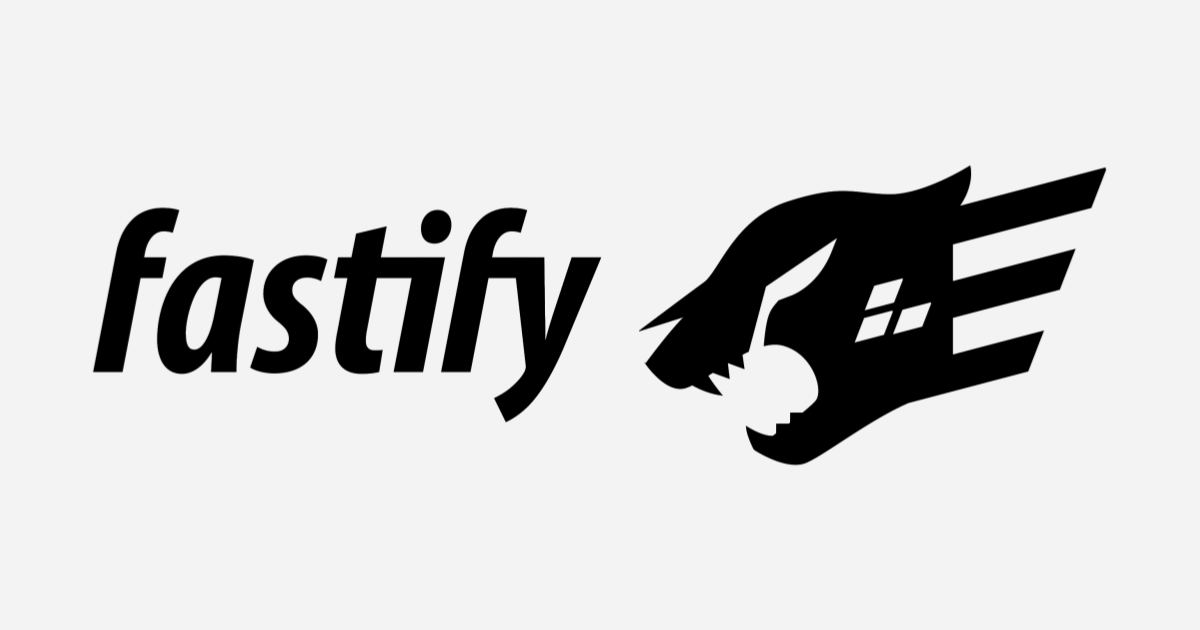
I solved ‘Property ‘get’ does not exist on type ‘FastifyInstance<Server<typeof IncomingMessage, typeof ServerResponse>, IncomingMessage, ServerResponse<IncomingMessage>, FastifyBaseLogger, FastifyTypeProviderDefault> & PromiseLike<...>” error
Hi, this is Charu from Classmethod. In this short blog, we will be solving the Property 'get' does not exist on type 'FastifyInstance
error.
Before moving on, I will show you the simple code which I wrote,
source.ts
import { fastify, FastifyReply, FastifyRequest } from "fastify"; const server = fastify({ logger: true }); server.get("/", async (request: FastifyRequest, reply: FastifyReply) => { return { greeting: "Hello from Fastify and TypeScript!" }; }); const start = async () => { try { await server.listen({ port: 8888 }); } catch (err) { server.log.error(err); process.exit(1); } }; start();
tsconfig.json
{ "compilerOptions": { "target": "es2018", "module": "commonjs", "moduleResolution": "node", "outDir": "./dist", "esModuleInterop": true, "strict": true, "skipLibCheck": true, }, "include": [ "src/**/*" ], "exclude": [ "node_modules" ] }
Everything looks fine and the code works properly. But still, I was getting the error at server.get("/"
statement.
I went through Google and got my solution in the Fastify official document.
My Typescript module was 'CommonJS' while I was importing Fastify according to ESmodules. Hence, I changed the import statement and the error was gone:D
Below is my final code with no errors.
const fastify = require("fastify")({ logger: true }); fastify.get("/", async (request: any, reply: any) => { return { greeting: "Hello from Fastify and TypeScript!" }; }); const start = async () => { try { await fastify.listen({ port: 8888 }); } catch (err) { fastify.log.error(err); process.exit(1); } }; start();
I hope this blog helped you too.
Thank you for reading!
Happy Learning:)