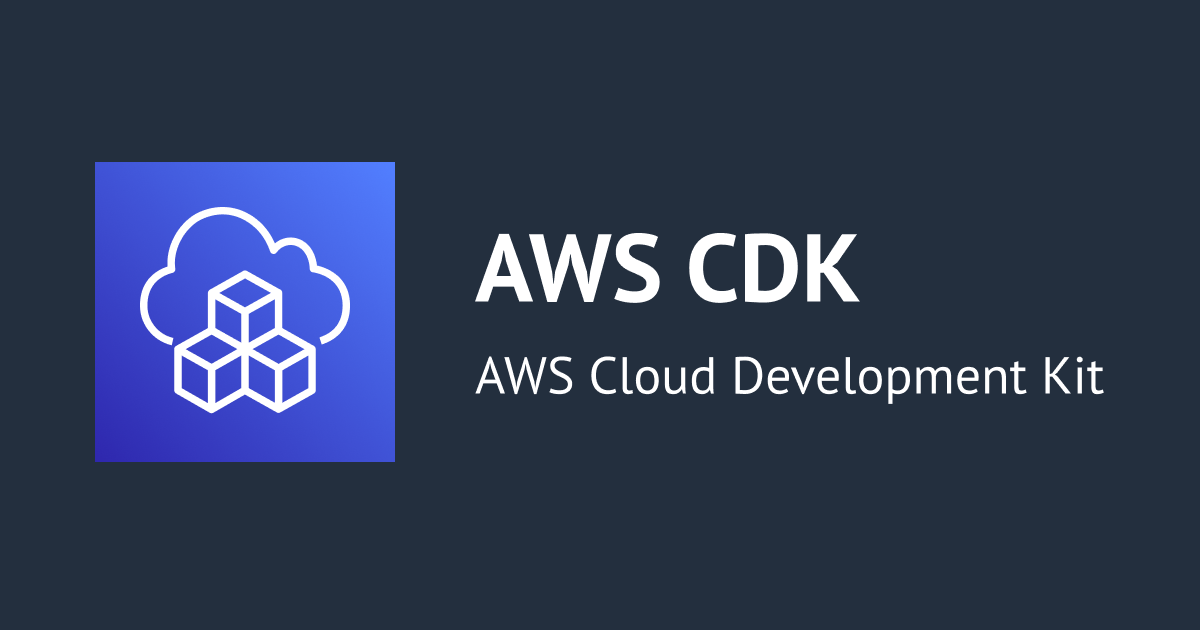
Simplifying Infrastructure as Code with AWS CDK
In my recent project, I came across AWS Cloud Development Kit (CDK) and started to learn it from basics. I started exploring various articles and thought of writing a summarised blog on CDK. I got to know that AWS CDK offers a powerful solution for deploying infrastructure as code (IaC) using familiar programming languages. In this hands-on blog, we will explore the key concepts of AWS CDK and guide you through building a sample application using CDK.
What is AWS CDK?
AWS CDK is an open-source software development framework that allows developers to define cloud infrastructure resources using popular programming languages such as TypeScript, Python, Java, and more. Unlike traditional IaC tools that use static configuration files, CDK enables developers to use the full power of their preferred programming language to define and deploy cloud infrastructure.
Key Benefits of AWS CDK:
1. The CDK provides developers with familiarity with programming and flexibility in defining their infrastructure by choosing from a wide range of supported programming languages. In this way, it is possible to speed up the development cycle and reuse code.
2. CDK treats infrastructure as code, enabling developers to use the same software engineering practices, including version control, unit testing, and integrated development environments. As a result infrastructure code becomes more reliable and maintainable.
3. Resources in AWS CloudFormation are abstracted by CDK's AWS Construct Libraries. These constructs allow developers to define resources using a higher-level, more intuitive API, reducing the complexity and verbosity of infrastructure code.
Building a Serverless Application with AWS CDK:
In this hands-on section, we will build a serverless application with AWS Lambda and Amazon DynamoDB.
Step 1:
Create a new directory for your CDK project and navigate into it. Initialise a new CDK project using the following command:
cdk init app --language python
Step 2:
Install the required AWS CDK packages by running the following command:
pip install aws-cdk.core
Step 3:
Open the generated lib folder and modify the lib/my-cdk-stack.py file. Replace the existing code with the following python code:
import aws_cdk.core as cdk import aws_cdk.aws_lambda as _lambda import aws_cdk.aws_dynamodb as dynamodb import aws_cdk.cloud_assembly_schema as cas class MyCdkStack(cdk.Stack): def __init__(self, scope: cdk.Construct, id: str, **kwargs) -> None: super().__init__(scope, id, **kwargs) # Create an AWS Lambda function function = _lambda.Function(self, 'MyFunction', runtime=_lambda.Runtime.PYTHON_3_9, handler='lambda_function.lambda_handler', code=_lambda.Code.from_asset('my-cdk/lambda_function.zip')) # Create a DynamoDB table table = dynamodb.Table(self, 'MyTable', partition_key=dynamodb.Attribute( name='id', type=dynamodb.AttributeType.STRING), billing_mode=dynamodb.BillingMode.PAY_PER_REQUEST) # Output the Lambda function ARN and DynamoDB table name cdk.CfnOutput(self, 'FunctionARN', value=function.function_arn) cdk.CfnOutput(self, 'TableName', value=table.table_name)
To run the commands in Python, you'll need to have the AWS CDK for Python (aws-cdk-lib) installed. You can install it using pip:
pip install aws-cdk-lib
To zip your lambda_function file, you can run the following command in the directory where lambda_function.py is present.
zip lambda_function.zip lambda_function.py
You can write your code in the lambda_function file. For simplicity, I wrote the following code,
def lambda_handler(event, context): # Your Lambda function logic here return "Hello from Lambda!"
Step 4:
Build your CDK Python code:
cdk bootstrap
cdk synth
Deploy the infrastructure to your AWS account using the following command:
cdk deploy
Step 5:
Once the deployment is complete, you can view your lambda function and dynamoDB table getting created. You are free to experiment with your CDK now.
With CDK, you can take advantage of the rich ecosystem of programming languages and software engineering practices to create and manage your AWS resources more effectively. So go ahead and give AWS CDK a try.
Thank you!
Happy Learning :)