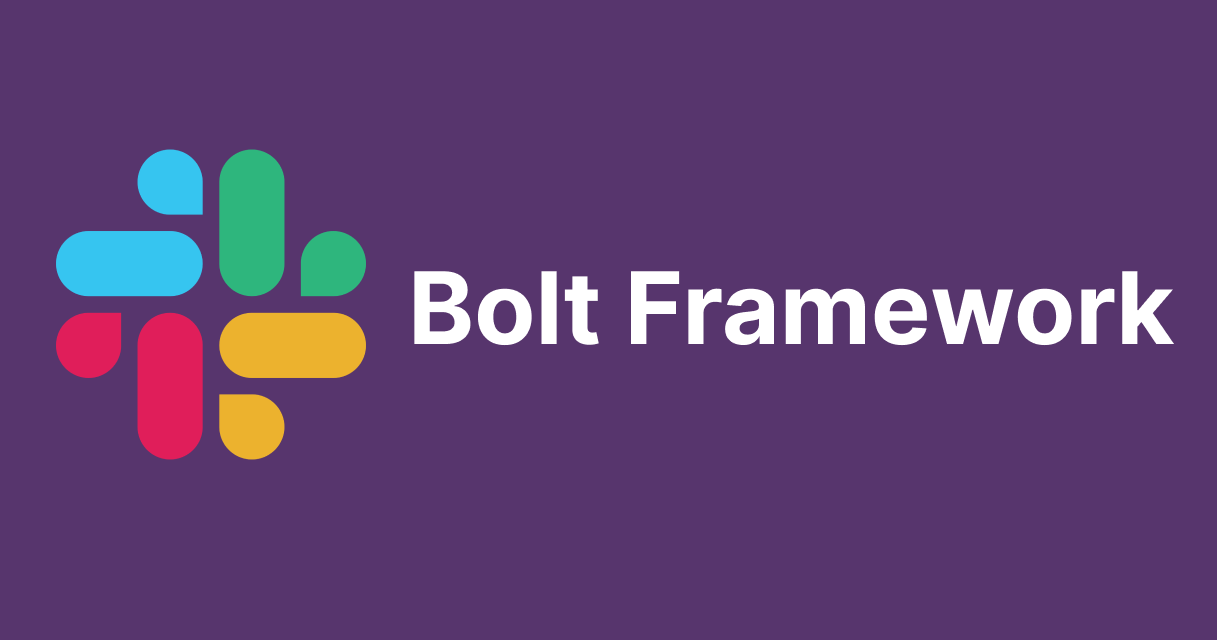
Slackbot slash command and messages with buttons
Introduction
In this blog post, I have shown how we can create slackbot which uses slash commands and also shown how we can have buttons in the messages. In my previous blog, I have also explained how we can setup the slack app and create a simple slackbot. Here is the link -> https://dev.classmethod.jp/articles/create-a-slackbot-to-send-messages-on-channel-using-bolt-framework/
Prerequisites
- A slack account with permissions to create apps
- Node.js and npm installed on the system
- Basic understanding of Typescript and Node.js
Setup the slash commands on slack app
- Navigate to the slash commands and click on Create New Command
- Enter the details such as slash command text. In our example, we have given
/echo
in the Command input field. Enter the Request URL. - Click on the save button to create the slash command on the slack app.
Program to create a slash command
const app: App = new App({ token: process.env.SLACK_BOT_TOKEN, signingSecret: process.env.SLACK_SIGNING_SECRET, socketMode: true, appToken: process.env.SLACK_APP_TOKEN, port: 3000, endpoints: ["/slack/events", "/slack/commands"], }); // この echo コマンドは ただ、その引数を(やまびこのように)おうむ返しする app.command("/echo", async ({ command, ack, respond }) => { // コマンドリクエストを確認 await ack(); await respond(`${command.text}`); });
- We can use the
app.command
method to respond to the slash command. - In our example, if the user types
/echo
command and writes any text after the slash command then the slackbot responds with the same text.
Result
Program to add buttons to the slackbot message
const app: App = new App({ token: process.env.SLACK_BOT_TOKEN, signingSecret: process.env.SLACK_SIGNING_SECRET, socketMode: true, appToken: process.env.SLACK_APP_TOKEN, port: 3000, endpoints: ["/slack/events", "/slack/commands"], }); // Define the menu options const menuOptions = [ { text: "カレーライス", value: "option_1" }, { text: "みそ汁", value: "option_2" }, { text: "ハンバーガー", value: "option_3" }, ]; app.command("/menu", async ({ ack, body, client }) => { await ack(); try { await client.chat.postMessage({ channel: body.channel_id, text: "本日のメニュー", attachments: [ { fallback: "本日のメニュー", color: "#36a64f", text: "オプションを選択してください", actions: menuOptions.map((option) => ({ type: "button", text: option.text, name: option.value, value: option.value, action_id: option.value, })), }, ], }); } catch (error) { console.error(`Error sending message: ${error}`); } });
- The code sets up a Slack bot using the Bolt for JavaScript framework, configuring it with tokens, endpoints, and options for handling Slack events.
- It defines an array of menu options, each containing text and a corresponding value.
- A command handler is established for the
/menu
command, which triggers the bot to acknowledge the command and send a message to the channel with menu options displayed as clickable buttons. - Each menu option is transformed into a button element within the message attachment, allowing users to select their desired option.
- Upon user interaction with the buttons, the bot listens for and processes these interactions, enabling dynamic interaction with the menu options within Slack.
Result
Conclusion
In conclusion, this blog post has demonstrated the creation of a Slack bot using Bolt for JavaScript, focusing on implementing slash commands and incorporating interactive message elements like buttons. By following the outlined steps, readers can set up a Slack app, define slash commands, and handle user interactions effectively within Slack channels. The provided code examples illustrate how to acknowledge commands, respond with messages containing menu options, and enable users to interact dynamically by selecting options via buttons. This approach enhances user engagement and streamlines communication workflows within Slack environments. Overall, by leveraging the capabilities of Bolt for JavaScript and Slack's interactive features, developers can create versatile and user-friendly bots tailored to meet various organizational needs and enhance team collaboration.