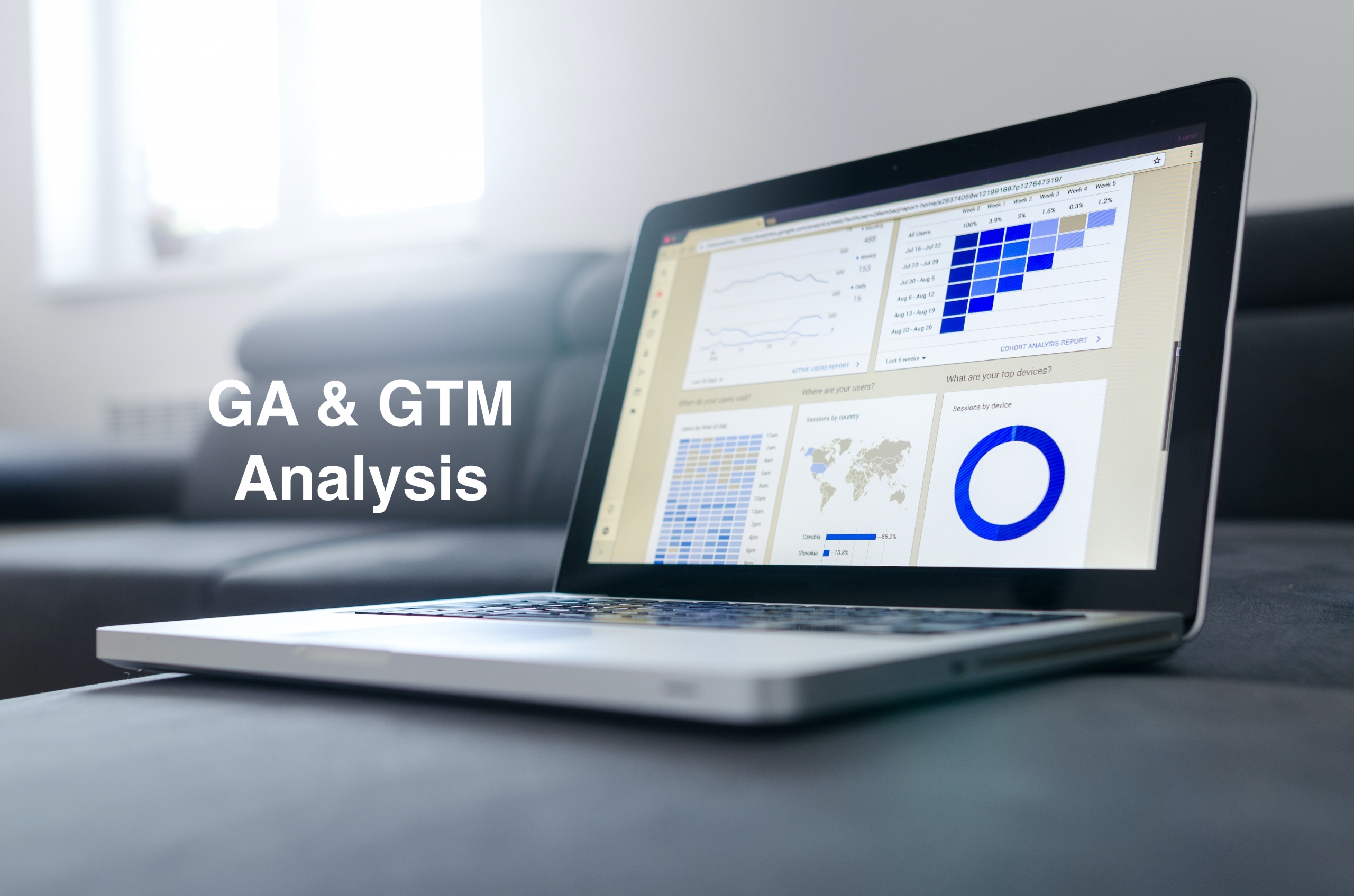
Google Analytics and Google Tag Manager setup into React Project
Introduction
Nowadays most web applications add Google Analytics(GA) as a feature that helps us identify the trends and patterns on how the user engages with the website. Years ago, the process of connecting GA with the web applications took most of the developer's bandwidth since everything (code changes, setup, and configuration) had to be done by their end, like from collecting the data and sending it to GA. To reduce the workload on the developer, Google introduced Google Tag Manager (GTM) in 2012 as a bridge to connect our application with GA by using a simple tag management system, where its configuration and setup are easy.
If simply to define GTM: Google Tag Manager is a free tracking tool and management platform that allows the user to add marketing tags, or snippets of code, to your website to track and collect marketing data. GTM allows users to easily implement tracking tags without modifying the code while improving the information gathering process.
Some people get confused about whether to use GTM or GA in their application. But the answer is “we should use both”.
When GA is alone, developers had to hard code the GA tracking codes on each page. Let’s say the user has to handle hundreds or even thousands of events in their application. Then it becomes the biggest task to maintain and update them rather than developing the website. But with GTM, we can simply use the tags provided by GTM in our application without modifying the code of our application.
The feature that comes with GTM are as follows:
GA Setup
Step 1: Create an account
Get on to Google Analytics, click on the admin icon present at the bottom-left corner of the screen. One shall be directed to the admin setting overview page. First and foremost, create an account, then give your account an appropriate name, and click next.
Set an account name for your project:
Next, you will be prompted to add a property, A property indicates a business’s web or app data. One account can have one or more properties. Next, fill in the reporting time zone and corresponding currency of the country, this data is useful in displaying the analysis reports and revenue-related data. If required this data can be modified later by the admin.
Next, one shall be moved to the business information section, add the relevant data as per the criteria of usage.
Now accept the Google Analytics Terms of Service Agreement and proceed. Next, we should set up the data stream
Step 2: Set up a data stream
Data-stream represents the platform from which we collect data. We can add multiple data streams to a property such as a stream from an Android app, one from the iOS app, and one from the website. This feature allows us to view the data from multiple streams in the reports.
Now, choose the platform, for me, it's a web app.
Then fill in the URL and Stream name for your target website. then click on create a stream.
Once we set up a data stream, we shall get tagging information and a Measurement ID for web streams.
GTM Setup
Create an account and a container
Once you are on the GTM page click on create an account button. Fill in the account name, generally, it's recommended to have one account name per company. Next comes a container, Container is the entity that holds all the tags, triggers, and variables, which helps in collecting the data and allowing it to pass to the other applications seamlessly for example Google Analytics or Google ads. Next, choose the target platform of the application and then click on create.
On creation we have a pop-up for the Google Tag Manager Terms of Service Agreement, please say yes.
Wait and we will see a screen that will pop up with the code snippet That needs to be implemented on the page. Please implement the code as described.
By chance, you miss the popup of the code snippet you can just click on the container ID on the header bar for the code snippet
*All the accounts created above are for demo purposes only. Thats why measurement IDs and tracking IDs are not blurred.
React Setup
Code Implementation
Now to implement the code into the application. We will embed generated script by GTM into the header section of index.html
in React project.
index.html
<!DOCTYPE html> <html lang="en"> <head> <!-- ...meta tags and styles are omitted --> <!-- Google Tag Manager --> <script> (function (w, d, s, l, i) { w[l] = w[l] || []; w[l].push({"gtm.start": new Date().getTime(), event: "gtm.js"}); var f = d.getElementsByTagName(s)[0], j = d.createElement(s), dl = l != "dataLayer" ? "&l=" + l : ""; j.async = true; j.src = "https://www.googletagmanager.com/gtm.js?id=" + i + dl; f.parentNode.insertBefore(j, f); })( window, document, "script", "dataLayer", "%REACT_APP_GA_TAG_MANAGER_ID%", ); </script> <!-- End Google Tag Manager --> </head> <body> <!-- Google Tag Manager (noscript) --> <noscript ><iframe src="https://www.googletagmanager.com/ns.html?id=%REACT_APP_GA_TAG_MANAGER_ID%" height="0" width="0" style="display:none;visibility:hidden" ></iframe ></noscript> <!-- End Google Tag Manager (noscript) --> <div id="root"></div> </body> </html>
It is often a better idea of not hard code GA/GTM tags. Instead, we can get value from .env file. Please refer to this blog as well.
Validation
To verify the set-up of GTM, run a simple test in dev tools. Open up dev tools and look for gtm.js under the network. On the right set-up, it shows up 200. If you see a 404 error code, double-check your GTM ID. Also, make sure that you have published the container at least once.