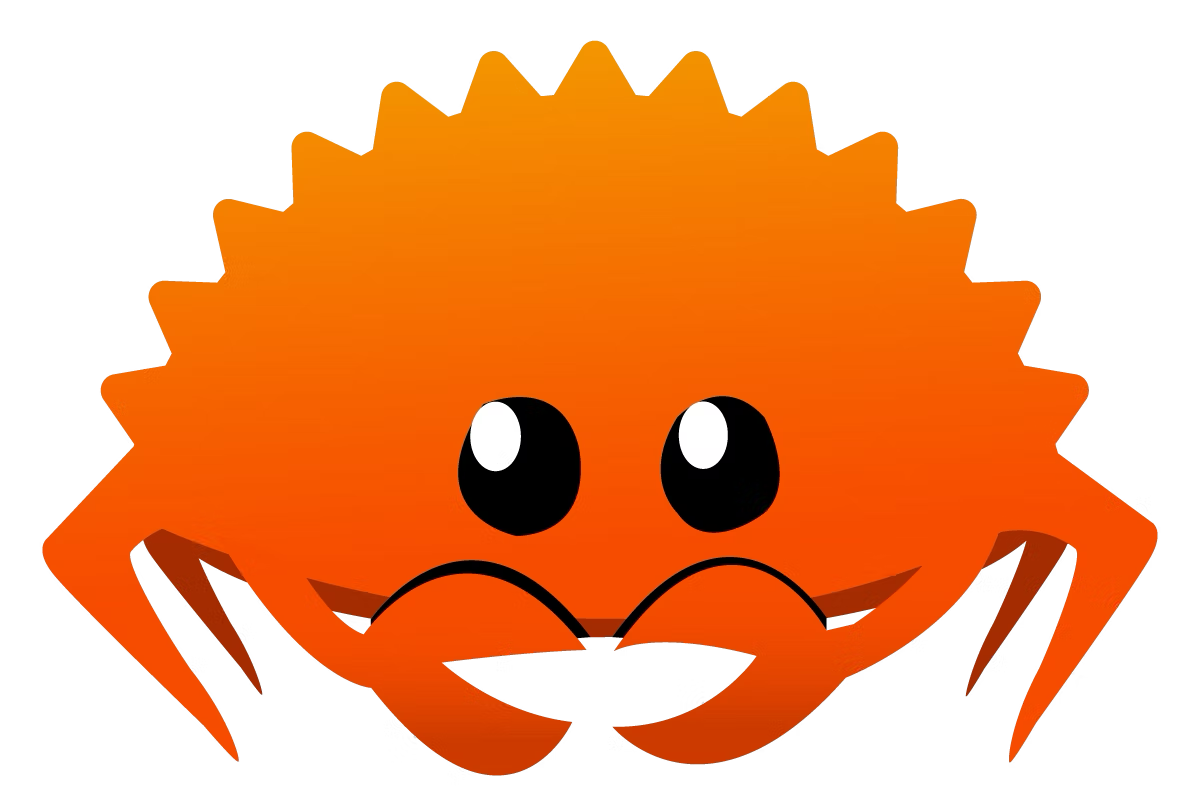
Rust – Functions and Closures
FUNCTIONS
In Rust, a function is a block of code that performs a specific task and can be executed by calling its name. Functions are declared using the keyword fn, followed by the function name, a set of parentheses, and a block of code.
The Rust style guide says to use snake-case for function names: lowercase words separated by underscores. Parameters are always defined with "name: type" and, of course, multiple parameters are separated by a comma. Then we specify the return type after the "->" symbol.
For example:
fn multiply(x: i32, y: i32) -> i32 { return x * y; }
Returning values also has a shortcut. The final expression in a block will be returned as the block's value if the semicolon is left off. This is called a "tail expression" and looks like
fn multiply(x: i32, y: i32) -> i32 { x * y }
CLOSURES
Rust also has the concept of closures, which are anonymous functions that can capture variables from the surrounding scope. Closures are often used in functional programming and can be passed as arguments to other functions or used in function expressions.
Closures are defined using the "pipe" symbol "|" followed by the parameter list and the closure body. The parameter list and the return type of closure are optional.
let mul = |x: i32, y: i32| x * y; let result = mul(3, 4);
Closures can also capture multiple variables, and also can be defined with an explicit return type.
let x = 5; let y = 10; let mul_xy = |z: i32| -> i32 {x * y * z}; let result = mul_xy(3);
Closures are also useful when we want to pass function as a parameter. For example, in the following code, the function complex takes a closure as an argument, which is executed with the passed value:
let x = 5; fn complex(num: i32, f: impl Fn(i32)-> i32) -> i32 { f(num) } let mul = |y: i32| -> i32 {x * y}; let result = complex(3, mul);
It's important to note that, functions are first-class citizens, meaning that they can be assigned to variables, passed as arguments to other functions, and returned as values from functions. This allows for a high degree of flexibility and functional programming capabilities.
Thank you, Happy learning.