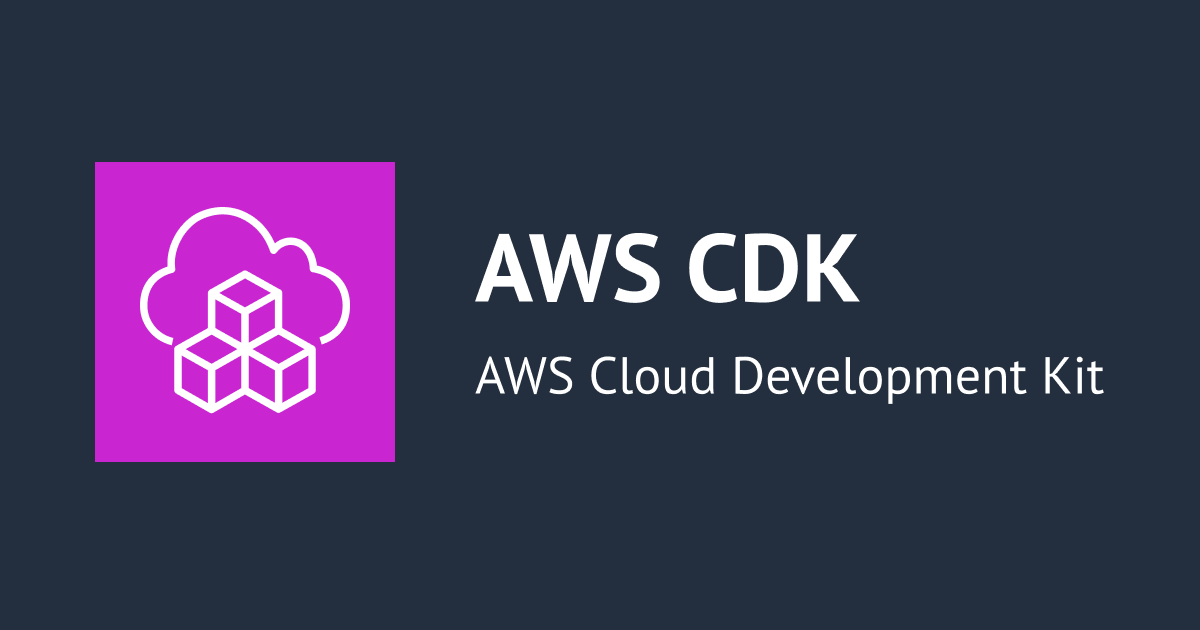
Create API Gateway using AWS CDK
Introduction
In this blog post, I will explain how to a create an API endpoint using API Gateway and AWS CDK. We will use AWS CDK Toolkit, which is a command line tool for interacting with CDK apps. We can use AWS CDK Toolkit to create, manage and deploy AWS CDK projects. AWS CDK supports Typescript, Javascript, Python, Java, C#/.Net and Go.
Prerequisites
- Knowledge of Typescript/Javascript, NodeJS
- AWS Account
- AWS CDK v2
Setup AWS CDK project
In this project, we are going to create a function which will fetch all the timezones from the external URL -> https://worldtimeapi.org/api/timezone
. We will create a lambda handler function to deploy our function that fetches all the timezones.
We will create an API endpoint with method GET
using API gateway and use our Lambda handler function to retrieve all the timezones,
Steps to create a CDK project
mkdir cdk-apigateway
: Create a directory called cdk-apigatewaycd cdk-apigateway
npx cdk init app --language typescript
: Command to initialize a cdk project with language template typescriptnpx cdk ls
: If the output isCdkApigatewayStack
, then your cdk project setup is completed.
Lambda Handler Function
File : <cdk-apigateway/lambda/index.ts>
export const handler = async () => { try { const url = "https://worldtimeapi.org/api/timezone"; const response = await fetch(url); const data = await response.json(); return { statusCode: 200, body: JSON.stringify(data), }; } catch (error) { console.error("Error fetching timezone data:", error); return { statusCode: 500, body: JSON.stringify({ message: "Error fetching timezone data." }), }; } };
Program to create cdk stack
File : <cdk-apigateway/lib/cdk-apigateway-stack.ts>
import * as cdk from "aws-cdk-lib"; import * as lambda from "aws-cdk-lib/aws-lambda"; import * as apigateway from "aws-cdk-lib/aws-apigateway"; import { Construct } from "constructs"; export class CdkApigatewayStack extends cdk.Stack { constructor(scope: Construct, id: string, props?: cdk.StackProps) { super(scope, id, props); /** * Stack of lambda function to get all the timezones */ const getAllTimezonesFunction = new lambda.Function( this, "GetAllTimezonesFunction", { runtime: lambda.Runtime.NODEJS_18_X, handler: "index.handler", code: lambda.Code.fromAsset("lambda"), } ); /** * API gateway stack */ const api = new apigateway.RestApi(this, "MyApiGateway", { restApiName: "MyApi", }); const getAllTimezonesResource = api.root.addResource("timezones"); const getAllTimezonesIntegration = new apigateway.LambdaIntegration( getAllTimezonesFunction ); getAllTimezonesResource.addMethod("GET", getAllTimezonesIntegration); new apigateway.Deployment(this, "MyApiGatewayDeployment", { api, }); } }
- Lambda Function Creation: The code creates a Lambda function named
GetAllTimezonesFunction
using thelambda.Function
construct from the AWS CDK. This function is intended to retrieve all timezones. It's configured with the Node.js 18.x runtime and is deployed from thelambda
directory as the function's code. - API Gateway Configuration: The code sets up an API Gateway named
MyApiGateway
using theapigateway.RestApi
construct. This API is given the name "MyApi". - Resource and Integration: It defines a resource
/timezones
under the root of the API usingapi.root.addResource("timezones")
. This resource is used to organize API endpoints. Then, it creates an integration between the/timezones
resource and theGetAllTimezonesFunction
Lambda function usingapigateway.LambdaIntegration
. This integration allows the API Gateway to forward requests to the Lambda function. - Method Assignment: The code assigns the
GET
method to the/timezones
resource by usinggetAllTimezonesResource.addMethod("GET", getAllTimezonesIntegration)
. This means that when a GET request is made to the/timezones
endpoint of the API, it triggers theGetAllTimezonesFunction
Lambda function. - Deployment: Finally, the code deploys the API using
apigateway.Deployment
. This step is crucial for making the API accessible to clients. It ensures that the API configuration is propagated and deployed to the API Gateway service in AWS.
Results
Now that we have written our code to setup and create the API endpoint, we have to deploy the stack using the following commands.
npx cdk bootstrap
: Initializes the AWS environment, enabling smooth deployment of CDK stacks.npx cdk deploy
: Command used to deploy AWS CDK stacks defined in the project, applying the changes to AWS infrastructure as specified in the code.
In the Outputs, you can see the endpoint is created as follows
After deploying, the API Gateway endpoint is created as follows
When we open the URL https://<apigateway_id>.execute-api.ap-northeast-1.amazonaws.com/prod/timezones
we can see the following result where all the timezones are displayed.
To access the URL, Navigate as follows in AWS console to see the Invoke URL
Conclusion
With the ability to define infrastructure as code, developers can efficiently manage and scale their AWS resources, ultimately streamlining the development process and enhancing overall project agility. By embracing modern tools like AWS CDK, developers can focus more on building innovative solutions while abstracting away the complexities of infrastructure management. AWS CDK offers a flexible and intuitive framework for building and deploying cloud-native applications. By combining the power of CDK with the scalability of API Gateway, developers can create robust APIs that meet the demands of modern web and mobile applications.