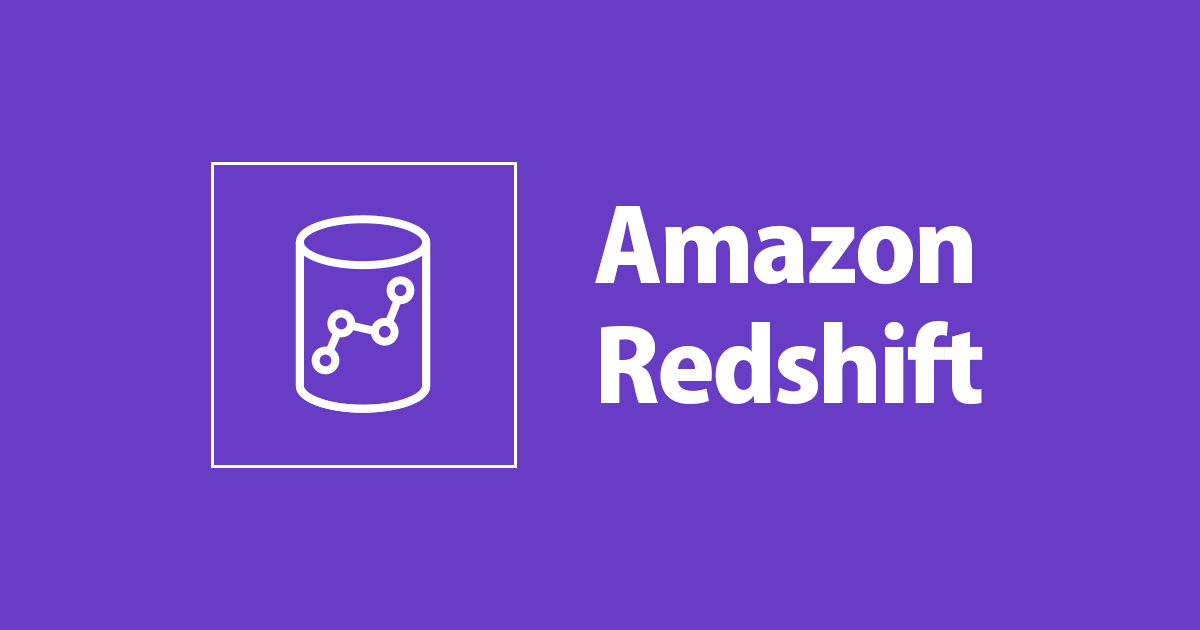
I tried connecting to Amazon Redshift using the Amazon Redshift Python connector (redshift_connector)
Introduction:
In this blog, I will share my experience of connecting to Amazon Redshift using the redshift_connector package in Python.
What is Redshift
Amazon Redshift is a fully managed, petabyte-scale data warehouse service in the cloud that makes it simple and cost-effective. Amazon Redshift lets you access and analyze data without all of the configurations of a provisioned data warehouse.
Amazon Redshift is a cloud-based data warehouse that allows users to store and analyze large amounts of data in a scalable and secure way.
What is redshift_connector
redshift_connector is the Amazon Redshift connector for Python that helps to connect Amazon Redshift with Python. It can integration with pandas and numpy, as well as support for numerous Amazon Redshift specific features help you get the most out of your data.
It Supports following Amazon Redshift features :
- IAM authentication
-
Identity provider (IdP) authentication
-
Redshift specific data types
For more information : redshift-connector
Step 1: Install redshift_connector package
First, Let's install the redshift_connector package using pip. Run the following command on terminal or command prompt :
pip install redshift_connector
For more information : Methods to install the Amazon Redshift Python connector
Step 2: Import the redshift_connector package
Next, Import the redshift_connector package in your Python code using the following command:
import redshift_connector
Step 3: Establish a connection to Amazon Redshift
You need to provide your Amazon Redshift database credentials to establish a connection. Use the following connect method of redshift_connector to establish a connection. Specify the following information to establish the connection :
- host: The endpoint of your Redshift cluster.
- port: The port number to connect to.
- database: The name of the database to connect to.
- user: The username to use for authentication.
- password: The password to use for authentication.
The redshift_connector.connect() function takes these parameters as keyword arguments and uses them to establish a connection to the Redshift cluster. The resulting conn object is a connection to the Redshift cluster that can be used to execute SQL statements.
conn = redshift_connector.connect( host='my-redshift-cluster.us-west-2.redshift.amazonaws.com', port=5439, database='my_database', user='my_username', password='my_password' )
Replace the values of host, port, user, password, and database with your own Redshift connection details.
Step 4: Create a cursor object
The conn.cursor() method is used to create a cursor object.
cursor = conn.cursor()
Step 5: Execute a SQL query
Try to execute a simple SQL query to test the connection. The cursor.execute() method is use to execute SQL statement.
cursor.execute('SELECT * FROM mytable') results = cursor.fetchall() for result in results: print(result)
This code will fetch all the rows from a table called mytable and then print them to the console.
Step 6: Close the connection
Finally Close the connection. The conn.close() method is use to close the connection.
conn.close()
Conclusion:
This blog discussed basic steps for using the redshift_connector package to connect to a Redshift cluster and execute SQL statements using Python. With the redshift_connector package, it is easy to establish a connection to Amazon Redshift from Python and execute SQL queries. The redshift_connector package also provides additional features such as support for IAM authentication, which makes it a useful tool for any Python developer working with Amazon Redshift.