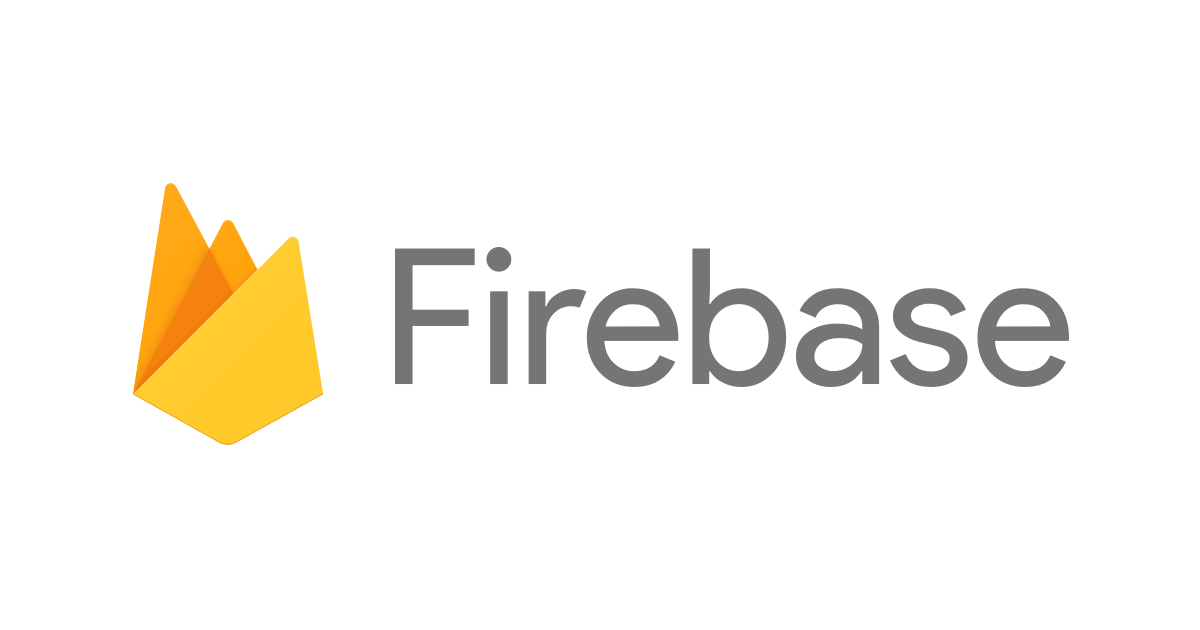
Firebase Integration and AWS Amplify hosting to my Sample Project
Introduction
As part of learning, I recently implemented a sample project which includes hands-on exposure to React, Typescript, Firebase Authentication, and Redux. The sample project has a simple login functionality with Google Sign-in. I have handled the Authentication using Firebase and maintained the state of the user using Redux(Redux-Toolkit). This blog specifically talks about Firebase Authentication and integration with the project and also about hosting procedures with AWS Amplify.
Prerequisites
Set up a React Typescript project. The below commands help us to set up a boilerplate code for React Typescript
npx create-react-app my-create-react-app typescript or yarn create-react-app my-app --template typescript
For the sample project the following were the version of tools used:
- Typescript 4.3.5 - React 17.0.2 - Redux 4.1.0 - Redux-Toolkit 1.6.0 - react-router-dom 5.2.0 - firebase 8.7.1 - react-google-button 0.7.2 - emotion 11.3.0
Firebase Setup
With a valid Google account create a Firebase account and click on add a “new project” in the Firebase console here. This step prompts you to add the project name. Secondly, it could ask us to enable or disable the Google Analytics for Firebase (it's not mandatory as it is an analytical tool for Firebase) and there you go, continue to launch your project.
Now you are redirected to the project overview page as shown below. Now as my sample project is a web application, I choose a web icon </>.
Now register your application with a nickname (it's just a convenient identifier visible only in the Firebase console) and you can also enable Firebase hosting now or later if you consider hosting your application on the Firebase. But I choose to host my application on AWS Amplify hence I skip this hosting part.
After registering the web app, we can proceed to add Firebase SDK to our React project. We can create an exclusive file named config.ts
under src
directory.
This config file includes Firebase imports and configuration contents, hence we should do the installation of the Firebase package.
npm install --save firebase or yarn add firebase
To complete the Firebase setup we should configure the Authentication method that one imbibes in the application. In my sample application, I'm using Google sign-in with popup feature hence I must enable the Google sign-in option in the Firebase console. For the following click on Authentication and get started.
Under the sign-in method click on the Google menu to enable it.
Once done, add a support email and click on save.
Here's how I added the Firebase SDK into my React project. I added Firebase config details to my index.tsx
file as shown below:
import React from 'react' import ReactDOM from 'react-dom' import App from './App' import firebase from 'firebase/app' const firebaseConfig = { apiKey: process.env.REACT_APP_FIREBASE_API_KEY, authDomain: process.env.REACT_APP_FIREBASE_AUTH_DOMAIN, projectId: process.env.REACT_APP_FIREBASE_PROJECT_ID, storageBucket: process.env.REACT_APP_FIREBASE_STORAGE_BUCKET, messagingSenderId: process.env.REACT_APP_FIREBASE_MESSAGING_SENDER_ID, appId: process.env.REACT_APP_FIREBASE_APP_ID, } // Initialize Firebase firebase.initializeApp(firebaseConfig) ReactDOM.render( <App />, document.getElementById('root') )
If you see in the firebaseConfig, all the key attributes are fetched from "process.env.REACT_APP_******". It's never a great idea to store important data like keys and credentials in the project. There are many ways to store them securely, and I choose to store my API keys in the .env files as shown below. I used the process.env property, which in turn returns an object containing the user environment variable. Generally .env file is not pushed to our repositories i.e. github or anywhere you store your code. This way our secrets are safe and are not exposed.
.env
REACT_APP_FIREBASE_API_KEY = "AIXXXXXXXXXXXXXXXX2192nL8" REACT_APP_FIREBASE_AUTH_DOMAIN = "XXXXXXXXXXX.firebaseapp.com" REACT_APP_FIREBASE_PROJECT_ID = "XXXXXXXXXX" REACT_APP_FIREBASE_STORAGE_BUCKET = "XXXXXXXXXX.appspot.com" REACT_APP_FIREBASE_MESSAGING_SENDER_ID = "345XXXXXXXXXXX9" REACT_APP_FIREBASE_APP_ID = "1:3450XXXXXXXX299:web:e5feXXXXXXXXXX7b39fe"
Implementation
My sample project is a very simple implementation of the React + Typescript with authentication handled by Firebase. Here's how I added the following to the sample project:
App.tsx
import React from 'react' import { Routes } from './Routes' const App: React.FunctionComponent = () => { return <Routes /> } export default App
Routes.tsx
import React, { VFC } from 'react' import { BrowserRouter as Router, Switch, Route } from 'react-router-dom' export const Routes: VFC = () => { return ( <Router> <Switch> <Route exact path="/" component={Login} /> </Switch> </Router> ) }
Login.tsx
import React, { VFC } from 'react' import styled from '@emotion/styled' import GoogleButton from 'react-google-button' import logoCxPassport from '../../../assets/logo_cx_passport.png' const Wrapper = styled.div` display: flex; align-items: center; justify-content: center; ` const Container = styled.div` display: block; align-items: center; justify-content: center; padding-top: 300px; padding-bottom: 30px; ` const LogoImage = styled.img` height: 40px; display: block; margin-right: auto; margin-left: auto; padding-bottom: 15px; ` export const Login: VFC = () =>{ LogoImage.defaultProps = { src: String(logoCxPassport), } return ( <Wrapper> <Container> <LogoImage /> <GoogleButton onClick={<function to handle onCLick of Google-Sign-in-with-Popup button>} /> </Container> </Wrapper> ) }
Hosting on AWS Amplify
From the AWS Console, under Services search for Amplify, select it and get started.
- For hosting, scroll down to Deliver option and get started.
-
We should connect our repository to proceed with deploying. Hence choose the location of your source code. My sample project is on GitHub.
-
Now that it is GitHub, for the first time, we should do GitHub Authorization. Then we can connect to the repository and branch of our source code.
-
Next, comes Configure Build settings, nothing to modify for right now, just check the box and click next to allow AWS Amplify to automatically deploy all files from your project root directory.
-
Next, review the Repository details and App settings. Provided everything is correct click on "save and deploy" and proceed.
-
Please wait for a couple of minutes until provision, build, deploy and verify are accomplished.
-
Now that our application has API Keys stored in .env files. We should setup Environment variables and build settings of AWS Amplify too.
a. In the App build specification edit the
amplify.yml
file as shown below and click on save.Amplify.yml
version: 1 frontend: phases: preBuild: commands: - yarn install build: commands: - yarn run build - echo "REACT_APP_FIREBASE_API_KEY=$REACT_APP_FIREBASE_API_KEY" >> .env - echo "REACT_APP_FIREBASE_AUTH_DOMAIN=$REACT_APP_FIREBASE_AUTH_DOMAIN" >> .env - echo "REACT_APP_FIREBASE_PROJECT_ID=$REACT_APP_FIREBASE_PROJECT_ID" >> .env - echo "REACT_APP_FIREBASE_STORAGE_BUCKET=$REACT_APP_FIREBASE_STORAGE_BUCKET" >> .env - echo "REACT_APP_FIREBASE_MESSAGING_SENDER_ID=$REACT_APP_FIREBASE_MESSAGING_SENDER_ID" >> .env - echo "REACT_APP_FIREBASE_APP_ID=$REACT_APP_FIREBASE_APP_ID" >> .env artifacts: baseDirectory: build files: - '**/*' cache: paths: - node_modules/**/*
b. Next in the Environment variables add the key and value pair by clicking on manage variables and save.
-
Point to remember, now that hosting is on AWS Amplify. For OAuth redirects, Amplify should be recognised by Firebase as an authorised Domain. For this, in the Firebase console, go to
Authentication
, under that go toAuthorized domains
and add the domain namemain.dfzm4r2uqpd8p.amplifyapp.com
. -
Finally hosting is done, go ahead and use your application.
Happy Learning!