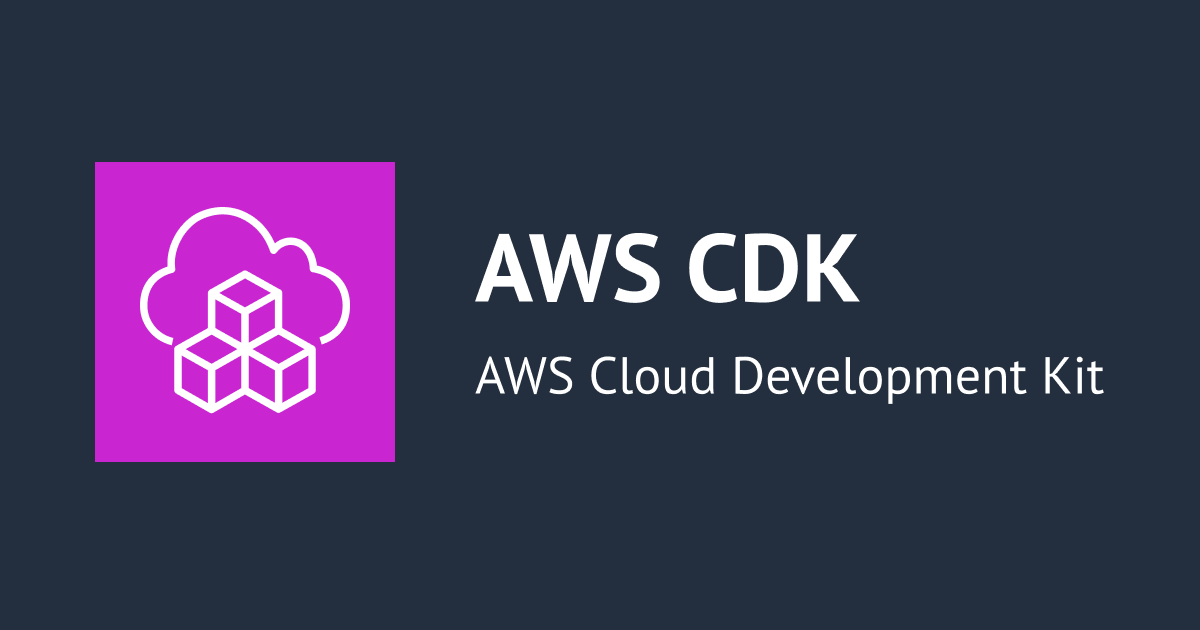
How to set up lifecycle rules for an S3 bucket using the CDK
Hi, this is Charu from Classmethod. In this blog post, we will guide you through setting up lifecycle rules for an S3 bucket using the aws-cdk-lib.
The AWS Cloud Development Kit (CDK) is a powerful tool that simplifies the definition, deployment, and management of cloud infrastructure through familiar programming languages. One of the advanced features you can manage through the CDK is the lifecycle rules of S3 buckets, which are crucial for optimizing storage costs and ensuring data is managed according to your organization's retention policies.
Let's get started!
Step 1:
First, create a directory for your project and navigate into it:
mkdir s3-lifecycle-cdk
cd s3-lifecycle-cdk
Initialize a CDK project using TypeScript (you can choose another language if you prefer):
cdk init app --language typescript
This command creates a new CDK app with a sample stack.
Step 2:
Add the S3 Module To work with S3, you need to add the AWS S3 module to your project. Run:
npm install @aws-cdk/aws-s3
Step 3:
Define the S3 Bucket with Lifecycle Rules
Open the main stack file (For example, lib/s3-lifecycle-cdk-stack.ts) and import the S3 package at the top:
import aws_s3 from '@aws-cdk/aws-s3';
Then, define an S3 bucket with lifecycle rules within the stack constructor. For example to delete the objects from the bucket after 7 days, you can add the following code:
const myLifecycleManagedBucket = new aws_s3.Bucket(this, "MyLifecycleManagedBucket", { removalPolicy: RemovalPolicy.DESTROY, }); myLifecycleManagedBucket.addLifecycleRule({ enabled: true, expiration: Duration.days(7), });
In this snippet:
The addLifecycleRule defines a set of rules for managing the lifecycle of objects within the bucket.
Step 4:
Deploy Your CDK Stack After defining your stack, it's time to deploy it. First, compile your TypeScript project and then, deploy your CDK stack to your AWS account:
npm run build
cdk deploy
Step 5:
Verification After deployment, you can verify the lifecycle rules are set up correctly by checking the S3 bucket's properties in the AWS Management Console.
Bonus:
Here is one more example for you to try,
// Create the S3 bucket const myLifecycleManagedBucket = new aws_s3.Bucket(this, "MyLifecycleManagedBucket", { removalPolicy: RemovalPolicy.DESTROY }); // Add a lifecycle rule for transitioning to Glacier after 90 days myLifecycleManagedBucket.addLifecycleRule({ id: 'TransitionToGlacier', transitions: [{ storageClass: aws_s3.StorageClass.GLACIER, transitionAfter: Duration.days(90), }], }); // Add a separate lifecycle rule for expiration after 365 days myLifecycleManagedBucket.addLifecycleRule({ id: 'ExpireAfter365Days', expiration: Duration.days(365), }); } }
In the above snippet, transitions specifies the conditions under which objects should be moved to a different storage class. In this case, objects are moved to Glacier after 90 days. While, expiration sets the lifetime of the objects in the bucket. Here, objects are deleted 365 days after being created. Each rule can specify transitions (e.g., moving objects to a different storage class) and an expiration action id gives a unique identifier for the lifecycle rule.
Conclusion:
Using the AWS CDK to manage your S3 buckets and their lifecycle rules can greatly simplify and automate the process, ensuring your data is stored efficiently and cost-effectively according to your policies.
Thank you for reading!
Happy Learning:)