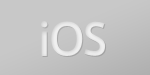
iOSアプリ開発でZXingObjCを使ってQRコードを生成する
今回は、iOSアプリ開発で ZXingObjC ライブラリを使い、文字列から QRコード を生成するサンプルを作ってみたいと思います。
ちなみに、Android版のQRコードの生成方法については、Android Tips #42 ZXing ライブラリ (2.1) を使って QR コードを生成する を参考にしてください。
環境構築
前回と同じ、以下の環境を使用します。
- ZXingObjC 2.1.0
- Xcode 4.6
- iOS SDK 6.1
- iPod touch 5th
プロジェクトの作成、ライブラリの設定方法は、前回と同じなので 前回のブログ( iOSアプリ開発でZXingObjCを使ってQRコードを読み取る ) を参考にしてください。
サンプルの実装
以下の部品を、画面の上から順に配置します。
- UITextField:QRコードにする文字列を入力するテキストフィールド
- UIButton:QRコード生成ボタン
- UIImageView:QRコードイメージ(240 x 240)
配置するとこんな感じになります。
次に、ヘッダーファイルを作成します。
#import <UIKit/UIKit.h> #import <ZXingObjC/ZXingObjC.h> @interface ViewController : UIViewController @property (weak, nonatomic) IBOutlet UITextField *qrcodeTxt; @property (weak, nonatomic) IBOutlet UIImageView *imageView; - (IBAction)createQrCodePressed:(id)sender; @end
- L2:ZXingObjCをインポートします。
- L6:画面の最上部に配置したテキストフィールドと「qrcodeTxt」を接続します。
- L7:画面の上から3番目に配置したQRコードイメージと「imageView」を接続します。
- L9:画面の上から2番目に配置したQRコード生成ボタンと「createQrCodePressed」を接続します。
最後に、実装ファイルを作成します。
#import "ViewController.h" @interface ViewController () @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; } - (void)viewDidAppear:(BOOL)animated { [super viewDidAppear:animated]; } - (IBAction)createQrCodePressed:(id)sender { [self createQrCode:self.qrcodeTxt.text]; // ソフトウェアキーボードを閉じます。 [self.qrcodeTxt resignFirstResponder]; } - (void)createQrCode:(NSString *)qrcodeTxt { if (qrcodeTxt == nil || [qrcodeTxt isEqualToString:@""]) { self.imageView.image = nil; return; } // QRコードを生成します。 ZXMultiFormatWriter* writer = [[ZXMultiFormatWriter alloc] init]; CGSize imageSize = self.imageView.frame.size; ZXBitMatrix* result = [writer encode:qrcodeTxt format:kBarcodeFormatQRCode width:imageSize.width height:imageSize.height error:nil]; if (result == nil) { self.imageView.image = nil; return; } // QRコードを表示します。 CGImageRef qrImageRef = [ZXImage imageWithMatrix:result].cgimage; self.imageView.image = [UIImage imageWithCGImage:qrImageRef]; } @end
- L28:生成したQRコード画像がソフトウェアキーボードに隠れてしまうので、ここでソフトウェアキーボードを閉じます。
- L38 ~ L44:QRコードを生成しています。
- L50 ~ L51:生成したQRコード画像を画面に表示しています。
シンプルですが、これで実装完了です。
動作確認
では、動かしてみます。
起動したらテキストフィールドをタップして、「http://classmethod.jp」と入力します。
そして、「QRコード生成」ボタンをタップします。
入力した文字列のQRコードが生成されました!
ちなみに、環境設定や使い方については、
https://github.com/TheLevelUp/ZXingObjC#readme と、
付属のサンプル( ZXingObjC-2.1.0/examples/QrCodeTest/QrCodeTest.xcodeproj )を参考にするとよいでしょう。
ではでは。