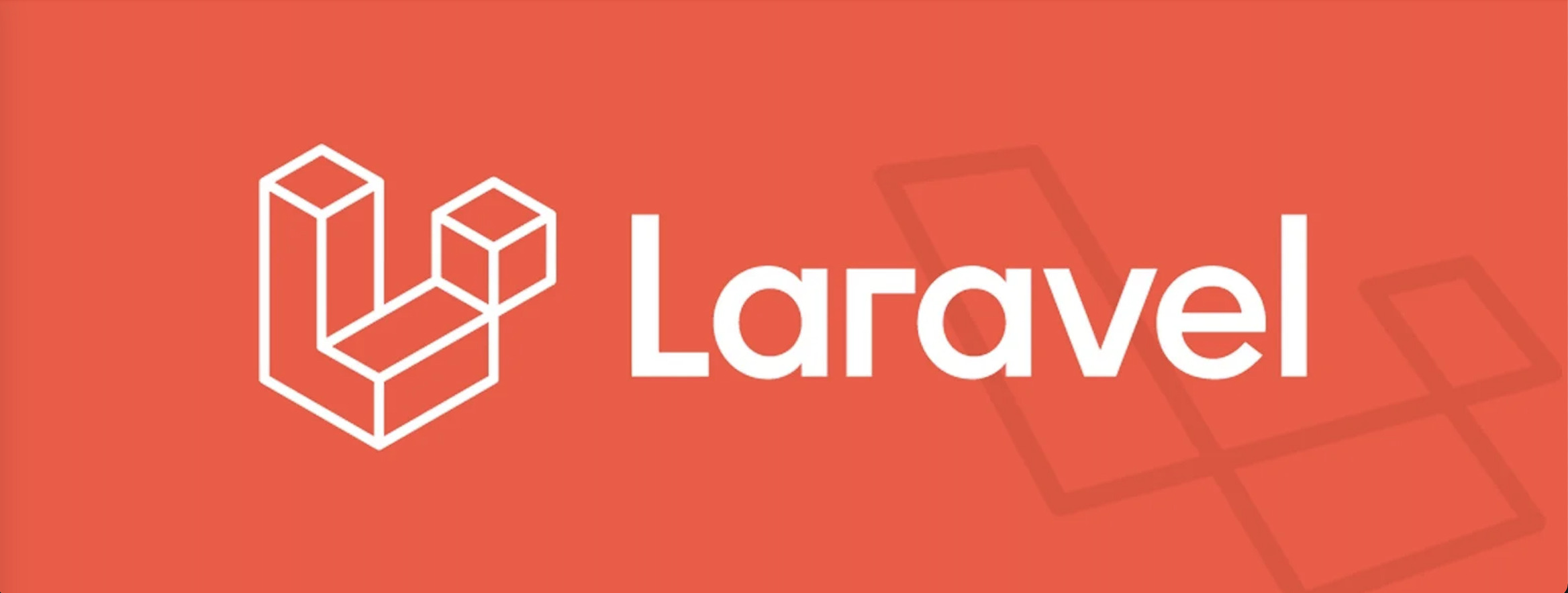
php Laravel : Installation and Basics
Laravel is an open source php framework which makes it easier to make web applications. Laravel is based upon the MVC pattern.Laravel updates every 6 months and releases an LTS (Long Term Support) version every 2 years adding new features which keeps it up to date with industry’s trends and needs.
If you want to build products quickly in php and need tedious stuff to be taken care of then Laravel is your answer.
Getting Started
To get started with Laravel we need to have Laravel on our local system. We use ‘composer’ package manager to get install Laravel. Composer is a package manager which is essentially used to manage php packages.
- To install composer, copy paste the following code in your terminal and run it
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');"php -r "if (hash_file('sha384', 'composer-setup.php') === '756890a4488ce9024fc62c56153228907f1545c228516cbf63f885e036d37e9a59d27d63f46af1d4d07ee0f76181c7d3') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;" php composer-setup.php php -r "unlink('composer-setup.php');" php -r "unlink('composer-setup.php');"
- Now move the composer.phar file to a $PATH folder so that you can use composer command directly, to do this run the following command.
sudo mv composer.phar /usr/local/bin/composer
- Go to Install with composer or install using docker desktop.
- Follow the instructions to install Laravel on your system.
- Run the following command
laravel new project-name
OR
composer create-project --prefer-dist laravel/laravel:^8.0 project-name
This will create a new directory with the name ‘project-name’ and install all dependencies in it.
Understanding the files
In the list of files, remember to not push the .env file to remote repositories, it should be in your gitignore. The reason being that it contains information very specific to the local environment.
Image: Local environment configuration including database configuration.
For the production environment we’ll have a separate .env file. With the details of the production environment.
Although, the .env.example file does go into version control so if you do want to share your config with others then you can push them in the .env.example file and then let others rename the file after they pull it.
Laravel has its own inbuilt server which can be used for testing only. So here we can use
php artisan serve
This will start the local server. You can view it on : http://127.0.0.1:8000
All commands in a docker installation are similar to a composer installation, except for using sail instead of php, so the command becomes:
sail artisan serve
You can read more about it here.
Artisan is the command line interface included with Laravel which has a set of commands which help in building applications. To know more run :
php artisan list
You can guess the command which is to be used in a docker installation.
The artisan file has the launch configurations for the artisan server. We can add startup and shutdown events through this file.
web.php can be found in routes directory, this is where you define all of your routes.
Image : A custom route /hello returns the defined output
Here view is Laravel’s templating system, in which it is calling a view called welcome and each view is just a simple html file. These are stored in resources/views/
This is the Viewing part of Laravel. Now we move to Controller.
The command
php artisan make:controller controller-name
will generate the controller classes for us. Find the file controller-name.php inside app/Http/Controllers . In this file we can define functions which can be called from web.php for displaying web pages, this is very helpful when we want to define complex logic while displaying the web pages.
I defined a function to display another template page for the path /hello inside the controller.
Now coming to the Model side of things.
php artisan make:mode model-name
This will make a model named model-name and a Message.php file in app/Models/. It is a naming convention in Laravel that we follow-up name of Controllers with Controller and do not do so with models.
This model can now interact with databases to perform operations on or with data.Since Laravel is made easy to deploy the easier way to transfer table data information from one system to another is to define the schema in a file and then later run it to get the tables locally.
Now we can create a migration by
php artisan make:migration migration-name
This will create a migration with a timestamp of time of creation as a suffix of its name, this migration essentially is a php file with 2 functions up() and down() the former is for running the migration and the latter is for reversing it, here you can define the schema of your database.
php artisan migrate
This command will install all the tables according to the migrations present in database/migrations . After creating your migration, you will see 4 migrations present in the folder, 4 of which ship with laravel, you can have a look at these and understand their syntax to get an idea on how to define a general schema.
Conclusion
Laravel is quite versatile and easy to use. This was just an overview of Laravel with some understanding about the files and general syntax which will help us a long way in understanding the basics of Laravel.