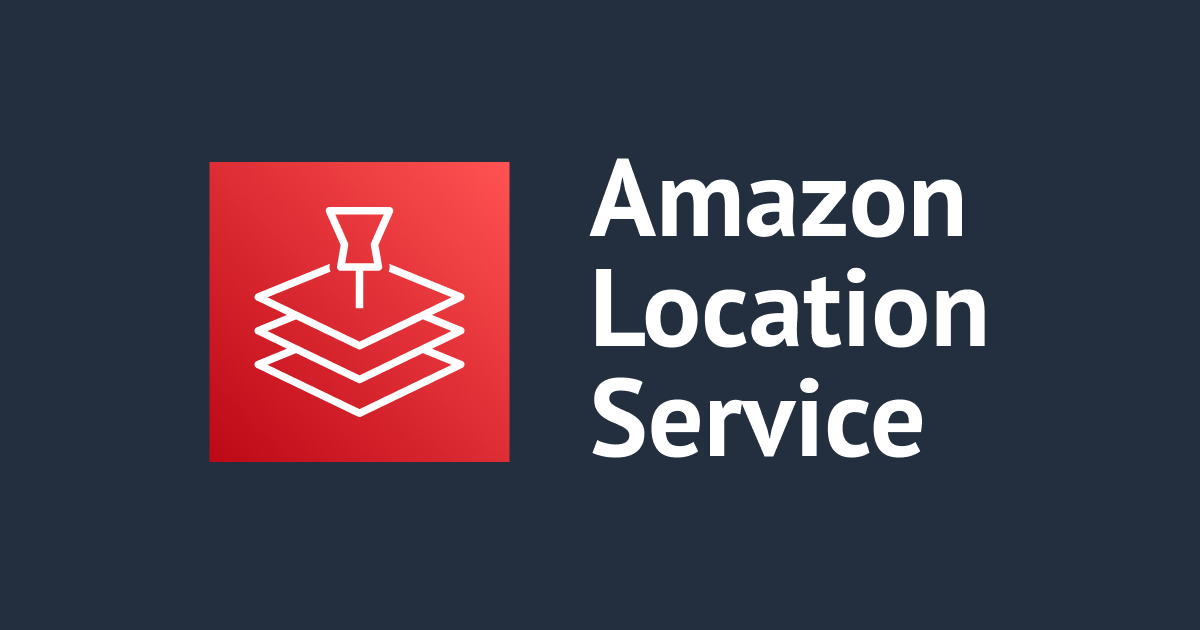
AWS CDKでAmazon Location Serviceの各種リソースを作成してみた
こんにちは、CX事業本部 IoT事業部の若槻です。
Amazon Location Serviceを利用すれば、デバイスや車両などの位置情報をトラッキングしたり、マップ上でデータを可視化したりすることができます。
Amazon Location Serviceでは各種リソースを作成して利用することによりこれらのユースケースを実現できるようになるのですが、せっかくなのでCloudFormationやCDKでリソースのプロビジョニングを自動化したいですね。
そこで今回は、AWS CDKでAmazon Location Serviceの各種リソースを作成してみました。
やってみた
現時点でAmazon Location ServiceのCDKモジュール(aws-cdk-lib.aws_location
)ではL2 Constructは未提供となります。
There are no official hand-written (L2) constructs for this service yet. Here are some suggestions on how to proceed:
よってL1 Construct(CfnXXX)を利用してリソースを作っていきます。
環境
$ npm ls aws-cdk aws-cdk-app@0.1.0 /Users/wakatsuki.ryuta/projects/cm-rwakatsuki/aws-cdk-app └── aws-cdk@2.50.0
モジュールのインポート
Stackで使えるようにモジュールをインポートします。
import { aws_location } from 'aws-cdk-lib'; //or import * as location from 'aws-cdk-lib/aws-location';
Map
Mapを作成する場合のCDKコードです。
new aws_location.CfnMap(this, 'myMap', { mapName: 'myMap', configuration: { style: 'VectorHereExplore' }, //必須 });
configuration.style
ではMapのスタイルを指定します。現在は下記の9種類が指定できます。(ソースコードから抜粋)先月のアップデートで追加された2つのスタイルはまだ使用できないようです。
VectorEsriDarkGrayCanvas
– The Esri Dark Gray Canvas map style. A vector basemap with a dark gray, neutral background with minimal colors, labels, and features that's designed to draw attention to your thematic content.RasterEsriImagery
– The Esri Imagery map style. A raster basemap that provides one meter or better satellite and aerial imagery in many parts of the world and lower resolution satellite imagery worldwide.VectorEsriLightGrayCanvas
– The Esri Light Gray Canvas map style, which provides a detailed vector basemap with a light gray, neutral background style with minimal colors, labels, and features that's designed to draw attention to your thematic content.VectorEsriTopographic
– The Esri Light map style, which provides a detailed vector basemap with a classic Esri map style.VectorEsriStreets
– The Esri World Streets map style, which provides a detailed vector basemap for the world symbolized with a classic Esri street map style. The vector tile layer is similar in content and style to the World Street Map raster map.VectorEsriNavigation
– The Esri World Navigation map style, which provides a detailed basemap for the world symbolized with a custom navigation map style that's designed for use during the day in mobile devices.VectorHereBerlin
– The HERE Berlin map style is a high contrast detailed base map of the world that blends 3D and 2D rendering.VectorHereExplore
– A default HERE map style containing a neutral, global map and its features including roads, buildings, landmarks, and water features. It also now includes a fully designed map of Japan.VectorHereExploreTruck
– A global map containing truck restrictions and attributes (e.g. width / height / HAZMAT) symbolized with highlighted segments and icons on top of HERE Explore to support use cases within transport and logistics.
CDK Deployすると、作成されたMapをコンソールで確認できました。
作成したMapのリソースは、アプリケーションへの埋め込みなどに使用できます。
PlaceIndex
PlaceIndexを作成する場合のCDKコードです。
new aws_location.CfnPlaceIndex(this, 'myPlaceIndex', { indexName: 'myPlaceIndex', dataSource: 'Here', //必須 });
dataSource
で、Data SourceとしてHere
またはEsri
のいずれかのMap Providerを指定します。
CDK Deployすると、作成されたPlaceIndexをコンソールで確認できました。
作成したPlaceIndexのリソースは、APIによるGeocoding、Reverse Geocodingに使用できます。
RouteCalculator
RouteCalculatorを作成する場合のCDKコードです。
new aws_location.CfnRouteCalculator(this, 'myRouteCalculator', { calculatorName: 'myRouteCalculator', dataSource: 'Here', //必須 });
dataSource
で、Data SourceとしてHere
またはEsri
のいずれかのMap Providerを指定します。
CDK Deployすると、作成されたPlaceIndexをコンソールで確認できました。
作成したRouteCalculatorのリソースは、APIによる経路計算に使用できます。
GeofenceCollection、Tracker
GeofenceCollectionを作成する場合のCDKコードです。(ハイライト部分)
const myGeofenceCollection = new aws_location.CfnGeofenceCollection( this, 'myGeofenceCollection', { collectionName: 'myGeofenceCollection', } ); const myTracker = new aws_location.CfnTracker(this, 'myTracker', { trackerName: 'myTracker', }); new aws_location.CfnTrackerConsumer(this, 'myTrackerConsumer', { trackerName: myTracker.trackerName, consumerArn: myGeofenceCollection.attrArn, });
CDK Deployすると、作成されたGeofenceCollectionをコンソールで確認できました。
このGeofenceCollectionにGeofenceを必要なタイミングで追加して、次節のTrackerを使用したデバイスや車両のジオフェンシングを行うことになります。
Tracker
Trackerを作成する場合のCDKコードです。(ハイライト部分)
const myGeofenceCollection = new aws_location.CfnGeofenceCollection( this, 'myGeofenceCollection', { collectionName: 'myGeofenceCollection', } ); const myTracker = new aws_location.CfnTracker(this, 'myTracker', { trackerName: 'myTracker', }); new aws_location.CfnTrackerConsumer(this, 'myTrackerConsumer', { trackerName: myTracker.trackerName, consumerArn: myGeofenceCollection.attrArn, });
CfnTracker
でTrackerを作成し、CfnTrackerConsumer
でGeofenceCollectionとリンクさせています。これによりGeofenceCollectionに含まれるGeofenceを利用したTrackerの追跡とイベント発生時のアクションの実行が可能になります。(前節の参考を参照)
CDK Deployすると、作成されたTrackerをコンソールで確認できました。
コード全体
import { aws_location, Stack, StackProps } from 'aws-cdk-lib'; import { Construct } from 'constructs'; export class AwsCdkAppStack extends Stack { constructor(scope: Construct, id: string, props?: StackProps) { super(scope, id, props); new aws_location.CfnMap(this, 'myMap', { mapName: 'myMap', configuration: { style: 'VectorHereExplore' }, //必須 }); new aws_location.CfnPlaceIndex(this, 'myPlaceIndex', { indexName: 'myPlaceIndex', dataSource: 'Here', //必須 }); new aws_location.CfnRouteCalculator(this, 'myRouteCalculator', { calculatorName: 'myRouteCalculator', dataSource: 'Here', //必須 }); const myGeofenceCollection = new aws_location.CfnGeofenceCollection( this, 'myGeofenceCollection', { collectionName: 'myGeofenceCollection', } ); const myTracker = new aws_location.CfnTracker(this, 'myTracker', { trackerName: 'myTracker', }); new aws_location.CfnTrackerConsumer(this, 'myTrackerConsumer', { trackerName: myTracker.trackerName, consumerArn: myGeofenceCollection.attrArn, }); } }
おわりに
AWS CDKでAmazon Location Serviceの各種リソースを作成してみました。
設定するプロパティなどはそんなに多くないですが、AWSリソースのプロビジョニングは極力IaCで管理したいケースはあると思うので、Amazon Location Serviceのリソースも例外とすることなくCDK管理と出来るのは嬉しいですね。
以上