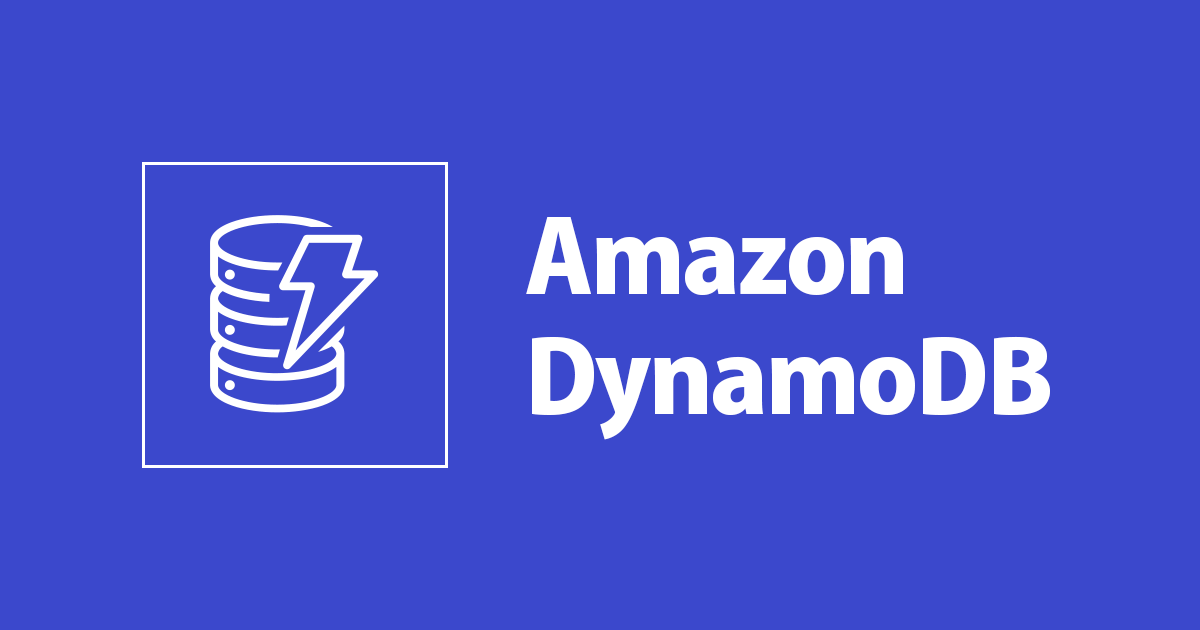
How To Put/Get Items From DynamoDB Table Using AWS Lambda (Boto3)
Hello, This is Shiwani Lawte from the DA Business Headquarters.
Today, we will learn how to Put/Get items from DynamoDB table using AWS Lambda.
Create Table In Amazon DynamoDB
First, Create a Table in DynamoDB by specifying Table Name and Partition Key.
- Go to the Amazon DynamoDB Console and Click on Create Table.
- Enter the Table Name and Partition Key. Click on Create Table.
-
Table has been created successfully.
Create IAM Role
In order to access the DynamoDB from AWS Lambda function, Create IAM role with the policy of 'read', 'write' and 'update' for the DynamoDB table. Attach this role to AWS Lambda Function.
- Go to the IAM Console and Click on Policies. Click on Create Policy.
-
Click on JSON and Create the following policy. Click on Next:Tags.
{ "Version": "2012-10-17", "Statement": [ { "Action": [ "dynamodb:GetItem", "dynamodb:PutItem", "dynamodb:UpdateItem", "dynamodb:DescribeTable" ], "Effect": "Allow", "Resource": "*" } ] }
- Tags[Optional]. Click on Next.
-
Enter the Name of the Policy. Click on Create Policy.
-
Policy has been created Successfully.
-
Go to the Roles. Click on Create Role.
-
In Select Trusted entity , Select Trusted identity type : AWS Service and Use Case : Lambda. Click on Next.
-
In add permissions, Select the following Permissions Policy.
- AWSLambdaBasicExecutionRole
- access_dynamodb_from_lambda
Enter the Role name . Click on Create Role.
Create AWS Lambda Function
- Go to the AWS Lambda Function Console. Click on Create Function.
-
Enter the basic information as shown in image. Attach the Execution Role which we have created already. Click on Create Function.
Code To Put Item In DynamoDB Table
PUT method is use to create an item in Amazon DynamoDB table. Write the following code in Lambda to PUT item in DynamoDB Table.
import json import boto3 client = boto3.client('dynamodb') def lambda_handler(event, context): PutItem = client.put_item( TableName='Customer', Item={ 'id': { 'S': '01' }, 'name': { 'S': 'XYZ' } } ) response = { 'statusCode': 200, 'body': json.dumps(PutItem) } return response</code>
- Item has been created successfully.
-
'S' indicates a String value, 'N' is a numeric value
- The Python SDK for AWS is Boto3. Boto3 is use to interact with AWS services including DynamoDB. For more information, refer to the official documentation of Boto3. Dynamodb-Boto3 Documentation
Code To Get Item From DynamoDB Table.
GET method is use to get an item by primary key in DynamoDB table. Write the following code in Lambda to GET item from DynamoDB Table.
import json import boto3 client = boto3.client('dynamodb') def lambda_handler(event, context): GetItem = client.get_item( TableName='Customer', Key={ 'id': { 'S': '01' } } ) response = { 'statusCode': 200, 'body': json.dumps(GetItem) } return response</code>
Execution result
Response { "statusCode": 200, "body": "{\"Item\": {\"id\": {\"S\": \"01\"}, \"name\": {\"S\": \"XYZ\"}}, \"ResponseMetadata\": {\"RequestId\": \"706J0D7NTFMSR6QKG5S7Q87RGBVV4KQNSO5AEMVJF66Q9ASUAAJG\", \"HTTPStatusCode\": 200, \"HTTPHeaders\": {\"server\": \"Server\", \"date\": \"Tue, 18 Apr 2023 06:58:38 GMT\", \"content-type\": \"application/x-amz-json-1.0\", \"content-length\": \"45\", \"connection\": \"keep-alive\", \"x-amzn-requestid\": \"706J0D7NTFMSR6QKG5S7Q87RGBVV4KQNSO5AEMVJF66Q9ASUAAJG\", \"x-amz-crc32\": \"988539630\"}, \"RetryAttempts\": 0}}" }
Conclusion
Amazon DynamoDB is a fully managed NoSQL database service that provides fast and predictable performance with seamless scalability. In this blog we have discussed about creating DynamoDB Table and methods to interact with a Amazon DynamoDB database from a AWS Lambda function using Boto3.