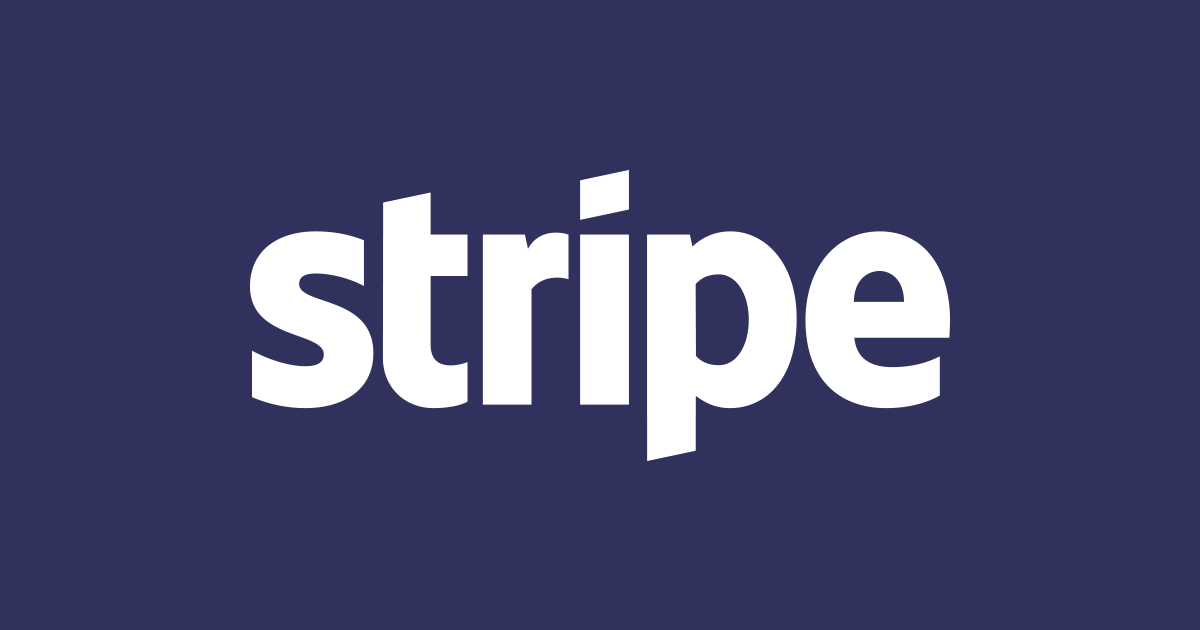
Stripe의 Web Element의 Mode Option을 이용해 결제 양식을 React에서 만들어 보았다.
Stripe의 결제 양식을 만들 때 Quick start와 같은 문서를 보면 요구되는 client secret 없이 결제 양식을 만들어 보았습니다.
준비
먼저 아래의 링크에서 Stripe의 계정을 만들어 주세요 Stripe
다음으로 프로젝트를 생성해 줍니다.
npm create vite@latest [project-name] -- --template react-ts
이후 필요한 라이브러리를 받아주세요.
npm install --save @stripe/react-stripe-js @stripe/stripe-js
이후 생성한 Stripe 계정에서 개발키를 복사 후 프로젝트에 넣어주시면 끝입니다.
문제없이 실행되었다면 간단하게 결제 양식을 만들어 보겠습니다.
결제 양식 생성
결제 양식을 만들어 줍니다.
import { PaymentElement } from "@stripe/react-stripe-js"; import { StripePaymentElementOptions } from "@stripe/stripe-js"; function CheckoutForm() { const options: StripePaymentElementOptions = { layout: { type: "tabs", }, }; return ( <form> <PaymentElement options={options} /> <button type="submit">SUBMIT</button> </form> ); } ...
이후 React의 App.tsx 파일에 아래의 코드를 적어주세요. 여기서 Quick start 다른 점은 options에 mode를 설정하는 것 뿐입니다.
import { PaymentElement } from "@stripe/react-stripe-js"; import { StripePaymentElementOptions } from "@stripe/stripe-js"; const stripePromise = loadStripe(your stripe publishable key); function App() { const options: StripeElementsOptions = { mode: "payment", amount: 100, currency: "usd", locale: "en", }; return ( <Elements options={options} stripe={stripePromise}> <CheckoutForm /> </Elements> ); } ...
이상 Stripe의 Web Elements의 Mode Options를 이용해서 결제 양식을 만들어 보았습니다.
이외에도 카드저장 양식 등을 만들 때는 아래와 같은 지정으로 쉽게 양식을 만들 수 있습니다.
const options: StripeElementsOptions = { mode: "setup", currency: "usd", locale: "en", };
보충
Stripe Element를 이용하다 보면 아래와 같이 표시하고 싶지 않은 영역이 있습니다.
이 영역을 없애고 싶은 경우에는 아래와 같은 지정으로 간단히 표시에서 제외할 수 있습니다.
const options: StripePaymentElementOptions = { layout: { type: "tabs", }, defaultValues: { billingDetails: { address: { country: "KR", }, }, }, fields: { billingDetails: { address: { country: "never", }, }, }, terms: { card: "never", }, };