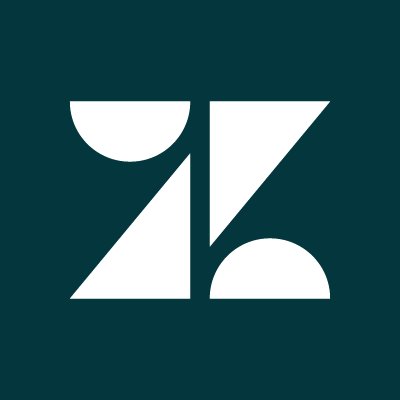
Check the tickets using Zendesk API
In this post, we introduce how to check tickets, comments and open tickets etc. using Zendesk's API.
The link below explains how to create API Tokens.
Creating and managing API tokens.
We set API tokens after creating API tokens.
email_address='************' password='*****************' url='https://subdomain.zendesk.com/api/v2/'
Tickets
GET /api/v2/tickets.json
Get a list of tickets.
$ curl ${url}tickets.json -u ${email_address}/token:${password} | jq .
A maximum of 100 tickets will be displayed. Tickets are ordered by date created, from the oldest. Archived tickets are not displayed.
An example of a response
{ "tickets": [ { "url": "https://subdomain.zendesk.com/api/v2/tickets/{ticket_id}.json", "id": {ticket_id}, "external_id": null, [...] "created_at": "yyyy-MM-ddThh:mm:ssZ", "updated_at": "yyyy-MM-ddThh:mm:ssZ", "type": null, "subject": "{sample_subject}", "raw_subject": "{sample_raw_subject}", "description": "{sample_comment}", [...] ], "next_page": "https://subdomain.zendesk.com/api/v2/tickets.json?page=2", "previous_page": null, "count":**** }
GET /api/v2/incremental/tickets.json?start_time={unix_time}
In the previous case GET /api/v2/tickets.json
, we could not check the all tickets. We re-check by specifying start_time={unix_time}
.
We will look for tickets from March 1, 2015.
$ curl ${url}incremental/tickets.json?start_time=1425135600 -u ${email_address}/token:${password} | jq .
This response returns up to 1000 items per page. Items can be checked sequentially using the next_page
URL.
Example Response
{ "tickets": [ { "url": "https://subdomain.zendesk.com/api/v2/tickets/{ticket_id}.json", "id": {ticket_id}, "external_id": null, [...] "created_at": "2015-03-27T01:32:49Z", "updated_at": "2015-03-31T09:01:09Z", "type": "incident", "subject": "{sample_ subject}", "raw_subject": "{raw_subject}", "description": "{sample_comment}", "priority": "high", "status": "closed", [...] "count": 1000, "next_page": "https://subdomain.zendesk.com/api/v2/incremental/tickets.json?start_time=1460707286", "end_time": 1460707286 }
We can get upto 1000 tickets, starting from the oldest ticket.
Ticket Comments
GET /api/v2/tickets/{ticket_id}/comments.json
To get the comment for a ticket, specify the ticket ID.
$ curl ${url}requests/{ticket_id}/comments.json -u ${email_address}/token:${password} | jq .
Example Response
{ "comments": [ { "url": "https://subdomain.zendesk.com/api/v2/requests/{ticket_id}/comments/{comment_id}.json", "id": {comment_id}, "type": "Comment", "request_id": {ticket_id}, "body": "test comment", "html_body": "<div class=\"zd-comment\"><p dir=\"auto\">test comment</p></div>", "plain_body": "test comment", "public": true, "author_id": {author_id}, "attachments": [], "created_at": "2017-11-18T22:28:30Z" }, { "url": "https://subdomain.zendesk.com/api/v2/requests/{ticket_id}/comments/{comment_id}.json", "id": {comment_id}, "type": "Comment", "request_id": {ticket_id}, "body": "public test comment", "html_body": "<div class=\"zd-comment\">public test comment<br>\n</div>", "plain_body": "public test comment", "public": true, "author_id": {author_id}, "attachments": [], "created_at": "2017-11-18T22:28:59Z" }, { "url": "https://subdomain.zendesk.com/api/v2/requests/{ticket_id}/comments/{comment_id}.json", "id": {comment_id}, "type": "Comment", "request_id": {ticket_id}, "body": "test2 comment", "html_body": "<div class=\"zd-comment\"><p dir=\"auto\">test2 comment</p></div>", "plain_body": "test2 comment", "public": true, "author_id": {author_id}, "attachments": [], "created_at": "2017-11-18T22:29:13Z" }, { "url": "https://subdomain.zendesk.com/api/v2/requests/{ticket_id}/comments/{comment_id}.json", "id": {comment_id}, "type": "Comment", "request_id": {ticket_id}, "body": "closed comment", "html_body": "<div class=\"zd-comment\">closed comment<br>\n</div>", "plain_body": "closed comment", "public": true, "author_id":{author_id}, "attachments": [], "created_at": "2017-11-18T22:29:55Z" } ], [...].
Search
Zendesk developers - Core API:Search
Use a search query to check a specific tickets.
.../api/v2/search.json?query={search_string}
query=type:ticket status<closed
This query can check tickets that are not closed.
$ curl ${url}search.json -G --data-urlencode "query=type:ticket status<closed" -u ${email_address}/token:${password} | jq .
Example Response
{ "results": [ { "url": "https://subdomain.zendesk.com/api/v2/tickets/{ticket_id}.json", "id": {ticket_id}, "external_id": null, [...] "created_at": "yyyy-MM-ddThh:mm:ssZ", "updated_at": "yyyy-MM-ddThh:mm:ssZ", "type": null, "subject": "{subject}", "raw_subject": "{raw_subject}", "description": "{description}", "priority": "low", "status": "new", [...] "facets": null, "next_page": "https://subdomain.zendesk.com/api/v2/search.json?page=2&query=type%3Aticket+status%3Cclosed", "previous_page": null, "count": {count} }
query=type:ticket status:closed brand:{brand_id}
This query can look for tickets that are closed and specify the brand. We can also check how many closed tickets there are using the command count
.
$ curl ${url}search.json -G --data-urlencode "query=type:ticket status:closed brand:{brand_id}" -v -u ${email_address}/token:${password} | jq .
[...] "next_page": "https://subdomain.zendesk.com/api/v2/search.json?page=2&query=type%3Aticket+status%3Cclosed", "previous_page": null, "count": {count} }
query=type:ticket brand:{brand_id} created>2017-10-01T00:00:00Z created<2017-11-01T00:00:00Z
This query looks up tickets that were created in October, with the brand specified.
curl ${url}search.json -G --data-urlencode "query=type:ticket brand:{brand_id} created>2017-10-01T00:00:00Z created<2017-11-01T00:00:00Z" -v -u ${email_address}/token:${password} | jq .
For search, please refer to the Zendesk Support search referenc
Conclusion
This post was an introduction to checking tickets using Zendesk's API. It is also possible to obtain necessary information using jq
.