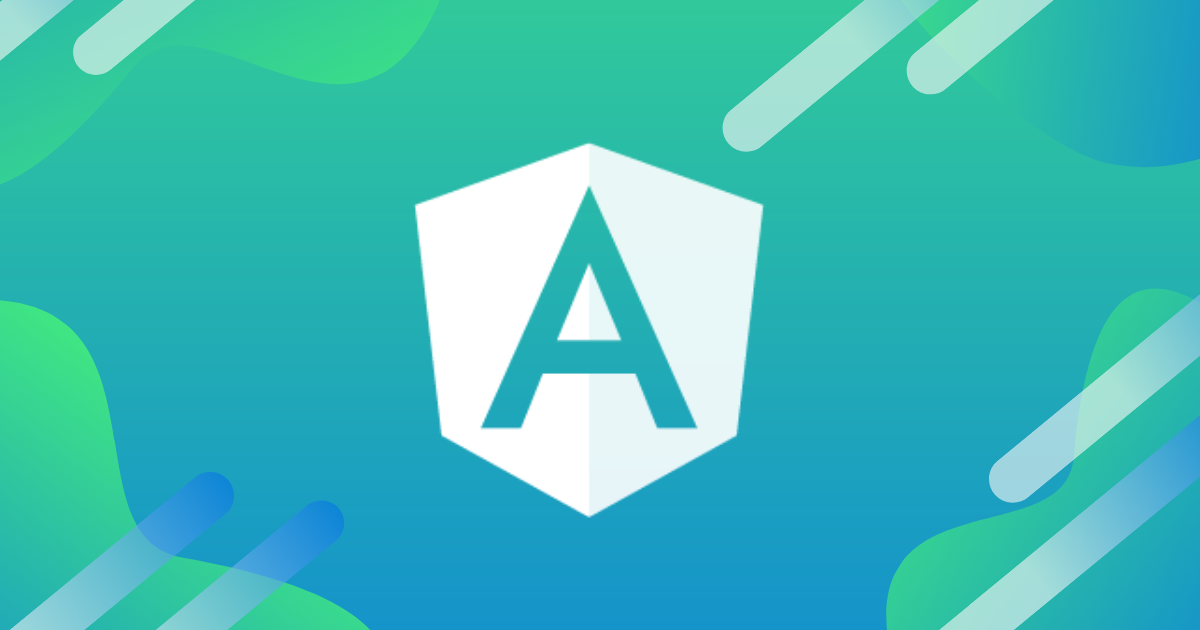
Angular10にESLintとPrettierを追加する #Angular #Angular10
2020/07/07現在の最新バージョン、Angular10ではTSLintが適用されています。
ですが、TSLintのロードマップにもある通り、既にTSLintは非推奨になっていて2020年12月1日に完全廃止することが宣言されています。
よってTSLintからESLintへの移行が推奨されています。
ここでは、Angular10のプロジェクトにESLintとPrettierを追加する手順を紹介します。
環境
- Angular: 10.0.2
- Angular CLI: 10.0.1
- eslint: 6.8.0
- prettier: 2.0.5
ESLint
TSLintの削除
まずはデフォルトでインストールされているTSLintを削除します。
$ rm tslint.json $ npm uninstall tslint
angular-eslint関連パッケージのインストール
以下のコマンドで関連するパッケージが一括でインストールされます。
$ ng add @angular-eslint/schematics
ESLintの設定ファイルを追加
$ touch .eslintrc.js
module.exports = { extends: ['plugin:@angular-eslint/recommended'], rules: { '@angular-eslint/directive-selector': [ 'error', { type: 'attribute', prefix: 'app', style: 'camelCase' }, ], '@angular-eslint/component-selector': [ 'error', { type: 'element', prefix: 'app', style: 'kebab-case' } ] }, overrides: [ { files: ['*.ts'], parser: '@typescript-eslint/parser', parserOptions: { ecmaVersion: 2020, sourceType: 'module', project: ['*/tsconfig.json', './tsconfig.**.json'] // 追加 }, plugins: ['@angular-eslint/template'], processor: '@angular-eslint/template/extract-inline-html' }, ], };
上記は@angular-eslintのサンプルです。
ただ、サンプルではAngular9のプロジェクトとなっているので、Angular10に合わせて少し修正します。
Angular10では、tsconfig.jsonが下記のように分割されています。
- tsconfig.json
- tsconfig.base.json
- tsconfig.app.json
- tsconfig.spec.json
- e2e/tsconfig.json
これを全て反映させるためにparserOptions.project
に以下を追加します。
['*/tsconfig.json', './tsconfig.**.json']
ちなみにESLintの設定ファイルはJSON|YAML|JSで記述できます。
ng lintの設定変更
angular.jsonのLintの項目を修正します。
"lint": { "builder": "@angular-eslint/builder:lint", "options": { "eslintConfig": ".eslintrc.js", // 追加 "tsConfig": [ "tsconfig.app.json", "tsconfig.spec.json", "e2e/tsconfig.json" ], "exclude": [ "**/node_modules/**" ] } }
確認
ESLintが正しく適用できているか確認します。
$ ng lint Linting "sample-app"... /Users/oka.haruna/playground/sample-app/src/app/app.component.html 12:1 error This line has a length of 159. Maximum allowed is 140 max-len // 中略 519:1 error This line has a length of 1046. Maximum allowed is 140 max-len ✖ 41 problems (41 errors, 0 warnings) /Users/oka.haruna/playground/sample-app/src/app/app.component.spec.ts 23:6 error Strings must use singlequote quotes ✖ 1 problem (1 error, 0 warnings) 1 error and 0 warnings potentially fixable with the `--fix` option. Lint errors found in the listed files.
初期構成のままだと app.component.spec.ts
と app.component.html
でエラーが出ました。
とりあえずエラーを消すため、fixオプションを実行します。
$ ng lint --fix
app.component.htmlの方は自動修正されないのでサンプルコード部分は一旦削除します。(routerを有効にしてる場合はその部分だけ残す)
<router-outlet></router-outlet>
$ ng lint Linting "sample-app"... All files pass linting.
これでLint自体の追加は完了です。 ルールはカスタマイズしてください。
Prettier
Prettierのインストール
$ npm i -D prettier \ eslint-config-prettier \ eslint-plugin-prettier
Prettierの設定ファイルを追加
Prettierの設定ファイルもJSON|YAML|JS|TOML、もしくはpackage.jsonに内包する形で記述できます。
ここでは記述量が比較して少ないYAMLにします。
$ touch .prettierrc.yml
以下はデフォルトのルールになります。
trailingComma: "es5" tabWidth: 4 semi: false singleQuote: true
こちらのドキュメントに従ってルールを変更してください。
VSCodeの設定(オプション)
VSCodeの拡張機能を追加することで、ファイル保存時に自動でフォーマットすることができます。
Prettierの拡張機能を追加して、.vscode/settings.json
に以下を追加してください。
"editor.formatOnSave": true