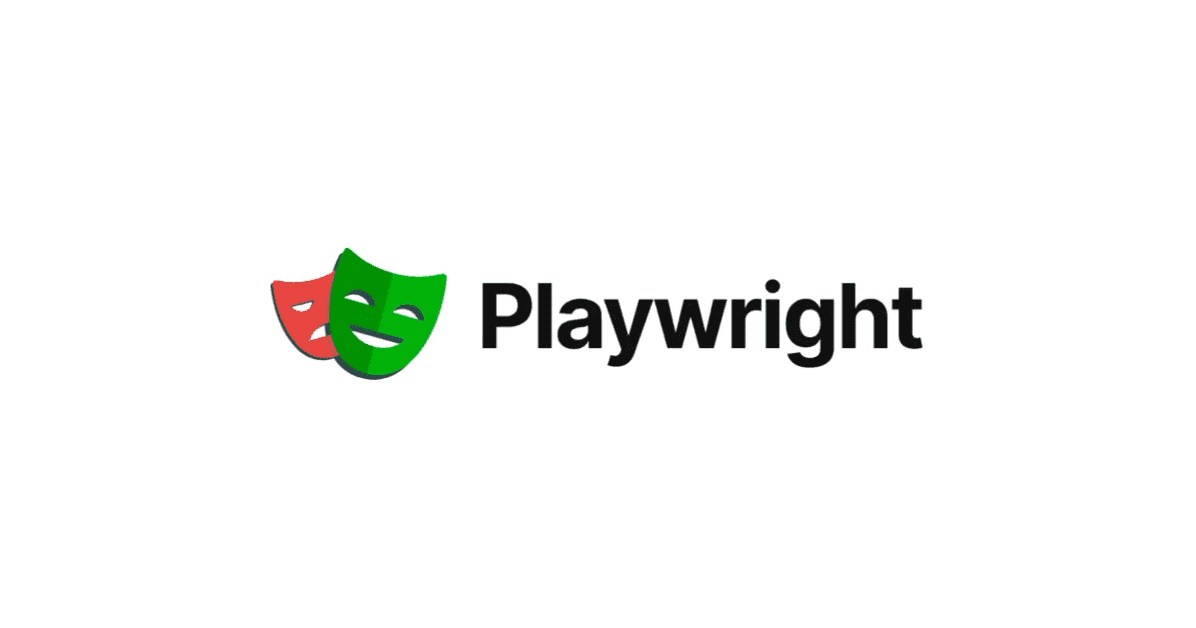
SalesforceのレポートをPlaywrightで自動ダウンロードする
Salesforceのレポートを手動でダウンロードするのは手間がかかることがあります。特に、定期的にデータを取得し、分析や他のシステムとの連携を行いたい場合、Playwright を活用すると、自動化が可能になります。
本記事では、Playwright を用いて Salesforce のレポートを CSV 形式で自動ダウンロードする方法 を紹介します。
1. Playwright とは?
Playwright は、ブラウザ操作を自動化できる強力なテストフレームワークです。
マルチブラウザ対応(Chromium, Firefox, WebKit など)であり、ヘッドレスモードでも動作するため、CI/CDパイプラインなどでも活用できます。
2. Salesforce のレポートを Playwright でダウンロードする流れ
(1) Salesforce にログイン
(2) レポートページへ移動
(3) iframe 内のレポートを操作
(4) CSV エクスポートを選択
(5) ダウンロードした CSV を保存
以下のコードで、これらの操作を自動化できます。
3. Playwright スクリプト
import { test, expect } from '@playwright/test';
import fs from 'fs';
test('Salesforceからレポートをダウンロード', async ({ page }) => {
// 1. Salesforce ログインページを開く
await page.goto(process.env.SALESFORCE_LOGIN_URI);
// 2. ログイン情報を入力
await page.fill('#username', process.env.SALESFORCE_USERNAME);
await page.fill('#password', process.env.SALESFORCE_PASSWORD);
await page.click('#Login');
// 3. レポート画面へ移動
await page.goto(process.env.SALESFORCE_DOWNLOAD_REPORT_URI);
// 4. iframe 内のレポートを取得
const frame = page.frameLocator('iframe[title="レポートビューアー"]');
await frame.locator('div#report-main').click();
// 5. エクスポートボタンをクリック
const element = await frame.locator('xpath=//div[contains(@class, "action-bars")]//button').nth(-1);
await element.click();
await frame.getByRole('menuitem', { name: 'エクスポート' }).click();
// 6. ダウンロード設定
await page.getByText('詳細行のみをエクスポートします。').click();
await page.getByLabel('形式').selectOption('localecsv');
await page.getByLabel('文字コード').selectOption('UTF-8');
// 7. CSV ダウンロード処理
const [download] = await Promise.all([
page.waitForEvent('download'), // ダウンロードイベントを待機
page.getByRole('button', { name: 'エクスポート' }).click(),
]);
// 8. ファイルを保存
const filePath = './salesforce_report.csv';
await download.saveAs(filePath);
console.log(`CSVがダウンロードされました: ${filePath}`);
// 9. ファイル内容を確認
const fileContent = fs.readFileSync(filePath, 'utf-8');
console.log('CSVの内容:', fileContent.split('\n').slice(0, 5)); // 最初の5行を表示
});
4. コードの解説
(1) Salesforce へログイン
await page.goto(process.env.SALESFORCE_LOGIN_URI);
await page.fill('#username', process.env.SALESFORCE_USERNAME);
await page.fill('#password', process.env.SALESFORCE_PASSWORD);
await page.click('#Login');
環境変数 SALESFORCE_LOGIN_URI
, SALESFORCE_USERNAME
, SALESFORCE_PASSWORD
を利用することで、パスワードのハードコーディングを防ぎます。
(2) レポートページへ移動
await page.goto(process.env.SALESFORCE_DOWNLOAD_REPORT_URI);
環境変数 SALESFORCE_DOWNLOAD_REPORT_URI
を使ってレポートに移動しています。
実際のURLは https://[your salesforce org domain]/lightning/r/Report/[レポートID]/view?queryScope=userFolders
などになるでしょう。 .env
ファイルに SALESFORCE_DOWNLOAD_REPORT_URI
を定義してください。
(3) iframe の操作
const frame = page.frameLocator('iframe[title="レポートビューアー"]');
await frame.locator('div#report-main').click();
Salesforce のレポートは iframe
内にあるため、frameLocator()
を使って iframe
の中の要素を操作します。
(4) CSV エクスポート
const element = await frame.locator('xpath=//div[contains(@class, "action-bars")]//button').nth(-1);
await element.click();
await frame.getByRole('menuitem', { name: 'エクスポート' }).click();
エクスポートボタンをクリックし、CSV の出力オプションを選択します。エクスポートボタンはレポートのアクションバーの一番右側のボタンの中に隠れているので、 nth(-1)
で最右ボタンを押してから、クリックしています。
(5) ファイルのダウンロード
const [download] = await Promise.all([
page.waitForEvent('download'),
page.getByRole('button', { name: 'エクスポート' }).click(),
]);
await download.saveAs(filePath);
page.waitForEvent('download')
を使用して、ダウンロードが完了するまで待機します。
ファイルダウンロードダイアログはiframe上ではなく、page上にレンダリングされるため、 page.*
で要素を操作していることに注意してください。
5. 実行方法
-
Playwright をインストール
npm install @playwright/test
-
環境変数を設定
.env
ファイルを作成し、Salesforce のログイン情報を保存します。SALESFORCE_LOGIN_URI=your_login_url SALESFORCE_USERNAME=your_username SALESFORCE_PASSWORD=your_password SALESFORCE_DOWNLOAD_REPORT_URI=your_report_url
-
テストを実行
npx playwright test salesforce-download.spec.js
6. まとめ
Salesforce のレポートを自動的にダウンロードする方法を紹介しました。
この方法を活用して、Salesforce のデータ取得を簡単に自動化してみてください!
CI/CDの自動テストに組み込むととても強力です!