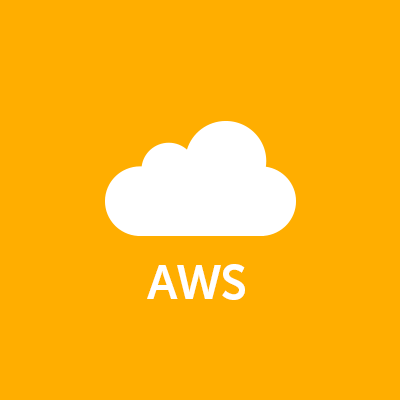
Get specific information from AWS Personal Health Dashboard using AWS SDK For Python (Boto 3)
AWS Personal Health Dashboard (AWS Health) displays event information affecting your AWS account. You can also use Amazon CloudWatch Events to detect event status changesand perform actions.
This time, I will demonstrate you how to acquire AWS Health information using Python (Boto 3).
Note
- The AWS Health API is available for business support plan and enterprise support plan accounts.
- Region used is
us-east-1
.
Sample Code
import boto3 import json from bson import json_util # Health constant REGION = 'us-east-1' # Function for describe_event (Specify desired information and acquire event.) def get_describe_event(services, event_status_codes): health = boto3.client('health', region_name=REGION) response = health.describe_events( filter={ 'services': services, 'eventStatusCodes': event_status_codes } ) return response # Function for describe_event_details (Detailed information about the specified event.) def get_describe_event_details(event_arns): health = boto3.client('health', region_name=REGION) response = health.describe_event_details(eventArns=event_arns) return response # Function for describe_affected_entities (Returns the list of entities affected by the specified event.) def get_describe_affected_entities(event_arns): health = boto3.client('health', region_name=REGION) response = health.describe_affected_entities( filter={ 'eventArns': event_arns } ) return response def main(): # Filter by specific information for describe_event function. # (ex. services is vpn, event status coeds except close status.) services = ['VPN'] event_status_codes = ['open', 'upcoming'] # Get describe event (Specify desired information as list type) result = get_describe_event(services, event_status_codes) print("starting describe event") print(json.dumps(result, default=json_util.default, sort_keys=True, indent=4)) print("end of describe event") # Get describe event details event_arns = [] for event in result['events']: event_arns.append(event['arn']) result = get_describe_event_details(event_arns) print("starting describe event details") print(json.dumps(result, default=json_util.default, sort_keys=True, indent=4)) print("end of describe event details") # Get describe affected entities result = get_describe_affected_entities(event_arns) print("starting describe affected entities") print(json.dumps(result, default=json_util.default, sort_keys=True, indent=4)) print("end of describe affected entities") if __name__ == '__main__': main()
DescribeEvents
Get events using DescribeEvents. In the sample code, we get events other than closed by setting Service
to VPN, eventStatusCodes
open, upcoming.
If you want to narrow down the results a little more, you can filter by filter
, so please add it from the 13th line.
- Execution result
{ "ResponseMetadata": { "HTTPHeaders": { "content-length": "736", "content-type": "application/x-amz-json-1.1", "date": "Wed, 18 Apr 2018 06:58:38 GMT", "x-amzn-requestid": "eba3fbf0-42d5-11e8-b7d6-************" }, "HTTPStatusCode": 200, "RequestId": "eba3fbf0-42d5-11e8-b7d6-************", "RetryAttempts": 0 }, "events": [ { "arn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "endTime": { "$date": date }, "eventTypeCategory": "scheduledChange", "eventTypeCode": "AWS_VPN_MAINTENANCE_SCHEDULED", "lastUpdatedTime": { "$date": date }, "region": "region", "service": "VPN", "startTime": { "$date": date }, "statusCode": "upcoming" }, { "arn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "endTime": { "$date": date }, "eventTypeCategory": "scheduledChange", "eventTypeCode": "AWS_VPN_MAINTENANCE_SCHEDULED", "lastUpdatedTime": { "$date": date }, "region": "region", "service": "VPN", "startTime": { "$date": date }, "statusCode": "upcoming" } ] }
We noticed that there were two events related to VPN.
DescribeEventDetails
We got events with DescribeEvents, so the next step is to check the event details with DescribeEventDetails.
In DescribeEventDetails, since eventArns
is required, it is acquired with list
as follows.
# Get describe event details event_arns = [] for event in result['events']: event_arns.append(event['arn'])
- Execution result
{ "ResponseMetadata": { "HTTPHeaders": { "content-length": "2971", "content-type": "application/x-amz-json-1.1", "date": "Wed, 18 Apr 2018 07:00:36 GMT", "x-amzn-requestid": "3267386c-42d6-11e8-b7d6-************" }, "HTTPStatusCode": 200, "RequestId": "3267386c-42d6-11e8-b7d6-************", "RetryAttempts": 0 }, "failedSet": [], "successfulSet": [ { "event": { "arn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "endTime": { "$date": date }, "eventTypeCategory": "scheduledChange", "eventTypeCode": "AWS_VPN_MAINTENANCE_SCHEDULED", "lastUpdatedTime": { "$date": date }, "region": "region", "service": "VPN", "startTime": { "$date": date }, "statusCode": "upcoming" }, "eventDescription": { "latestDescription": "You're receiving this notification because you have at least one VPN Connection scheduled for planned maintenance in the region region. [...]" } }, { "event": { "arn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "endTime": { "$date": date }, "eventTypeCategory": "scheduledChange", "eventTypeCode": "AWS_VPN_MAINTENANCE_SCHEDULED", "lastUpdatedTime": { "$date": date }, "region": "region", "service": "VPN", "startTime": { "$date": date }, "statusCode": "upcoming" }, "eventDescription": { "latestDescription": "You're receiving this notification because you have at least one VPN Connection scheduled for planned maintenance in the region region. [...]" } } ] }
DescribeAffectedEntities
We got detailed information about the events, but we do not know the resources that are affected, so we can check for the resources with DescribeAffectedEntities.
DescribeAffectedEntities also needs eventArns
.
- Execution result
{ "ResponseMetadata": { "HTTPHeaders": { "content-length": "1046", "content-type": "application/x-amz-json-1.1", "date": "Wed, 18 Apr 2018 07:00:36 GMT", "x-amzn-requestid": "328065c2-42d6-11e8-b7d6-************" }, "HTTPStatusCode": 200, "RequestId": "328065c2-42d6-11e8-b7d6-************", "RetryAttempts": 0 }, "entities": [ { "awsAccountId": "************", "entityArn": "arn:aws:health:region:************:entity/AWLT6N8y************", "entityValue": "vpn-********", "eventArn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "lastUpdatedTime": { "$date": date }, "statusCode": "IMPAIRED" }, { "awsAccountId": "************", "entityArn": "arn:aws:health:region:************:entity/AWKV5Zj************", "entityValue": "vpn-********", "eventArn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "lastUpdatedTime": { "$date": date }, "statusCode": "IMPAIRED" }, { "awsAccountId": "************", "entityArn": "arn:aws:health:region:************:entity/AWKV5Zj************", "entityValue": "vpn-********", "eventArn": "arn:aws:health:region::event/AWS_VPN_MAINTENANCE_SCHEDULED_************", "lastUpdatedTime": { "$date": date }, "statusCode": "IMPAIRED" } ] }
We can confirm which resources were affected by looking at the entityValue
field.
Conclusion
This time AWS Health was used to acquire information related to VPN maintenance. If you want to acquire specific event information, we hope that you will be able to obtain the necessary information with the contents of the sample code we introduced, so it would be great if you could refer to it.