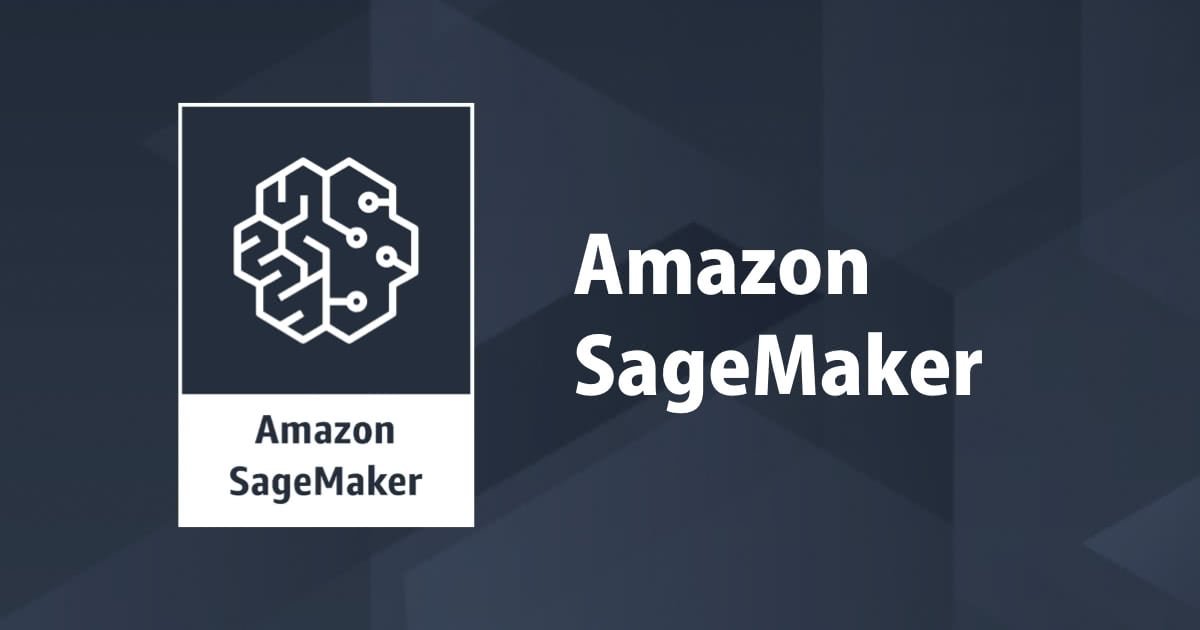
Marketplaceに公開されているアルゴリズムをSageMakerノートブックインスタンスから利用する:Amazon SageMaker Advent Calendar 2018
概要
こんにちは、データインテグレーション部のyoshimです。
この記事は「クラスメソッド Amazon SageMaker Advent Calendar」の17日目の記事となります。
先日のre:inventで発表されたAWS Marketplace for Machine Learningに公開されているアルゴリズムを使って、「トレーニング」や「ハイパーパラメータチューニング」をしてみました。
コンソール画面上からでも同じことはできるのですが、今回は「SageMakerノートブックインスタンス」上から実行しています。
主に、下記のドキュメントを参考にしています。
Amazon SageMaker Resources in AWS Marketplace
Use an Algorithm to Run a Training Job
Use an Algorithm to Run a Hyperparameter Tuning Job
目次
- 1.やること
- 2.「AWS Marketplace for Machine Learning」について
- 3.普通にトレーニングしてみる
- 4.ハイパーパラメータの自動チューニングをしてみる
- 5.備考
- 6.まとめ
1.やること
「AWS Marketplace for Machine Learning」に公開されているアルゴリズムを「実際に使う工程について把握したい」というモチベーションから下記の2点を試してみました。
先日、コンソール画面上から学習させる手順については確認したので、今回は「SageMakerノートブックインスタンス」上から利用する手順についてです。
a.普通にトレーニングする
通常のビルトインアルゴリズムと同様に、「ハイパーパラメータ」や「参照データ」等を指定して学習させてみます。
b.ハイパーパラメータの自動チューニングをしてみる
ハイパーパラメータチューニングの際は、私はよく「ハイパーパラメータの自動チューニング機能」を使っているので、こちらの手順についても確認します。
・今回利用したパッケージ
2.「AWS Marketplace for Machine Learning」について
詳細についてはAmazon SageMaker Resources in AWS Marketplaceをご参照ください。
「AWS Marketplace for Machine Learning」について「概要をとりあえず知りたい」という方は、手前味噌ですが、こちらをご参照ください。
3.普通にトレーニングしてみる
MarketPlaceでサブスクライブしたアルゴリズムを使う場合も、特別な手順はほとんどありません。
まずは「通常のトレーニング」、「ハイパーパラメータチューニングJOB」の両方に必要な変数を指定します。
ここで「アルゴリズムのARN」を指定していることにご注意ください。
import os import sagemaker from sagemaker import get_execution_role bucket = 's3://<your bucket>' # Customize your own bucket name train_prefix = 'marketplace/sklearn_decision_tree/fromsdk/data/training/train.csv' algorithm_arn = <your argorithm arn> data_path = os.path.join(bucket, train_prefix) sess = sagemaker.Session() role = get_execution_role()
続いて、「AlgorithmEstimator」を使って先ほど指定した「アルゴリズムのARN」を利用して学習を実行します。
from sagemaker import AlgorithmEstimator # マーケットプレイスのアルゴリズムで学習する際は、「AlgorithmEstimator」を使います algo = AlgorithmEstimator( algorithm_arn=algorithm_arn, role=role, train_instance_count=1, train_instance_type='ml.c4.xlarge', sagemaker_session=sess, base_job_name='marketplace-sklearn-dt') algo.set_hyperparameters(max_leaf_nodes=10) algo.fit({'training': data_path})
これだけです。
シンプルな記述で実行できます。
4.ハイパーパラメータの自動チューニングをしてみる
続いて、「ハイパーパラメータのチューニングJOB」を実行してみます。
この場合も、シンプルな記述ですみます。
from sagemaker import AlgorithmEstimator from sagemaker.tuner import IntegerParameter, CategoricalParameter, ContinuousParameter, HyperparameterTuner algo = AlgorithmEstimator( algorithm_arn=algorithm_arn, role=role, train_instance_count=1, train_instance_type='ml.c4.xlarge', sagemaker_session=sess, base_job_name='marketplace-sklearn-dt') hyperparameter_ranges = {'max_leaf_nodes': IntegerParameter(10, 100)} tuner = HyperparameterTuner(estimator=algo, base_tuning_job_name='marketplace-sklearn-dt-hyperparameter-tuning', objective_metric_name='validation:accuracy', hyperparameter_ranges=hyperparameter_ranges, max_jobs=2, max_parallel_jobs=2) tuner.fit({'training': data_path}, include_cls_metadata=False) tuner.wait()
ハイパーパラメータチューニングJOBを使ったことがある方にはお馴染みのコードだと思います。
気をつけるのは「AlgorithmEstimator」を使うことと、「アルゴリズムのARNをAlgorithmEstimatorで指定すること」だけです。
5.備考
実際にコードを記述する際に必要な確認事項について2点だけ、下記に記述します。
アルゴリズムのARNの調べ方
こちらはコンソール画面上から確認するのがいいかと思います。
対象のアルゴリズムをクリックして、
ここに記述されています。
チューニング可能なハイパーパラメータの取得
チューニング可能なハイパーパラメータの確認方法について、「SageMakerノートブックインスタンスから」、「コンソール画面上から」の2通りの確認方法について紹介します。
SageMakerノートブックインスタンスから確認する場合は、下記のようなコマンドを実行すると「ハイパーパラメータ名」、「チューニングできるか」、「データ型」、「デフォルト値」等が確認できます。
import sagemaker from sagemaker import get_execution_role from sagemaker import AlgorithmEstimator algorithm_arn = <your argorithm arn> sess = sagemaker.Session() role = get_execution_role() algo = AlgorithmEstimator( algorithm_arn=algorithm_arn, role=role, train_instance_count=1, train_instance_type='ml.c4.xlarge', sagemaker_session=sess, base_job_name='marketplace-sklearn-dt') hyper_param = algo.hyperparameter_definitions.items() print(hyper_param)
dict_items([('max_leaf_nodes', {'spec': {'Name': 'max_leaf_nodes', 'Description': 'Grow a tree with max_leaf_nodes in best-first fashion. Best nodes are defined as relative reduction in impurity. If None then unlimited number of leaf nodes', 'Type': 'Integer', 'Range': {'IntegerParameterRangeSpecification': {'MinValue': '1', 'MaxValue': '100000'}}, 'IsTunable': True, 'IsRequired': False, 'DefaultValue': '100'}, 'range': <sagemaker.parameter.IntegerParameter object at 0x7f4e8bee2e80>, 'class': <class 'sagemaker.parameter.IntegerParameter'>})])
コンソール画面上からは、下記のように取得できます。
まずは対象のアルゴリズムを選択し、「アクション」、「チューニングジョブの作成」とクリックしていき、
「次へ」をクリック
評価指標とする「メトリックス」と「チューニングできるハイパーパラメータ」を確認できます。
6.まとめ
マーケットプレイスに公開されているアルゴリズムを使って、SageMakerノートブック上から学習JOBを実行してみました。
特に複雑な記述が必要になるわけでもないので、とても使いやすいです。
気になるアルゴリズムがある方は、是非「アルゴリズムの無料試用期間」を利用して試してみてください。