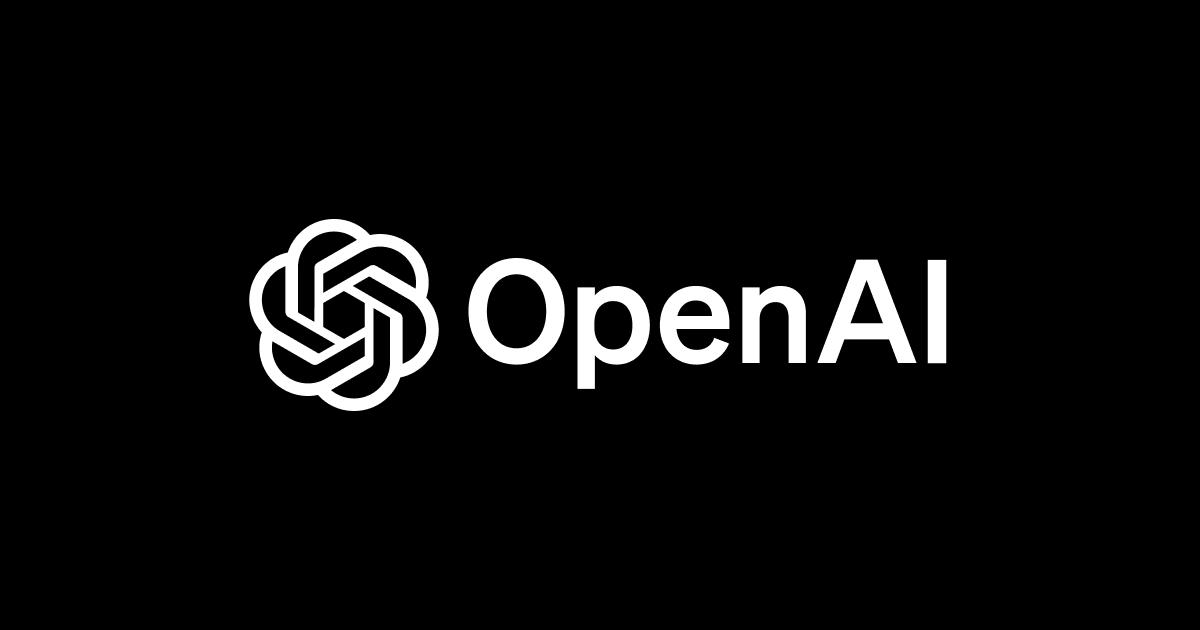
Using OpenAI and Dalle to generate a recipe and Image for the list of ingredients provided
Introduction
In this blog let's aim at Openai's Completion API and Image API to return us with a recipe and appropriate image for the list of ingredients provided. As a prerequisite, you should have an OpenAI account, API key, and Juypiter environment to work with. For a detailed step-by-step explanation of the mentioned prerequisite check out this blog from steps 1 to 4.
Now let's get into the action
Step 1: Create a Prompt
The prompt is the most important attribute of OpenAI parameters. For the recipe generator let's provide the list of ingredients and also prompt the API to start off the response with 'recipe name', 'time required to cook' 'ingredients list', and 'step-by-step procedure for cooking'.
def create_dish_prompt(list_items): prompt = f"create a detailed recipe with only the following ingredients: {','.join(list_items)}. \n"\ +f"Start off with 'recipe name', 'time required to cook' 'ingredients list' and 'step-by-step procedure for cooking " return prompt
Note: In this line ','.join(list_items)
--> I'm extracting the items from the list to concat with a delimiter ,
using the join function. Let's see an example of how this prompt is turned into.
Step 2: Call OpenAI Completion API
openai.Completion.create
is a method provided by the OpenAI Python client library that allows you to make requests to the OpenAI Completion API. In the below example, we are calling the Completion API with certain parameters. In the prompt, we are requesting a recipe for items = ['wheat', 'carrot', 'egg']
. Max_tokens are set to 512
as it's a descriptive response and temperature to 0.7
. Temperature is set to a higher value than the default value to allow the API to be creative while responding.
response = openai.Completion.create( engine='text-davinci-003', prompt = create_dish_prompt(items), max_tokens=512, temperature=0.7 )
Here's the response of the above API.
The response is as per our instruction. OpenAI is suggesting a Carrot Wheat Egg Cake
recipe for the given list of items, with the duration, ingredients, and procedure. That's Quite amazing, right?
Step 3: Call Image API
Extract recipe name
Rather than manually providing the recipe name, let's extract it from the response string using a regular expression.
- For the regular expression, let's import the
re
library.
import re def extract_title(text): return re.findall('^.*Recipe Name: .*$', text, re.MULTILINE)[0].strip().split('Recipe Name: ')[-1]
Explanation of regexp:
- From the multiline response phrase we are extracting the part of the key pair that we encounter with
Recipe Name:
like this'^.*Recipe Name: .*$'
. Beware to put in the same text from the response that you want to grep with, although you type in the same letters, the spacing distance is also matched, so I would recommend copying. -
Now with this
re.findall('^.*Recipe Name: .*$', text, re.MULTILINE)
--> response is:['Recipe Name: Carrot Wheat Egg Cake']
- Next by
[0].strip()
we are truncating the 0th location object as such -->'Recipe Name: Carrot Wheat Egg Cake'
- Next by
.split('Recipe Name: ')
--> we have result as['', 'Carrot Wheat Egg Cake']
- Next by [-1] --> we are fetching the last object which is our resultant Recipe name. -->
'Carrot Wheat Egg Cake'
Call DALL.E Model
With openai.Image.create
we can generate or manipulate images with DALL·E models. It's still a beta model that allows you to create an original image given a text prompt. Generated images can have a size of 256x256, 512x512, or 1024x1024 pixels. We can develop 1-10 images in one go, by using the n
parameter.
img = openai.Image.create( prompt= extract_title(response['choices'][0]['text']) #extract only recipe name. n=1, size='512x512' )
The response for the following:
Even here we can play around with prompts to get a better image or image of our choice.
Let's define the prompt to get us professional food photography with snowy Christmas theme background.
def trail2_prompt(title): prompt = f"{title}, professional food photography with snowy Christmas theme background" return prompt
img = openai.Image.create( prompt=trail2_prompt(extracted_receipe_title), n=1, size='512x512' )
For this, my response image was something like this.
With little prompting, a beta version API is able to achieve this extent of image identification and production. I find it incredible.
another example
For my list of items: Tomato, Bread and Egg
, Openai.Completion API suggested a Tomato Bread Omelette
recipe and openai.Image API suggested the following image.
Try yourself and learn more..!!