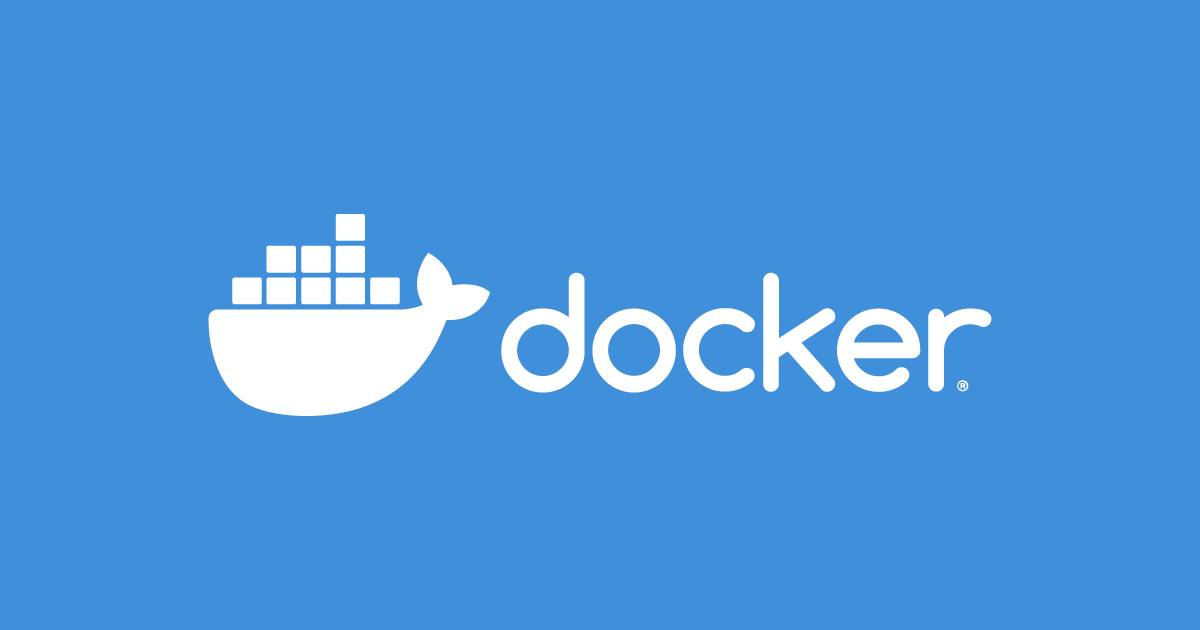
TypeORM をサクッと試せる Docker 環境を TypeORM CLI を使って構築する方法
TypeORM には CLI が用意されており、typeorm init
コマンドを用いて新規プロジェクトをサクッと作ることができます。
その typeorm init
のオプションで --docker
を渡すことで docker-compose.yml
ファイルも生成してくれます。
簡単ですね。やってみましょう。
やってみた
npx typeorm init --name my-project --docker
上記のコマンドを実行すれば、my-project
というディレクトリが作成され、TypeORM を動かすのに必要なソースコード一式や docker-compose.yml
が生成されます。
今回はオプションでデータベースを指定していないので、デフォルトの PostgreSQL が使われます。
また上記のコマンドで以下の内容が記載された README.md
が生成されます。
# Awesome Project Build with TypeORM Steps to run this project: 1. Run `npm i` command 2. Run `docker-compose up` command 3. Run `npm start` command
npm install
するように書いてありますが、実は typeorm init
コマンドを実行することで自動的に npm install
も実行されるので改めて実行する必要はなかったりします。(ちなみにオプション次第で yarn を使うこともできます)
というわけで次に PostgreSQL を Docker で動かします。
docker compose up
Docker が起動しましたら、次に TypeORM を使っているプログラムを実行します。
npm start
以下の出力がされていれば成功です。
Inserting a new user into the database... Saved a new user with id: 1 Loading users from the database... Loaded users: [ User { id: 1, firstName: 'Timber', lastName: 'Saw', age: 25 } ] Here you can setup and run express / fastify / any other framework.
このプログラムは src/index.ts
に記述されていますので、TypeORM を試したり、TypeORM の使い方を学んだりするにはそちらのファイルを変更するといいでしょう。
実案件では試しにくい SQL インジェクションも ローカルの Docker で試す分には安心ですね。
おまけ: TypeORM CLI で express + TypeORM の環境を作る
--express
オプションを付与すれば Web アプリケーションフレームワークの express を使用したプロジェクトを作成できます。
もちろん --docker
オプションと併用できます。
npx typeorm init --name my-project2 --docker --express cd my-project2 docker compose up
ただしこちらはあまり積極的にメンテナンスされていないのか、このまま npm start
して案内される http://localhost:3000/users
にアクセスしても例外が吐かれました。
Express server has started on port 3000. Open http://localhost:3000/users to see results ConnectionNotFoundError: Connection "default" was not found.
そうなった場合は src/controller/UserController.ts
を以下のように userRepository
の取得方法を変更してみてください。
import { getRepository } from 'typeorm' import { NextFunction, Request, Response } from 'express' import { User } from '../entity/User' import { AppDataSource } from '../data-source' export class UserController { private userRepository = AppDataSource.getRepository(User) async all(request: Request, response: Response, next: NextFunction) { return this.userRepository.find() } async one(request: Request, response: Response, next: NextFunction) { return this.userRepository.findOne(request.params.id) } async save(request: Request, response: Response, next: NextFunction) { return this.userRepository.save(request.body) } async remove(request: Request, response: Response, next: NextFunction) { let userToRemove = await this.userRepository.findOneBy({ id: request.params.id, }) await this.userRepository.remove(userToRemove) } }
変更後に再度 npm start
を実行し、URL にアクセスすればユーザー一覧が見えるようになります。