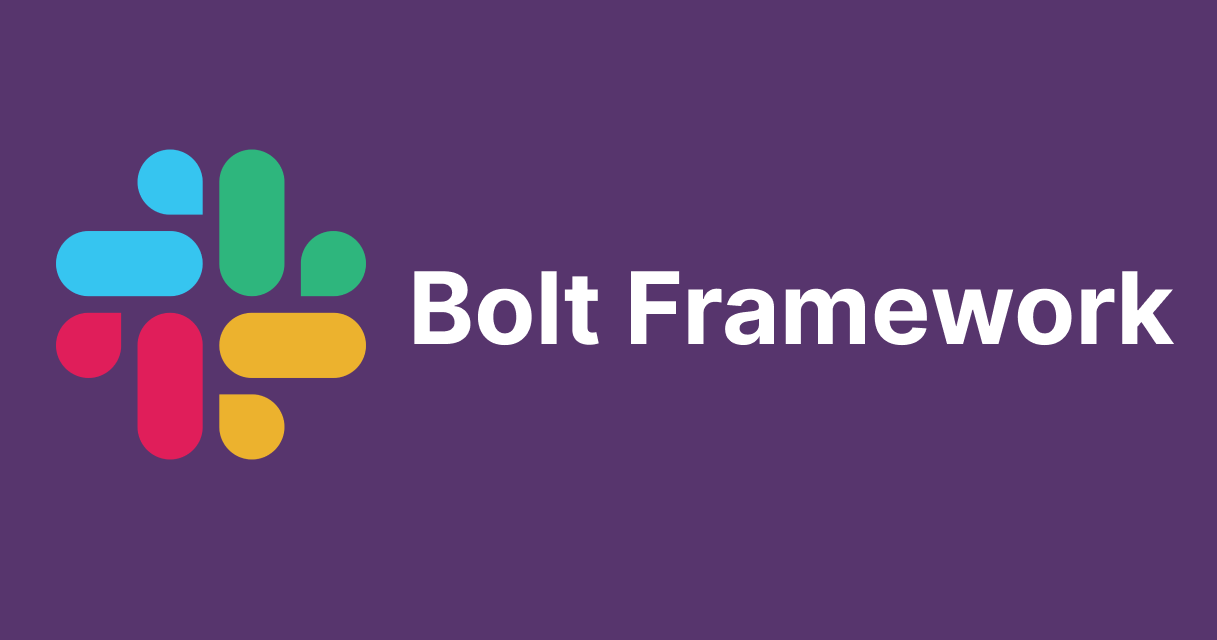
Create a slackbot to send messages on channel using Bolt Framework
Introduction
In this blog post, I have shown how we can create a simple slackbot that is used to send a message on a channel. As we know, slack is a very useful that makes the communication between the coworkers very easy and efficient. According to slack.com, it replaces email with something faster, better organised and more secure.
About Slack Bolt Framework
Bolt is an official Slack framework designed to simplify the creation of Slack apps. It provides an abstraction layer over Slack's APIs, allowing developers to build with less boilerplate code. With Bolt, you can handle events, create commands, and interact with Slack's API in a more intuitive way.
Prerequisites
- A slack account with permissions to create apps
- Node.js and npm installed on the system
- Basic understanding of Typescript and Node.js
Slack App and project setup
To begin, we need to create a slack app on the workspace and give it relevant permissions.
Create your slack app
- Open https://api.slack.com/ and go to "Your Apps".
- Click on "Create New App", input a name and select a workspace
- Under "OAuth & Permissions", Add the scopes
channels:history
chat:write
to the Bot Token Scopes. - Install the app to the workspace and save the Bot User OAuth Token
Initialize your project
Create a new directory for your project and initialize it.
mkdir slackbot-sample-message cd slackbot-sample-message npm init -y
Install the Bolt framework and Typescipt dependencies
npm install @slack/bolt npm install typescipt @types/node dotenv ts-node --save-dev
Initialize Typescipt in your project
npx tsc --init
This will create a tsconfig.json
file.
Enable the socket mode
- Under the Socket Mode , enable the socket mode
- Go to Basic Information and scroll down under the App Token section and click Generate Token and Scopes to generate an app token. Add the
connections:write
scope to this token and save the generatedxapp
token. - Under the Event Subscriptions, toggle to enable the events
- In the subscribe to bot events, add
message.channels
event
- In the subscribe to bot events, add
Program to send Slackbot message
Create a .env file to store the signing-secret and slackbot token from the app
Slack signing secret and app token can be obtained by navigating to the Basic Information, and under App credentials and App-Level Tokens
Slack bot token can be obtained by navigating to the OAuth & Permissions, and under OAuth Tokens for Your Workspace
SLACK_SIGNING_SECRET = "<add-signing-secret>", SLACK_BOT_TOKEN = "<add-slack-bot-token>" SLACK_APP_TOKEN = "<add-slack-app-token>"
Create a file index.ts
and add the following code
import { App, GenericMessageEvent, MessageEvent } from "@slack/bolt"; import * as dotenv from "dotenv"; dotenv.config(); const app: App = new App({ token: process.env.SLACK_BOT_TOKEN, signingSecret: process.env.SLACK_SIGNING_SECRET, socketMode: true, appToken: process.env.SLACK_APP_TOKEN, }); app.message("Hello", async ({ message, say }) => { await say(`Hello there <@${(message as GenericMessageEvent).user}>!`); }); (async (): Promise<void> => { const port: number = Number(process.env.PORT) || 3000; await app.start(port); console.log(`Slackbot is running on port ${port}!`); })();
Add the command to run slackbot
Update the scripts in the package.json file as follows
... "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "dev": "ts-node index.ts" } ...
Execute the following command
npm run dev
Results
Add the slackbot to the channels in which the slackbot has to interact
Send a "Hello" in the channel. The expected result is as follows
Conclusion
We have created a Slackbot using Typescript and Bolt framework that can send a simple message to the Slack channel. A slackbot can be widely used for various purposes like adding polls, buttons which will increase the interactivity with users of the workspace.