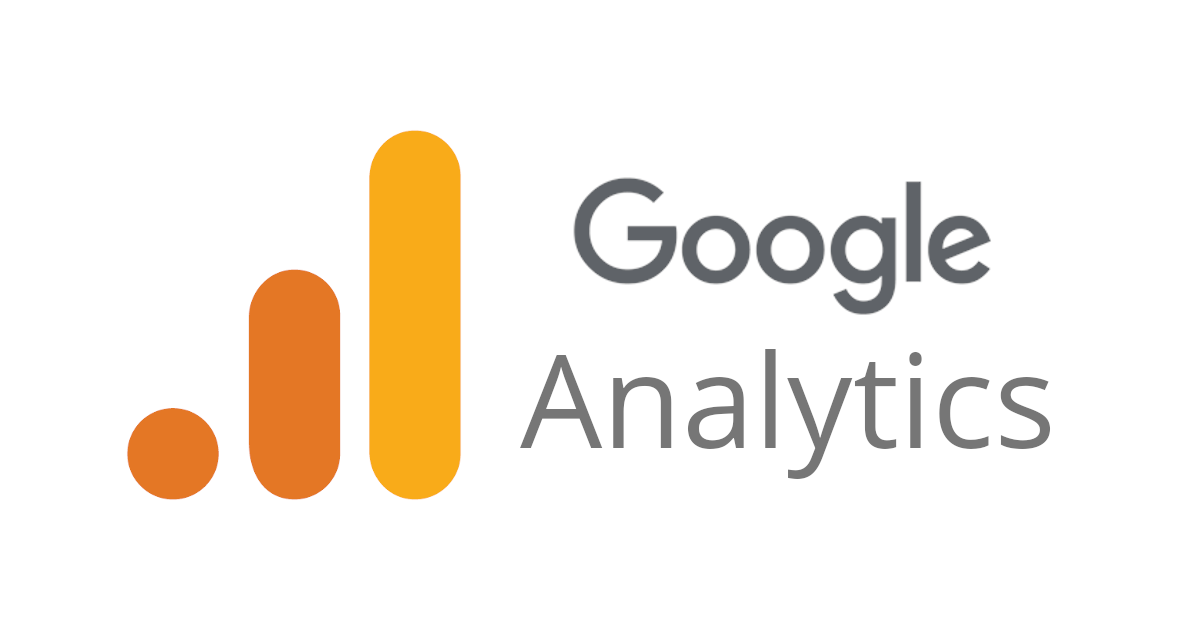
API v4を使いGoogle Analyticsからユーザーの行動履歴を取得する
はじめに
データアナリティクス事業本部のkobayashiです。
前回はユーザーアカウント認証でGoogle AnalyticsのデータをPythonでGoogle Analytics APIのV4でデータを取得してみました。
記事ではAPIを使ってページビュー数やセッション数を取得してみましたが、今回は同じAPIを使って個々のユーザーの行動履歴を取得してみます。
環境
- macOS Mojave
- Python 3.7.4
Google Analytics APIでユーザーの行動履歴を取得する方法
Analytics APIを使ってユーザーの行動履歴を取得するには
- reportRequestsとしてClientIDを取得する
- ClientIDごとにuserActivityを使って行動履歴を取得する
という手順になります。
Analytics API を使う準備に関しては前回の記事を参考にしてください。
では早速ユーザーの行動履歴を取得するコードを記述します。
Analytics APIを使ってデータを取得する
from googleapiclient.discovery import build from pprint import pprint import json import pydata_google_auth VIEW_ID = '<REPLACE_WITH_VIEW_ID>' # ユーザーアカウントを使用した認証 def initialize_analytics_reporting(): file = open(KEY_FILE_LOCATION, mode='r') credencial = json.load(file) scoped_credentials = pydata_google_auth.get_user_credentials( [ 'https://www.googleapis.com/auth/cloud-platform', 'https://www.googleapis.com/auth/analytics.readonly' ], client_id='{クライアントID}', client_secret='{クライアントシークレット}' ) return build("analyticsreporting", "v4", credentials=scoped_credentials) def get_activity(analytics, user_id): return analytics.userActivity().search( body={ "viewId": VIEW_ID, "user": { "type": "CLIENT_ID", "userId": user_id }, "dateRange": { "startDate": "2021-02-01", "endDate": "2021-02-20", } } ).execute() def get_report(analytics, next_page_token="0"): request_body = { "reportRequests": [{ "viewId": VIEW_ID, "pageToken": next_page_token, "pageSize": 100000, "samplingLevel": "LARGE", "dateRanges": [{"startDate": "2021-02-01", "endDate": "2021-02-20"}], "metrics": [{"expression": "ga:users"}], "dimensions": [{"name": "ga:clientId"}], "orderBys": [{"fieldName": "ga:users", "sortOrder": "DESCENDING"}] }] } return analytics.reports().batchGet(body=request_body).execute() def print_response(response): rows = [] for report in response.get("reports", []): column_header = report.get("columnHeader", {}) dimension_headers = column_header.get("dimensions", []) metric_headers = column_header.get("metricHeader", {}).get("metricHeaderEntries", []) next_page_token = report.get('nextPageToken') for row in report.get("data", {}).get("rows", []): row_data = {} dimensions = row.get("dimensions", []) date_range_values = row.get("metrics", []) for header, dimension in zip(dimension_headers, dimensions): row_data[header] = dimension for i, values in enumerate(date_range_values): for metricHeader, value in zip(metric_headers, values.get("values")): row_data[metricHeader.get("name")] = value rows.append(row_data) return rows, next_page_token def print_all_response(analytics): next_page_token = "0" rows = [] while next_page_token is not None: response = get_report(analytics, next_page_token) r, next_page_token = print_response(response) rows.extend(r) for _row in rows: print(_row['ga:clientId']) r = get_activity(analytics, _row['ga:clientId']) pprint(r) # pprint(srows) def main(): analytics = initialize_analytics_reporting() print_all_response(analytics) if __name__ == "__main__": main()
前回のページビュー数を取得するコード を流用しています。
Google Analytics APIでユーザーの行動履歴を取得する方法で説明した通り、get_report
関数でAnalyticsリポートのデータを取得しています。この中でmetrics
としてga:users
、dimensions
としてga:clientId
を指定して対象期間中にアクセスしたユーザーのClientIDを取得します。
次にClientIDごとにuserActivity().search()
を使って指定したClientIDの対象期間中の行動履歴を取得します。
実際にこのスクリプトを実行すると以下の様なデータが取得できます。
{'sampleRate': 1, 'sessions': [{'activities': [{'activityTime': '2021-02-16T12:56:40.378722Z', 'activityType': 'PAGEVIEW', 'campaign': '(not set)', 'channelGrouping': 'Organic Search', 'customDimension': [{'index': 1}, {'index': 2}], 'hostname': 'dev.classmethod.jp', 'keyword': '(not provided)', 'landingPagePath': '/articles/fukubernetes-node2-report/', 'medium': 'organic', 'pageview': {'pagePath': '/articles/fukubernetes-node2-report/', 'pageTitle': '【レポート】福岡で Kubernetes ' 'を語るコミュニティ「 ' '#ふくばねてす」に参加してきました | ' 'DevelopersIO'}, 'source': 'yahoo'}], 'dataSource': 'web', 'deviceCategory': 'mobile', 'platform': 'Android', 'sessionDate': '2021-02-16', 'sessionId': '33333333'}], 'totalRows': 1}, {'sampleRate': 1, 'sessions': [{'activities': [{'activityTime': '2021-02-19T10:19:40.916112Z', 'activityType': 'PAGEVIEW', 'campaign': '(not set)', 'channelGrouping': 'Organic Search', 'customDimension': [{'index': 1}, {'index': 2}], 'hostname': 'dev.classmethod.jp', 'keyword': '(not provided)', 'landingPagePath': '/articles/angular-flex-layout/', 'medium': 'organic', 'pageview': {'pagePath': '/articles/angular-flex-layout/', 'pageTitle': '[Angular8]サイトを簡単スタイリング!Angular ' 'Flex-Layoutの使い方をまとめてみた ' '| DevelopersIO'}, 'source': 'google'}], 'dataSource': 'web', 'deviceCategory': 'desktop', 'platform': 'Macintosh', 'sessionDate': '2021-02-19', 'sessionId': '22222222'}, {'activities': [{'activityTime': '2021-02-09T06:19:18.369666Z', 'activityType': 'PAGEVIEW', 'campaign': '(not set)', 'channelGrouping': 'Organic Search', 'customDimension': [{'index': 1}, {'index': 2}], 'hostname': 'dev.classmethod.jp', 'keyword': '(not provided)', 'landingPagePath': '/articles/angular6-ngx-chart/', 'medium': 'organic', 'pageview': {'pagePath': '/articles/angular6-ngx-chart/', 'pageTitle': '【NGX-Charts】超絶素晴らしいAngular6対応のグラフライブラリがあるからみんなに知ってほしい ' '| DevelopersIO'}, 'source': 'google'}], 'dataSource': 'web', 'deviceCategory': 'desktop', 'platform': 'Macintosh', 'sessionDate': '2021-02-09', 'sessionId': '11111111'}], 'totalRows': 2} ....
この様な形でデータが取得できるのであとはこのデータを加工して色々面白いデータを作れると思います。
まとめ
Google AnalyticsのデータをAnalytics API V4を使い、アクセスしたユーザーごとの行動履歴を取得してみました。これらのデータはGoogle Analyticsの画面で見ることもできますが、自分の使い慣れたツールで使いたいという要望もあるかと思いますので参考にしてみてください。
最後まで読んで頂いてありがとうございました。