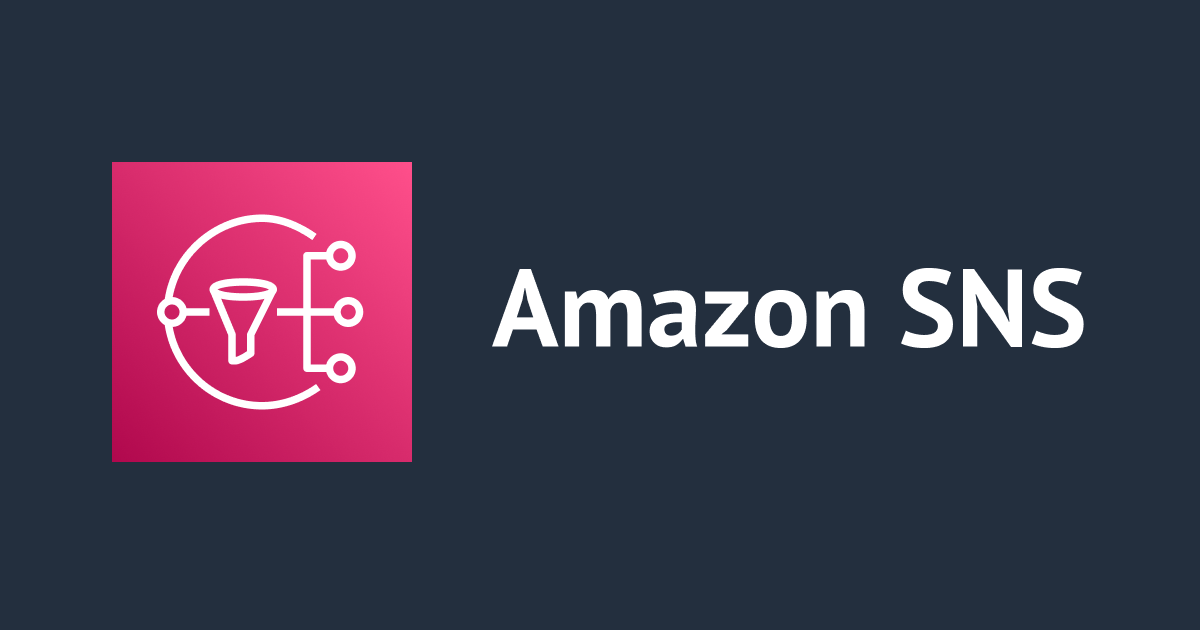
Create SNS topic and subscription using Python
Introduction
The Simple Notification Service (SNS) service provided by AWS can be used to provide Application to application(A2A) notification services and Application to person(A2P) notification services. The notifications can be sent to the subscribed customers using SMS texts, E-mail and push notifications. It is highly available, secure and durable.
Prerequisite
- Python
- AWS console and CLI
Create SNS Topic
import logging import boto3 from botocore.exceptions import ClientError logger = logging.getLogger() sns_client=boto3.client('sns') # creating topic
def create_topic(name): try: topic = sns_client.create_topic(Name=name) logger.info("Created topic %s with ARN %s.", name, topic) except ClientError: logger.exception("Couldn't create topic %s.", name) raise else: return topic
So, boto3 is basically used to manage AWS services. Here, we are using boto3 to manage SNS service. First we create an SNS client using boto3.client()
. Then we can create an SNS topic by using sns.create_topic()
by specifying the name and it returns a dictionary that contains the ARN of the created topic.
Create a subscription
# creating subscription
def subscribe(topic, protocol, endpoint): try: subscription = sns_client.subscribe( TopicArn=topic['TopicArn'], Protocol=protocol, Endpoint=endpoint, ReturnSubscriptionArn=True) logger.info("Subscribed %s %s to topic %s.", protocol, endpoint, topic) except ClientError: logger.exception( "Couldn't subscribe %s %s to topic %s.", protocol, endpoint, topic) raise else: return subscription
The above code is the function to create subscription of the topic. We need to specify the protocol we want to use for the subscription that is email
, here we would like to use email address as the endpoint.
When we call the sns.subscribe()
method to create the subscription, it returns a dictionary that contains the ARN of the subscription that was created.
topic_name = 'a-new-topic' print(f"Creating topic {topic_name}.") topicArn = create_topic(topic_name) subscribe(topicArn, "email", "example@xyz.com")
Then add these lines of code for creating the topic and subscription.
Results
When we run the code we get the following as the result.
When you open the AWS console, you can see that the topic with name a-new-topic
is created and a subscription is also created.
Check the mail inbox to confirm the subscription. Once you confirm the subscription, the status of the subscription will change from Pending confirmation
to Confirmed
.
Conclusion
This the basic example for creating an SNS topic and subscription. You can add your specifications and modifications such as different protocols to fit your requirements.
Here are some references:
Boto3: https://boto3.amazonaws.com/v1/documentation/api/latest/reference/services/sns.html
Getting-started with AWS SNS: https://aws.amazon.com/sns/getting-started/
Thank you!