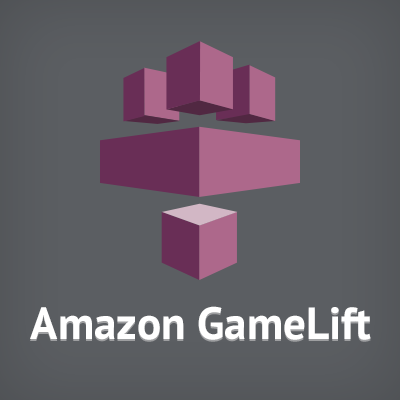
Unityで作成したビルドをGameLiftで起動してみる
GameLiftでは、主要なゲームエンジン (Amazon Lumberyard、Unreal Engine、Unity など) で作成したビルドをGame serversとして起動させることができます。 この記事では主要なゲームエンジンの中でもよく利用されているUnityでビルドを作成し、そのビルドをGameLiftで起動させる方法を紹介したいと思います。
Game serversとGameLift serviceの通信について
GameLiftの全体アーキテクチャのおさらいです。GameLiftにはゲームエンジンで作成したビルドを起動させるためのGame serversと、Game serversを管理するためのGameLift serviceが存在します。
Game serversとGameLift service間では、各フェーズに毎にいくつかの通信が発生しており、 この通信を元にゲームセッション、ユーザーセッションの管理や、Game serversのスケールアウト/インが行われています。
ビルド作成
上記の通信を簡易に実現させるため、AWSからServer SDKが提供されています。 Unreal Engine、Unityについては導入のための簡単な手順も用意されています。
今回はこの手順を元に、Unityでビルドを作成し、GameLiftで起動させてみようと思います。
環境
ビルドを作成するためには以下が必要です。必要に応じてインストールしてください。
- 以下のIDEのいずれか
- Visual Studio 2012 以上
- Xamarin Studio 6.1 以上
- Mono develop 6.1 以上
- .Net framework 3.5
- Unity
この記事ではVisual Studio 2017 を使った手順を紹介します。
Server SDKのダウンロード、ライブラリ構築
まずは、Server SDKをダウンロードし、
Visual Studioにてダウンロードしたソリューションファイル(GameLiftServerSDKNet35.sln)を開きます。
次にNuGet パッケージを復元し、ソリューションを構築します。
プロジェクトをビルドします。
ビルドが完了すると対象のディレクトリに以下のdllが生成されます。
- EngineIoClientDotNet.dll
- GameLiftServerSDKNet35.dll
- log4net.dll
- Newtonsoft.Json.dll
- protobuf-net.dll
- SocketIoClientDotNet.dll
- System.Threading.Tasks.NET35.dll
- WebSocket4Net.dll
UnityプロジェクトへのSDK追加
Unityを起動し、新規にプロジェクトを作成します。
次にAPI の互換性の設定を行います。 Unity エディタで、[File]、[Build Settings]、[Player Settings] の順に選択し、 API の互換性レベルを [.Net 2.0] に設定します。
UnityにServer SDKを追加します。 Unity エディタで、Assets/Plugins ディレクトリを作成し、先ほど作成したdllを配置します。
Game serverの初期化コード追加
Hierarchyに空のGameObjectを作成し、そのオブジェクトにC#のスクリプトを追加します。
using UnityEngine; using Aws.GameLift.Server; using System.Collections.Generic; public class GameLiftServerExampleBehavior : MonoBehaviour { //This is an example of a simple integration with GameLift server SDK that makes game server processes go active on Amazon GameLift public void Start() { var listeningPort = 7777; //InitSDK establishes a local connection with Amazon GameLift's agent to enable further communication. var initSDKOutcome = GameLiftServerAPI.InitSDK(); if (initSDKOutcome.Success) { ProcessParameters processParameters = new ProcessParameters( (gameSession) => { //When a game session is created, GameLift sends an activation request to the game server and passes along the game session object containing game properties and other settings. //Here is where a game server should take action based on the game session object. //Once the game server is ready to receive incoming player connections, it should invoke GameLiftServerAPI.ActivateGameSession() GameLiftServerAPI.ActivateGameSession(); }, () => { //OnProcessTerminate callback. GameLift invokes this callback before shutting down an instance hosting this game server. //It gives this game server a chance to save its state, communicate with services, etc., before being shut down. //In this case, we simply tell GameLift we are indeed going to shut down. GameLiftServerAPI.ProcessEnding(); }, () => { //This is the HealthCheck callback. //GameLift invokes this callback every 60 seconds or so. //Here, a game server might want to check the health of dependencies and such. //Simply return true if healthy, false otherwise. //The game server has 60 seconds to respond with its health status. GameLift will default to 'false' if the game server doesn't respond in time. //In this case, we're always healthy! return true; }, listeningPort, //This game server tells GameLift that it will listen on port 7777 for incoming player connections. new LogParameters(new List<string>() { //Here, the game server tells GameLift what set of files to upload when the game session ends. //GameLift uploads everything specified here for the developers to fetch later. "/local/game/logs/myserver.log" })); //Calling ProcessReady tells GameLift this game server is ready to receive incoming game sessions! var processReadyOutcome = GameLiftServerAPI.ProcessReady(processParameters); if (processReadyOutcome.Success) { print("ProcessReady success."); } else { print("ProcessReady failure : " + processReadyOutcome.Error.ToString()); } } else { print("InitSDK failure : " + initSDKOutcome.Error.ToString()); } } void OnApplicationQuit() { //Make sure to call GameLiftServerAPI.Destroy() when the application quits. This resets the local connection with GameLift's agent. GameLiftServerAPI.Destroy(); } }
ビルド作成
Build Settingを起動後、対象のシーンを追加しビルドを作成します。
Game server起動
アップロード
AWS CLIを使ってビルドをGameLiftにアップロードします。
#Windowsの場合 aws gamelift upload-build --name <your build name> --build-version <your build number> --build-root <local build path> --operating-system WINDOWS_2012 --region ap-northeast-1
アップロードが完了すると、コンソールよりビルドが確認できます。
フリート作成
長くなりましたが、最後にフリートを作成します。
フリートの詳細には、先ほどアップロードしたビルドを選択。
プロセス管理には、ビルドの起動パスと起動パラメータを指定します。Unityで作成したWindows用のビルドをFleetとして起動させる場合は、起動パラメータとして-batchmode
を指定する必要があります。
その他設定値については、一旦デフォルトとします。
ステータス確認
フリートのステータスがアクティブ
となっていればOKです。
フリートのステータスの遷移も確認することができます。
まとめ
簡単にではありますが、Unityで作成したビルドをGameLift上で起動させることができましたー!!やったね