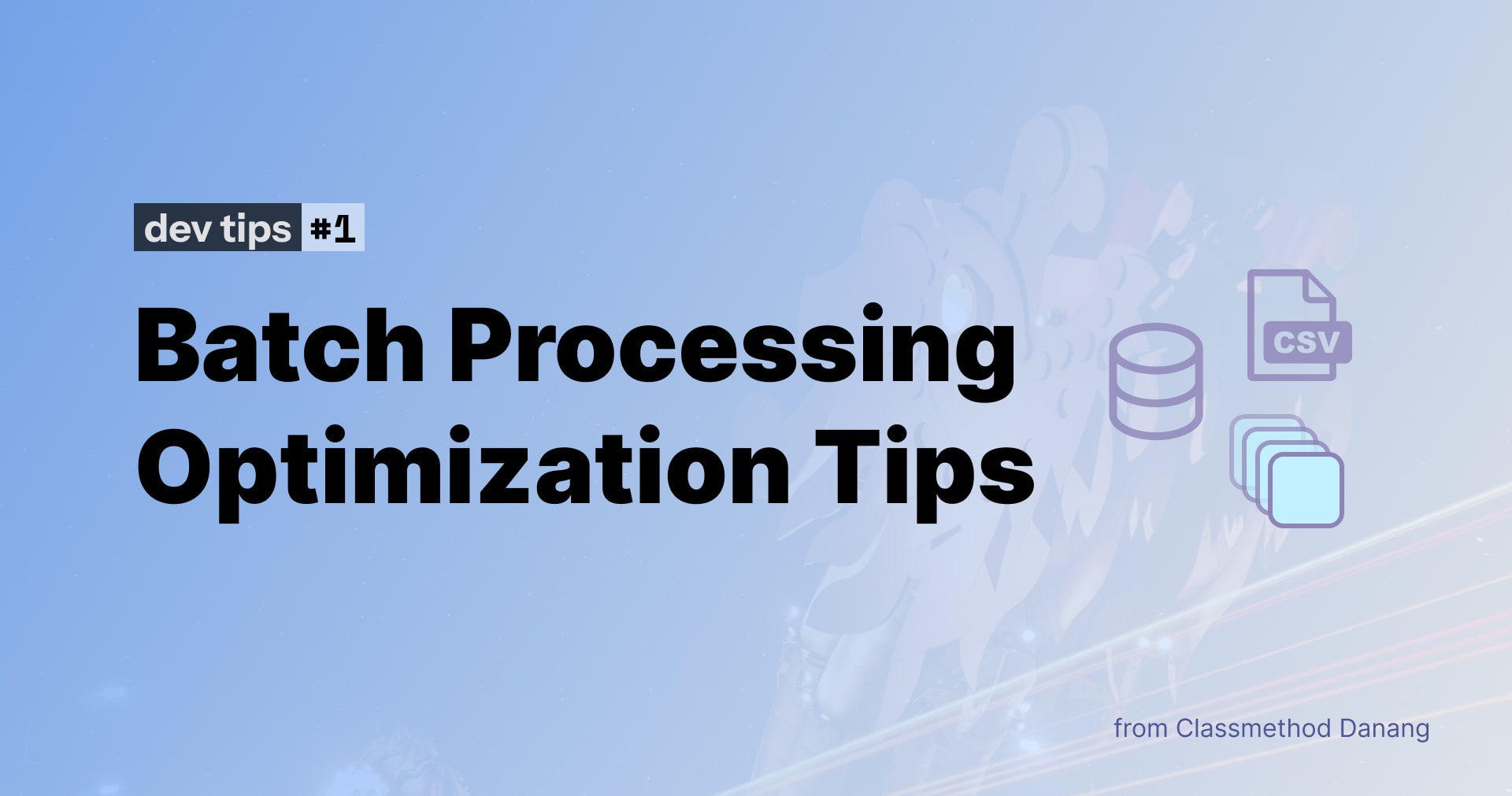
Batch Processing Optimization Tips
Overview
Batch processing is vital in backend systems for efficiently handling large datasets, such as importing CSV files, synchronizing data, or generating reports. Without proper optimization, it can lead to high memory usage, slow execution, or even system failures.
This article focuses on strategies and tips to optimize batch processing in both Laravel code and database queries using MySQL.
Whether you are handling millions of records in a relational database or processing files, these techniques will ensure your batch jobs run efficiently.
Code Optimization Strategies
1. Chunk Data
Processing large datasets at once can overwhelm your system’s memory. Laravel’s chunk()
or chunkById()
method provides an efficient way to process records in manageable pieces.
Bad:
$users = User::all(); // Loads all users into memory
foreach ($users as $user) {
// Process user
}
Good:
User::chunkById(1000, function ($users) {
foreach ($users as $user) {
// Process user
}
});
Explanation: The chunkById()
method fetches 1,000 records at a time, preventing memory exhaustion.
2. Reduce Loops
Nested loops can drastically slow down your batch processing.
Bad:
$users = User::all();
foreach ($posts as $post) {
// Get user by post
$user = $users->firstWhere([
'user_id' => $post->user_id
]);
}
Good:
$users = User::all()->keyBy('user_id');
foreach ($posts as $post) {
// Get user by post
$user = $users->get($post->user_id);
}
Explanation: Use key-value to search user_id
with O(1) complexity
3. Stream Files
If processing large files (e.g., CSVs), reading the entire file into memory can cause performance issues. Stream the file instead.
Bad:
$data = Storage::get('large-file.csv');
$lines = explode("\n", $data);
foreach ($lines as $line) {
// Process line...
}
Good:
$handle = Storage::readStream('large-file.csv');
while (($line = fgetcsv($handle)) !== false) {
// Process line
}
Explanation: Streaming reads the file line by line, conserving memory.
Query Optimization Strategies
1. Use Indexes
Indexes significantly improve query performance by reducing the number of rows scanned.
Bad:
-- No index on user_id
SELECT * FROM orders WHERE user_id = 1;
Good:
CREATE INDEX idx_user_id ON orders(user_id);
-- Utilizes the index
SELECT * FROM orders WHERE user_id = 1;
Explanation: Adding an index on user_id reduces query execution time, especially for large tables.
2. Avoid N+1 Queries
The N+1 problem occurs when fetching related data results in excessive queries.
Bad:
$users = User::all();
foreach ($users as $user) {
$posts = $user->posts; // Query for each user
}
Good:
$users = User::with('posts')->get();
foreach ($users as $user) {
$posts = $user->posts; // Loaded in a single query
}
Explanation: Using with()
retrieves related data in fewer queries.
3. Use Bulk Insert/Update
Updating or inserting records one by one is inefficient. Instead, use bulk operations.
Bad:
$handle = Storage::readStream('large-file.csv');
while (($data = fgetcsv($handle)) !== false) {
DB::table('users')->insert(data);
}
Good:
$handle = Storage::readStream('large-file.csv');
$usersData = [];
while (($data = fgetcsv($handle)) !== false) {
$usersData[] = $data;
if (count($usersData) == 1000) {
DB::table('users')->insert($usersData);
$usersData = [];
}
}
if (count($usersData)) {
DB::table('users')->insert($usersData);
$usersData = [];
}
Explanation: Insert every 1000 data users performs the operation in a single query, reducing overhead.
Conclusion
Optimizing batch processing in Laravel requires attention to both code and query design. By chunking data, reducing loops, and leveraging database features like indexing and bulk operations, you can dramatically improve performance. These strategies ensure your backend systems remain efficient, scalable, and cost-effective.