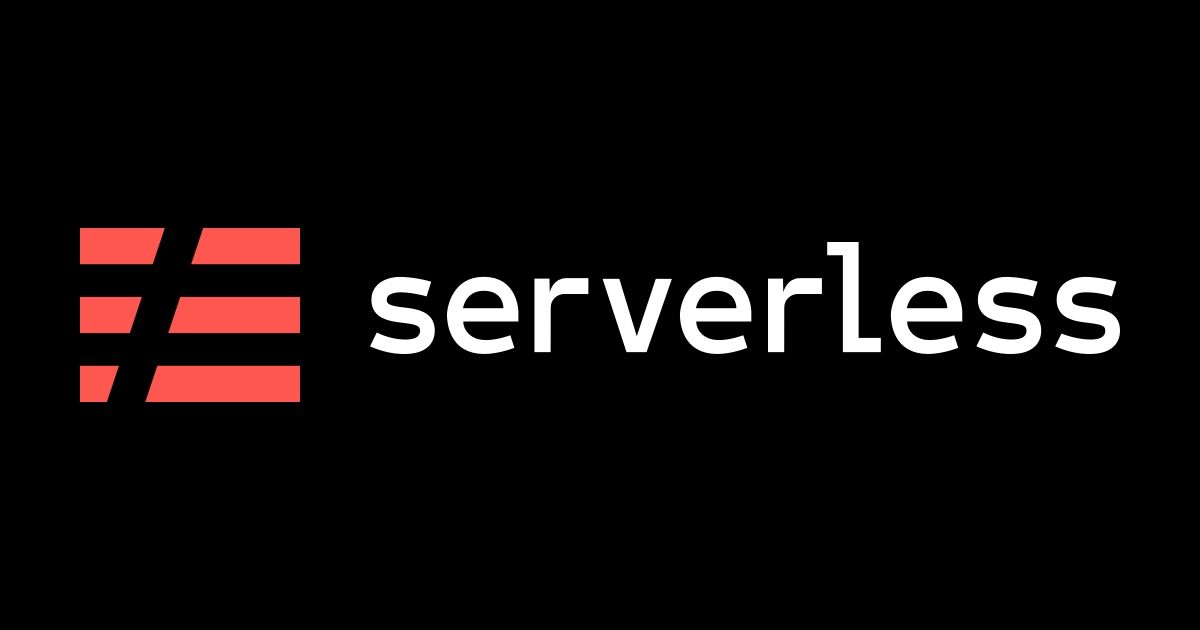
How to map lambda error to API Gateway error response with Serverless Framework
Serverless Framework provides an easy way to deploy aws serverless applications. In this article, I'll show you how to build a simple REST API handling error from Lambda Function.
Prerequisite
- AWS account
- Node.js
- AWS CLI (configured).
Sample Repository and Usage
git clone git@github.com:cm-wada-yusuke/sls-lambda-error-api-integration-sample.git cd sls-lambda-error-api-integration-sample yarn install yarn deploy # sls deploy
Check 1: Create a REST Resource for handling error
I updated serverless.yml as shown below.
service: api-response-codes provider: name: aws runtime: nodejs12.x plugins: - serverless-offline functions: hello: handler: handler.hello events: - http: method: get path: whatever integration: lambda response: headers: Content-Type: "'application/json'" template: $input.path('$') statusCodes: 200: pattern: "" # Default response method template: $input.path("$.body") 404: pattern: '.*"statusCode":404,.*' # JSON response template: $util.parseJson($input.json('$.errorMessage')) # JSON return object headers: Content-Type: "'application/json'"
Highlighted object deploys API Gateway Integration Response
:
Check 2: Create a Lambda Function that responds error status
Lambda code is simple, but note an error mapping with the API Gateway:
- Our API Gateway's
Lambda Error Regex
runs a check when throwing an exception in Lambda Function. It does not run on a regular response. - Our Lambda Function packs the exception into an object called
errorMessage
by herself.
See documentation for more details.
Handle Lambda errors in API Gateway - Amazon API Gateway
I've written a Lambda Function to cause errors on purpose.
exports.hello = async (event, context) => { const error = true; if (error) { throw new Error(JSON.stringify({ statusCode: 404, message: "order not found" })); } else { return { body: 'Hello from Lambda!', }; } };
throw new Error(JSON.stringify({statusCode: 404,message:"order not found"}));
- this error will be packed to another object:
{ "errorMessage": "{\"statusCode\":404,\"message\":\"order not found\"}", "errorType": "somestring", "stackTrace": [ "somestring", ... ] }
In the API Gateway, errorMessage
is checked with regex pattern -- .*"statusCode":404,.*
in this time. Finally, the API call failed to get and respond 404
code and parsed the error message.
Conclusion
In this article, I showed you how to create API Gateway integration response handling Lambda Function error.