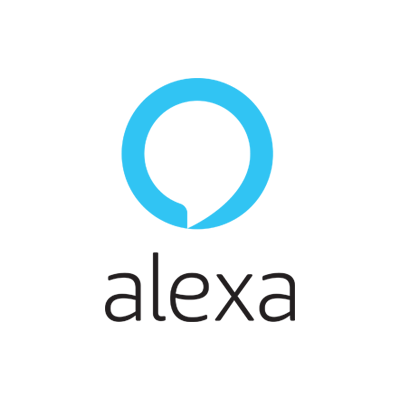
対話モデルを元にLambdaテンプレートを生成してくれる「ASK-SDK Code Generator」を試す
はじめに
AlexaのバックエンドをLambdaで実装する場合、コードテンプレートとしてLambdaのBlueprint(設計図)を活用すると便利です。
但し、2018年8月7日現在、LambdaのBlueprintはv1(alexa-sdk)のコードしか設計図がなく、v2(ask-sdk)のコードのBlueprintは存在しません。
できれば、v2のコードもスキル開発時にテンプレート的なものがあると助かりますね。
今回は、Alexaの対話モデル(JSON)からバックエンドのLambdaテンプレート(v2/Node.js)を自動生成してくれる「ASK-SDK Code Generator」という便利なツールをご紹介します。
対話モデルの準備
まず、コード生成元となるスキルの対話モデルを準備します。
開発者コンソールで簡単なスキルを作ってみます。
初期状態にHelloIntent
を追加しただけの単純なものになります。
作成後モデルをビルドし、「JSONエディター」を開くと、対話モデルが以下のようなJSONで表示されますので、コピーしておきます。
{ "interactionModel": { "languageModel": { "invocationName": "ハローワールド", "intents": [ { "name": "AMAZON.CancelIntent", "samples": [] }, { "name": "AMAZON.HelpIntent", "samples": [] }, { "name": "AMAZON.StopIntent", "samples": [] }, { "name": "HelloIntent", "slots": [], "samples": [ "どうも", "こんちは", "ハロー", "こんにちは" ] } ], "types": [] } } }
コードジェネレータを試してみる
ASK-SDK Code Generatorにアクセスします。
画面左側のLanguage Model JSONに先程コピーしたJSONをペーストします。
GENERATE CODEボタンをクリックすると、すぐにコードが生成されます。
それでは、生成されたコードを一部見てみましょう。
// 1. Intent Handlers ============================================= const AMAZON_CancelIntent_Handler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'IntentRequest' && request.intent.name === 'AMAZON.CancelIntent' ; }, handle(handlerInput) { const request = handlerInput.requestEnvelope.request; const responseBuilder = handlerInput.responseBuilder; let sessionAttributes = handlerInput.attributesManager.getSessionAttributes(); let say = 'Okay, talk to you later! '; return responseBuilder .speak(say) .withShouldEndSession(true) .getResponse(); }, }; const AMAZON_HelpIntent_Handler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'IntentRequest' && request.intent.name === 'AMAZON.HelpIntent' ; }, handle(handlerInput) { const request = handlerInput.requestEnvelope.request; const responseBuilder = handlerInput.responseBuilder; let sessionAttributes = handlerInput.attributesManager.getSessionAttributes(); let intents = getCustomIntents(); let sampleIntent = randomElement(intents); let say = 'You asked for help. '; // let previousIntent = getPreviousIntent(sessionAttributes); // if (previousIntent && !handlerInput.requestEnvelope.session.new) { // say += 'Your last intent was ' + previousIntent + '. '; // } // say += 'I understand ' + intents.length + ' intents, ' say += ' Here something you can ask me, ' + getSampleUtterance(sampleIntent); return responseBuilder .speak(say) .reprompt('try again, ' + say) .getResponse(); }, }; const AMAZON_StopIntent_Handler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'IntentRequest' && request.intent.name === 'AMAZON.StopIntent' ; }, handle(handlerInput) { const request = handlerInput.requestEnvelope.request; const responseBuilder = handlerInput.responseBuilder; let sessionAttributes = handlerInput.attributesManager.getSessionAttributes(); let say = 'Okay, talk to you later! '; return responseBuilder .speak(say) .withShouldEndSession(true) .getResponse(); }, }; const HelloIntent_Handler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'IntentRequest' && request.intent.name === 'HelloIntent' ; }, handle(handlerInput) { const request = handlerInput.requestEnvelope.request; const responseBuilder = handlerInput.responseBuilder; let sessionAttributes = handlerInput.attributesManager.getSessionAttributes(); let say = 'Hello from HelloIntent. '; return responseBuilder .speak(say) .reprompt('try again, ' + say) .getResponse(); }, }; const LaunchRequest_Handler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'LaunchRequest'; }, handle(handlerInput) { const responseBuilder = handlerInput.responseBuilder; let say = 'hello' + ' and welcome to ' + invocationName + ' ! Say help to hear some options.'; let skillTitle = capitalize(invocationName); return responseBuilder .speak(say) .reprompt('try again, ' + say) .withStandardCard('Welcome!', 'Hello!\nThis is a card for your skill, ' + skillTitle, welcomeCardImg.smallImageUrl, welcomeCardImg.largeImageUrl) .getResponse(); }, }; const SessionEndedHandler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'SessionEndedRequest'; }, handle(handlerInput) { console.log(`Session ended with reason: ${handlerInput.requestEnvelope.request.reason}`); return handlerInput.responseBuilder.getResponse(); } }; const ErrorHandler = { canHandle() { return true; }, handle(handlerInput, error) { const request = handlerInput.requestEnvelope.request; console.log(`Error handled: ${error.message}`); // console.log(`Original Request was: ${JSON.stringify(request, null, 2)}`); return handlerInput.responseBuilder .speak('Sorry, an error occurred. Please say again.') .reprompt('Sorry, an error occurred. Please say again.') .getResponse(); } };
HelloIntent
も含め、対話モデルで定義したインテントのハンドラが書かれています。
起動時のLaunchRequestやエラーハンドラも書かれていますね。
// 4. Exports handler function and setup =================================================== const skillBuilder = Alexa.SkillBuilders.standard(); exports.handler = skillBuilder .addRequestHandlers( AMAZON_CancelIntent_Handler, AMAZON_HelpIntent_Handler, AMAZON_StopIntent_Handler, HelloIntent_Handler, LaunchRequest_Handler, SessionEndedHandler ) .addErrorHandlers(ErrorHandler) .addRequestInterceptors(InitMemoryAttributesInterceptor) .addRequestInterceptors(RequestHistoryInterceptor) // .addResponseInterceptors(ResponseRecordSpeechOutputInterceptor) // .addRequestInterceptors(RequestPersistenceInterceptor) // .addResponseInterceptors(ResponsePersistenceInterceptor) // .withTableName("askMemorySkillTable") // .withAutoCreateTable(true) .lambda();
exports.hanlder
ではインテントのハンドラとエラーハンドラ、セッション情報に関するインターセプター(ハンドラの前処理)がそれぞれ追加されています。
データ永続化に関する処理も用意されていますが、生成時はコメントアウトされています。
// 3. Helper Functions =================================================================== function capitalize(myString) { return myString.replace(/(?:^|\s)\S/g, function(a) { return a.toUpperCase(); }) ; } function randomElement(myArray) { return(myArray[Math.floor(Math.random() * myArray.length)]); } function stripSpeak(str) { return(str.replace('<speak>', '').replace('</speak>', '')); } function getSlotValues(filledSlots) { const slotValues = {}; Object.keys(filledSlots).forEach((item) => { const name = filledSlots[item].name; if (filledSlots[item] && filledSlots[item].resolutions && filledSlots[item].resolutions.resolutionsPerAuthority[0] && filledSlots[item].resolutions.resolutionsPerAuthority[0].status && filledSlots[item].resolutions.resolutionsPerAuthority[0].status.code) { switch (filledSlots[item].resolutions.resolutionsPerAuthority[0].status.code) { case 'ER_SUCCESS_MATCH': slotValues[name] = { heardAs: filledSlots[item].value, resolved: filledSlots[item].resolutions.resolutionsPerAuthority[0].values[0].value.name, ERstatus: 'ER_SUCCESS_MATCH' }; break; case 'ER_SUCCESS_NO_MATCH': slotValues[name] = { heardAs: filledSlots[item].value, resolved: '', ERstatus: 'ER_SUCCESS_NO_MATCH' }; break; default: break; } } else { slotValues[name] = { heardAs: filledSlots[item].value, resolved: '', ERstatus: '' }; } }, this); return slotValues; } (以下省略)
フレーズのランダム化やスロット値の取得など、便利なヘルパー関数も実装されています。
先程追加されていたインターセプターの定義もこちらで書かれています。
また、コード生成時のオプションとして以下を利用することもできます。
- Variety Greeting(スキル起動時挨拶のランダム化)
- Echo display welcome screen(ディスプレイ付きEchoのスキル起動時スクリーン表示)
const LaunchRequest_Handler = { canHandle(handlerInput) { const request = handlerInput.requestEnvelope.request; return request.type === 'LaunchRequest'; }, handle(handlerInput) { const responseBuilder = handlerInput.responseBuilder; // `Variety Greeting` Option let say = randomElement(['hello','hi', 'greetings']) + ' and welcome to ' + invocationName + ' ! Say help to hear some options.'; let skillTitle = capitalize(invocationName); // `Echo display welcome screen` Option if (supportsDisplay(handlerInput)) { const myImage1 = new Alexa.ImageHelper() .addImageInstance(DisplayImg1.url) .getImage(); const myImage2 = new Alexa.ImageHelper() .addImageInstance(DisplayImg2.url) .getImage(); const primaryText = new Alexa.RichTextContentHelper() .withPrimaryText('Welcome to the skill!') .getTextContent(); responseBuilder.addRenderTemplateDirective({ type : 'BodyTemplate2', token : 'string', backButton : 'HIDDEN', backgroundImage: myImage2, image: myImage1, title: skillTitle, textContent: primaryText, }); } return responseBuilder .speak(say) .reprompt('try again, ' + say) .withStandardCard('Welcome!', 'Hello!\nThis is a card for your skill, ' + skillTitle, welcomeCardImg.smallImageUrl, welcomeCardImg.largeImageUrl) .getResponse(); }, };
先日発売されたEcho Spot対応のスキル開発も、サクッと取りかかれそうですね。
おわりに
Alexaの対話モデルからASK SDK(v2)のLambdaコードを自動で生成してくれる、「ASK-SDK Code Generator」を試してみました。
対話モデルを準備しておけば、最低限必要な実装がされたv2のテンプレートを簡単に生成することができます。
これらのツールを活用することで、これからスキル開発をしようと思っている方もバックエンド側の開発に取り掛かりやすくなるのではないでしょうか。
それでは。