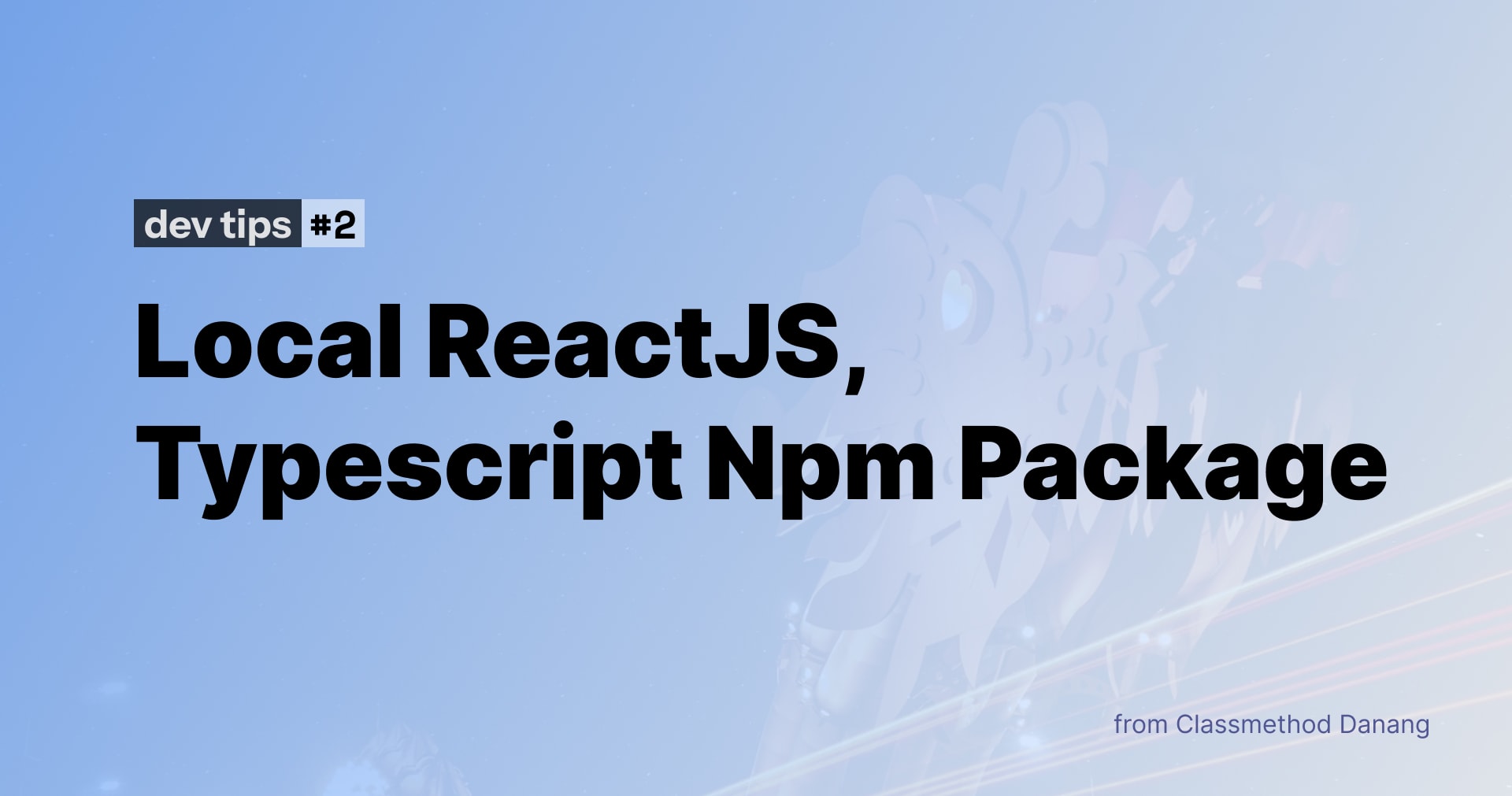
Local ReactJS, Typescript Npm Package
When working on multiple front-end projects, you might discover that many of your React code could be reused across different projects. But how can we achieve that? An npm package allows us to do just that. You can write your code once and use it whenever you need.
In this article, I will guide you through the process of creating an npm package using React and TypeScript, and show you how to use it locally.
1. How to create your own package
1.1. Requirement
- Npm
- Git & Github account
1.2. Project setup
First, you need to create a folder for your npm package. Run the following, replacing <packagename>
with your chosen name:
mkdir <packagename> && cd <packagename>
1.3. Git setup
You can initialize a new repository by running the following command.
git init
After that, you want to create a .gitignore file with the content below.
# .gitignore
node_modules
.vscode
1.4. Npm setup
Now we can set up npm. Run the command below to set up your npm package. Leave all the prompts as default except the entry point, you want it to be index.ts
npm init
The output package.json file should be similar to this.
{
"name": "myPackage",
"version": "1.0.0",
"main": "index.ts",
"scripts": {
"test": "echo "Error: no test specified" && exit 1"
},
"author": "",
"license": "ISC",
"description": ""
}
1.5. Setting up Typescript
After creating our npm package, we need to install TypeScript:
npm install --save-dev typescript
This command installs TypeScript as a development dependency, which means it will not be included in the final package since it is not needed once our code is built. Next, we need to set up the TypeScript compiler:
npx tsc --init
Now, replace the contents of this file with the following:
{
"include": ["src"],
"compilerOptions": {
"target": "es2016" ,
"module": "commonjs",
"declaration": true,
"outDir": "dist",
"esModuleInterop": true ,
"forceConsistentCasingInFileNames": true,
"strict": true,
"skipLibCheck": true,
"jsx": "preserve"
}
This will ensure our typescript files are generated correctly for our package and in the right location.
1.6. Package.json Setup
We would like to make a few changes to the package.json
file.
Firstly, we want to include only our distribution (dist) files in the package. Next, we plan to add React as a peerDependencies
.
Additionally, we need to create a build script to compile our code.
Finally, it’s important to export the correct files so that we can import them into other projects.
Our package.json file should look like the following:
{
"name": "<mypackage>",
"version": "1.0.0",
"description": "",
"main": "./dist/index.js", // Set compiled file as main
"files": [
"dist" // Only export dist directory
],
"exports": { // Setup exports with typing
".": {
"types": "./dist/src/index.d.ts",
"default": "./dist/src/index.js"
}
},
"scripts": {
"build": "tsc" // Build our project
},
"author": "",
"license": "ISC",
"devDependencies": {
"typescript": "^5.7.3"
},
"peerDependencies": {
"react": "^18.2.0" // Include react as a peer dependency
}
}
1.7. Write code for the package
Create a new folder named src
Inside this folder, create a file called index.ts
This file will serve as the entry point for our package. From this point, we can import functions from other files and export any items that we want to make available from our package.
For example:
import { functionA } from “./contexts/functionA”
import { functionB } from “./hooks/functionB”
export { functionA, functionB}
For the purpose of this tutorial, we'll create a simple function to export. So we just need to add the following code to "index.ts":
/* src/index.ts */
export const myComponent = () => {
return (
<p>Hello World!~</p>
)
}
2. How to use your package locally
First, you need to build your package by running:
npm run build
Then you want to move your app that needs to install the package, Remember to replace ../packagename with your package location you have created at the first step, and run:
npm i ../packagename
Inside your package.json file, in dependencies will have the line:
“<mypackage>”: “file:..//packagename”
Now you are able to use your package with simple import, remember the package name is the name inside the package.json of your package file, not the folder itself.
import { myComponent } from "<myPackage>"
const test = () => {
return <myComponent/>
}
3. How you can store your package on GitHub
If you want to store your package for easy access or to share it with friends or colleagues, here's how to do it.
First, create a new repository on GitHub. Then, follow the steps provided by GitHub to link your local package with the repository and push your code. If you want to keep the repository private for your own use, be sure to set it to private during the creation process.
When you need to use the package, you just need to put this line to dependencies of your project package.json
“<yourPackage>”: “git+ssh://yourGithubRepository.git”
You can choose whatever protocol you want: git, git+ssh, git+https in my case I use git+ssh. Also, you can provide the tag for each version you want to install like #v0.0.1 in the last of the command.
More information about this you can find it here:
4. Conclusion
Creating and sharing npm packages is a powerful way to modularize code, collaborate with other developers in your project. Throughout this guide, we've covered the essential steps to create, develop, and publish your own npm package. By following this process, you've gained valuable skills in creating reusable React and TypeScript modules. These skills will serve you well whether you're working on personal (private) projects or contributing to larger open-source efforts.
Happy coding!