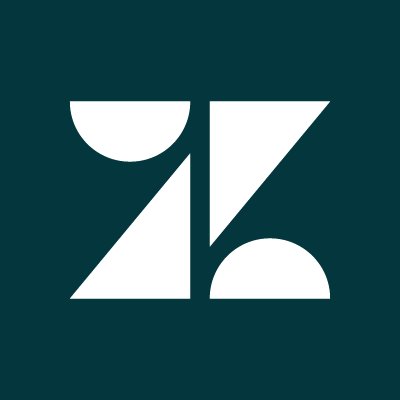
Check the Zendesk’s ticket using Python
In the previous post, we got ticket data and comment data using Zendesk API.
In this post, we introduce that get zendesk's ticket and comment data using Python. ※We used Python version 3.6.1.
Let's install the requests
module if you don't have it.
$ pip3 install requests
Get ticket data
Get the following ticket data
- id: Automatically assigned when the ticket is created
- subject: The value of the subject field for this ticket
- created_at: When this record was created
- updated_at: When this record last got updated
Sample code
import requests from urllib.parse import urlencode import time # Set the request parameters url = 'https://subdomain.zendesk.com/api/v2/search.json?' user = 'email address' + '/token' pwd = 'api key' session = requests.Session() session.auth = (user, pwd) params = {'query': 'type:ticket status:closed brand:{brand_id}'} url = url + urlencode(params) ticket_results = [] while url: response = session.get(url) if response.status_code == 429: print('Rate limited! Please wait.') time.sleep(int(response.headers['retry-after'])) continue if response.status_code != 200: print('Status:', response.status_code, 'Problem with the request. Exiting.') exit() data = response.json() ticket_results.extend(data['results']) url = data['next_page'] for ticket in ticket_results: ticket_id = ticket['id'] created_at = ticket['created_at'] updated_at = ticket['updated_at'] subject = ticket['subject'] print(f'ticket_id: {ticket_id}, ' f'create_at: {created_at}, ' f'updata_at: {updated_at}, ' f'subject: {subject}')
We got ticket data with a specific brand status is "closed".
params = {'query': 'type:ticket status:closed brand:{brand_id}'}
search.json
can only return a maximum of 100 records per page so we got all data using next_page
.
while url: [...] url = data['next_page']
We continue to get after retry-after
time if we reach the API Rate limited.
if response.status_code == 429: print('Rate limited! Please wait.') time.sleep(int(response.headers['retry-after'])) continue
Example Response
ticket_id: ****4, create_at: 2017-06-02T00:36:35Z, updata_at: 2017-06-12T17:02:09Z, subject: test ticket_id: ****5, create_at: 2017-06-01T19:12:09Z, updata_at: 2017-06-05T21:01:49Z, subject: test2 ticket_id: ****6, create_at: 2017-06-01T18:56:38Z, updata_at: 2017-06-05T20:03:50Z, subject: test3 ticket_id: ****7, create_at: 2017-05-31T14:58:46Z, updata_at: 2017-06-04T18:02:43Z, subject: test4
Get comment data
We could get ticket id so get comment data from comments.json
.
※comments.json
specifies the ticket id and use it.
ticket_id = '1'
Get the following comment data
- id: Automatically assigned when the comment is created
- public: true if a public comment; false if an internal note.
- body: The comment string
Sample code
import requests # Set the request parameters url = 'https://subdomain.zendesk.com/api/v2/tickets/' user = 'email_address' + '/token' pwd = 'api key' session = requests.Session() session.auth = (user, pwd) ticket_id = '1' url = url + ticket_id + "/comments.json" response = session.get(url) if response.status_code != 200: print('Status:', response.status_code, 'ticket_id:', ticket_id, 'Problem with the request. Exiting.') exit() data = response.json() for comment in data['comments']: comment_id = comment['id'] public = comment['public'] body = comment['body'] print(f'comment_id: {comment_id}, ' f'public: {public}, ' f'body: {body}')
Example Response
comment_id: 4***********, public: True, body: test comment comment_id: 4***********, public: False, body: private comment comment_id: 4***********, public: True, body: public test comment comment_id: 4***********, public: True, body: test2 comment comment_id: 4***********, public: True, body: closed comment
Conclusion
This post introduced how to get zendesk data using python.