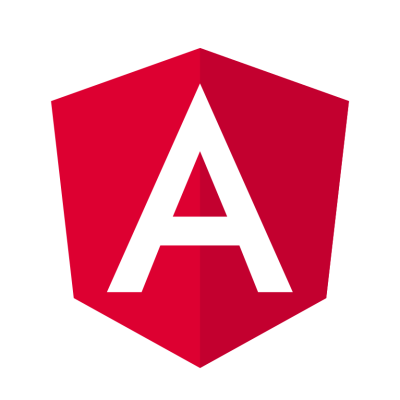
Angular用の都道府県マスタを作成
Angular用の都道府県マスタです。ご自由にお使いください。
はじめに
Angular用の都道府県マスタを作成したので公開しておこうと思います。
環境
Angular CLI: 6.1.3 Node: 8.11.3 OS: darwin x64 Angular: 6.1.2 ... common, compiler, compiler-cli, core, forms, http ... language-service, platform-browser, platform-browser-dynamic ... router Package Version ----------------------------------------------------------- @angular-devkit/architect 0.7.3 @angular-devkit/build-angular 0.7.3 @angular-devkit/build-optimizer 0.7.3 @angular-devkit/build-webpack 0.7.3 @angular-devkit/core 0.7.3 @angular-devkit/schematics 0.7.3 @angular/animations 6.1.0 @angular/cdk 6.4.3 @angular/cli 6.1.3 @angular/material 6.4.3 @ngtools/webpack 6.1.3 @schematics/angular 0.7.3 @schematics/update 0.7.3 rxjs 6.2.2 typescript 2.7.2 webpack 4.9.2
マスタを作成
総務省が定めている都道府県コードの通りにマスタを作成しました。
value
に都道府県コード、 viewValue
に実際に画面に表示される都道府県名を定義しておきます。
src/app/util/prefectures.ts
export interface State { value: number; viewValue: string; } export const SelectPrefectures = { states: [ {value: '01', viewValue: '北海道'}, {value: '02', viewValue: '青森県'}, {value: '03', viewValue: '岩手県'}, {value: '04', viewValue: '宮城県'}, {value: '05', viewValue: '秋田県'}, {value: '06', viewValue: '山形県'}, {value: '07', viewValue: '福島県'}, {value: '08', viewValue: '茨城県'}, {value: '09', viewValue: '栃木県'}, {value: '10', viewValue: '群馬県'}, {value: '11', viewValue: '埼玉県'}, {value: '12', viewValue: '千葉県'}, {value: '13', viewValue: '東京都'}, {value: '14', viewValue: '神奈川県'}, {value: '15', viewValue: '新潟県'}, {value: '16', viewValue: '富山県'}, {value: '17', viewValue: '石川県'}, {value: '18', viewValue: '福井県'}, {value: '19', viewValue: '山梨県'}, {value: '20', viewValue: '長野県'}, {value: '21', viewValue: '岐阜県'}, {value: '22', viewValue: '静岡県'}, {value: '23', viewValue: '愛知県'}, {value: '24', viewValue: '三重県'}, {value: '25', viewValue: '滋賀県'}, {value: '26', viewValue: '京都府'}, {value: '27', viewValue: '大阪府'}, {value: '28', viewValue: '兵庫県'}, {value: '29', viewValue: '奈良県'}, {value: '30', viewValue: '和歌山県'}, {value: '31', viewValue: '鳥取県'}, {value: '32', viewValue: '島根県'}, {value: '33', viewValue: '岡山県'}, {value: '34', viewValue: '広島県'}, {value: '35', viewValue: '山口県'}, {value: '36', viewValue: '徳島県'}, {value: '37', viewValue: '香川県'}, {value: '38', viewValue: '愛媛県'}, {value: '39', viewValue: '高知県'}, {value: '40', viewValue: '福岡県'}, {value: '41', viewValue: '佐賀県'}, {value: '42', viewValue: '長崎県'}, {value: '43', viewValue: '熊本県'}, {value: '44', viewValue: '大分県'}, {value: '45', viewValue: '宮崎県'}, {value: '46', viewValue: '鹿児島県'}, {value: '47', viewValue: '沖縄県'} ] };
入力フォームを作成(画面)
HTML
src/app/component/home-address.component.html
<br /><!-- address form group --> <form>住所入力フォーム <!-- postal code --> <input id="update-postal_code" maxlength="7" required="" type="text" placeholder="郵便番号" /> {{postalCode.value.length}} / 7 ※半角数字のみ入力できます <!-- state --> 都道府県 {{state.viewValue}} <!-- city --> <input id="update-city" required="" type="text" placeholder="市区町村" /> <!-- block --> <input id="update-block" required="" type="text" placeholder="番地" /> ※半角数字と-(ハイフン)のみ入力できます <!-- etc --> <input id="update-address_etc" type="text" placeholder="マンション名・部屋番号" /> </form>
Typescript
src/app/component/home-address.component.ts
import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, Validators, FormControl, FormGroupDirective, NgForm } from '@angular/forms'; import { SelectPrefectures } from './../../util/prefectures'; // 上で作成した都道府県マスタ @Component({ selector: 'app-home-address', templateUrl: './home-address.component.html', styleUrls: ['./home-address.component.css'] }) export class ChangeUserInfoComponent implements OnInit { addressForm: FormGroup; constructor( private fb: FormBuilder, private router: Router ) {} ngOnInit() { this.initForm(); } // 入力フォーム初期化 initForm() { this.addressForm = this.fb.group({ postal_code: new FormControl( '', Validators.compose([ Validators.required, Validators.minLength(7) ]) ), state: ['', Validators.required], city: ['', Validators.required], block: ['', Validators.required], address_etc: [''] }); } }