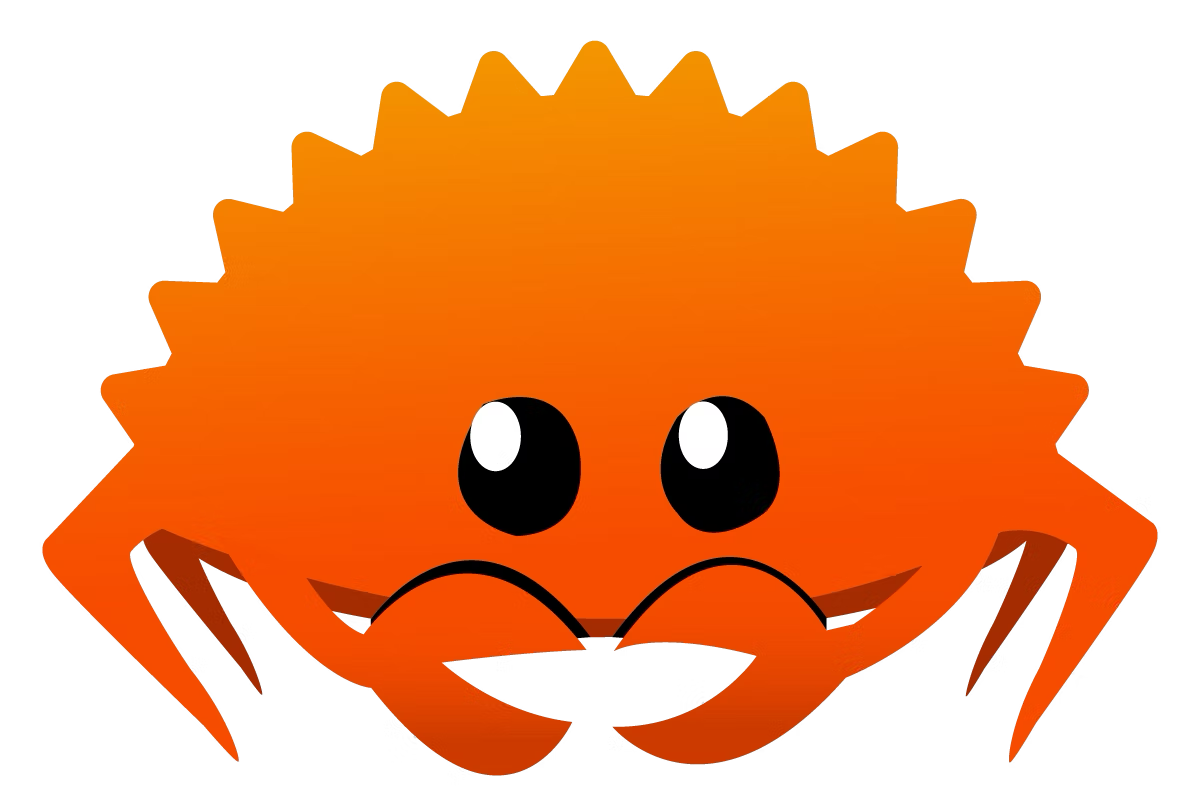
Rust – Conditions and loops
IF CONDITION
The if
statement is used to conditionally execute a block of code based on the value of an expression. The syntax is as follows. You don't need parentheses around the condition, because everything between the "if" and the opening curly brace is the condition.
if condition { // code to execute if the condition is true } else { // code to execute if the condition is false }
Example:
if num == 0 { mgs = "zero"; } else if num > 0 { mgs = "positive"; } else { mgs = "negative"; }
The if
statement can also be used as an expression, meaning it can return a value. The syntax is similar to a regular if statement, except for a few things:
- There are no semicolons after the branch values to make it so that the values get returned from the blocks as tail expressions. But there is a semicolon at the end of the if expression.
- We can't use return for this purpose, even if we wanted to, because return only applies to blocks that are function bodies, so return would return out of the current function.
- All the blocks must return the values that are of the same data type.
Example:
let num = 5; let value = if num > 0 { "positive" } else { "not positive" };
FOR LOOP
The for
loop in Rust is used to iterate over a range, an iterator, or a collection. It is a powerful and flexible construct for controlling the flow of your program.
for
Loop Over a Range:
The syntax for a range is two dots separating your start and end points. The start is inclusive and the end is exclusive. So this will count from zero to nine, and if you use dot dot equal then the end will be inclusive as well (..=10).
for i in 0..10 { println!("The value of i is: {}", i); }
for
Loop Over an Iterator:
The iter
method is one of the most common ways to get an iterator. This for loop can be used with any type that implements the Iterator trait, including vectors, arrays, and other collections. The iterator you use determines which items are returned and the order that they are returned in. In the below example, the vec!
macro is used to create a vector of numbers. The iter() method is called on the vector to generate an iterator over its elements.
let numbers = vec![1, 2, 3, 4]; for number in numbers.iter() { println!("{}", number); }
for
Loop Over a Collection:
The for loop can also be used to iterate over a collection. A collection is any type that implements the IntoIterator
trait, which allows it to be converted into an iterator. Here is an example of a for loop over a collection:
let numbers = vec![1, 2, 3, 4]; for number in numbers { println!("{}", number); }
WHILE LOOP
The while
loop is used to execute a block of code repeatedly as long as a condition is met. The condition is evaluated before each iteration of the loop and if the condition is true, the loop body is executed. If the condition is false, the loop terminates and the program continues with the next statement.
let mut x = 5; while x != 0 { println!("The value of x is: {}", x); x -= 1; }
LOOP
A loop expression denotes an infinite loop. Aparts general execution flow, break
and continue
are the control flow modifiers. Break is used to exit the loop when a certain condition is met.
let mut y = 5; loop { println!("The value of y is: {}", y); y -= 1; if y == 0 { break; } }
Rust also has a mechanism to break from a nested loop. For this, annotate the loop you want to break out of with a label. Here the label is named "dropout". They have a strange syntax with a single apostrophe prefixed to an identifier and followed by a colon. With the identifier being mentioned, when the code hits the break statement in the inner loop, it will break all the way out of the outermost loop. Isn't it interesting?
'dropout: loop { loop{ loop{ break 'dropout; } } }
Continue also follows the same syntax and also works the same as break, where you can jump and continue from any nested location to any labeled outer loop.
loop { 'jumphere: loop{ loop{ continue 'jumphere; } } }
Thank you, Happy learning !!