあたりを細かく指定する必要がありそうです。
以下は初期コードのテキストの下にボタンを追加したコードです。
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/text" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView" /> </androidx.constraintlayout.widget.ConstraintLayout>
処理部分(MainActivity.kt)
処理を記述する部分はKotlinで記述します。
今回は先ほど配置したボタンを押すことで、表示されるテキストが変わる処理を入れています。
コードの解説はコメントで記述しています。
package com.example.kotlin_firsttest import android.annotation.SuppressLint import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import android.widget.Button import android.widget.TextView // AppCompatActivityを継承したMainActivityクラスを定義 class MainActivity : AppCompatActivity() { @SuppressLint("SetTextI18n") // onCreateはActivityが作成されたときに呼び出されるメソッド // savedInstanceStateには、前回の情報が保存されている override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) // activity_main.xmlファイルをActivityの表示内容に設定 setContentView(R.layout.activity_main) // findViewByIdでレイアウトファイルに定義したTextViewとButtonのViewを取得 // R.id.textViewとR.id.buttonは、ViewIDを表している val textView = findViewById<TextView>(R.id.textView) val button = findViewById<Button>(R.id.button) // ボタンがクリックされたときに実行 // textView内のtextを変更する button.setOnClickListener { if (textView.text == "Hello World!") { textView.text = "Button clicked!" } else { textView.text = "Hello World!" } } } }
アプリの設定情報の定義(AndroidManifest.xml)
アプリの設定情報の定義をするファイルです。
Flutterで開発していても触る機会が出やすいファイルかなと思います。
(個人的に理解が足りていなくて、もっと理解しなければならないと感じています。)
manifest
タグについて
・xmlns:android
:Android SDKが提供する属性を使用できるようにする宣言
・xmlns:tools
:ツールが提供する属性を使用できるようにする宣言
application
タグにて、アプリのアイコンやアプリ名を指定しています。@mipmap/…
などはパスを表しています。
activity
タグにて、アプリケーションのメインアクティビティを定義しています。
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <application android:allowBackup="true" android:dataExtractionRules="@xml/data_extraction_rules" android:fullBackupContent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.Kotlin_firsttest" tools:targetApi="31"> <activity android:name=".MainActivity" android:exported="true"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
実行
一通りコードを見ていって追加修正したため動かしてみます。
Android StudioだとRunボタンから実行することが可能です。
実行時、以下2点が思ったことです。
・Flutterみたいなホットリロードではない(設定を修正すればできるかもしれない)
・エミュレータを起動していなくても、Runボタンを押すと自動的にエミュレータが立ち上がって実行される
⇒Flutterの場合、エミュレータが存在しない旨のメッセージが出る
最後に
今回は、Androidアプリ開発(Kotlin)に入門してみたことを記事にしました。
どなたかの参考になると幸いです。