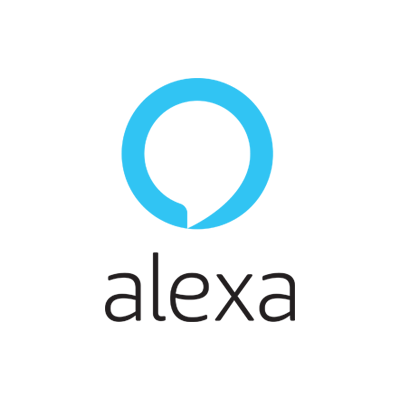
How to store persistence attributes into Amazon S3 using Alexa Skills Kit SDK for Node.js
この記事には日本語版があります。
Introduction
If you want to persist the data which are used by Alexa skills, It's easy by using the API called AttributesManager of Alexa Skills Kit(ASK) SDK for Node.js. The AttributesManager uses Amazon DynamoDB as a persistence layer by default, but you can use any of the data persistence services by using the API called PersistenceAdapter.
In this post, you can find how to use S3PersistenceAdapter, a PersistenceAdapter to be able to use Amazon S3 as a data persistence service.
Sample skill overview
I have created a dummy skill which simulates taking an order of a cafe as a demonstration of PersistenceAdapter. You can order the menu previously ordered by saying something like "I'll have my usual". The previously ordered menu is stored in Amazon S3 via the S3PersistenceAdapter. The following are the possible conversation with the skill.
First skill invocation:
User: "Alexa open persistent caffe." Alexa: "Welcome! What would you like to drink? I have coffee, tea, green tea, and coke." User: "a coffee, please." Alexa: "'Alright! Your coffee will be served soon!"
Second skill invocation:
User: "Alexa open persistent caffe." Alexa: "Welcome! What would you like to drink? I have coffee, tea, green tea, and coke." User: "I'll have my usual." Alexa: "Got it. I will make your usual coffee!"
First skill invocation without previously ordered menu:
User: "Alexa open persistent caffe." Alexa: "Welcome! What would you like to drink? I have coffee, tea, green tea, and coke." User: "I'll have my usual." Alexa: "It seems like you are coming to this store for the first time! What would you like to order? I have coffee, tea, green tea, and coke."
Preparation
- Install ask-sdk-s3-persistence-adapter
- A PersistenceAdapter for DynamoDB is installed together when you install the ASK SDK for Node.js, but S3PersistenceAdapter is not. So you have to install it separately.
- Create an S3 bucket for storing persistent attributes
- You can use the withAutoCreateTable helper function to automatically create a DynamoDB table for your skill when using DynamoDB. On the other hand, you have to create an S3 bucket for your skill before skill invocation since there is no such helper function for S3.
- Add IAM policy for accessing S3 to the IAM role of the skill's Lambda
How to use S3PersistenceAdapter
The following are the excerpt from the sample skill project on GitHub I created for this post.
Load S3PersistenceAdapter module:
import * as Adapter from 'ask-sdk-s3-persistence-adapter';
Instantiate S3PersistenceAdapter object and set it to the SkillBuilder object:
// Instantiate S3PersistenceAdapter object const S3BUCKET_NAME = process.env['S3BUCKET_NAME'] as string; const config = { bucketName: S3BUCKET_NAME }; const S3Adapter = new Adapter.S3PersistenceAdapter(config); // Main process as a Lambda function exports.handler = Alexa.SkillBuilders.custom() .addRequestHandlers( LaunchRequestHandler, OrderIntentHandler, RegularOrderIntentHandler ) .addErrorHandlers(ErrorHandler) .withPersistenceAdapter(S3Adapter) // Set a S3PersistenceAdapter object to the SkillBuilder object .lambda();
Retrieving and Storing persistent attributes:
const {attributesManager} = handlerInput; // Retrieve parsistent attributes object const attributes = await attributesManager.getPersistentAttributes(); // Set a value to the persistent attributes object attributes.drink = drink; attributesManager.setPersistentAttributes(attributes); // Store persistent attributes object await attributesManager.savePersistentAttributes();
Persistent attributes stored on Amazon S3
The followings are the actual result of the dummy skill execution.
An order like "I'll have my usual." is not accepted since there is no persistent attribute stored.
After the first ordering, a file (object) is created for each user ID in the S3 bucket created for the skill.
This file is a JSON format text file, and the order is saved as follows.
This time, an order like "I'll have my usual." is accepted by referencing the persistent attribute saved before.
Supplementary information
ObjectKeyGenerator
You can use any key other than userId by giving an S3PersistentAdapter constructor an ObjectKeyGenerator object. ASK SDK provides an example ObjectKeyGenerator implementation which uses deviceId as a key.
Data Consistency Model
Amazon S3 has a different consistency model from Amazon DynamoDB. You should use DynamoDB if you need real-time data consistency for persistent attributes of your Alexa skill.
UserId maintained in different language settings
A user's userId does not change during a skill is enabled. This is true even when language settings of a skill has changed.
Conclusion
An Alexa skill's attributes can be persisted easily by using AttributesManager API provided in ASK SDK. The default data persistence service is Amazon DynamoDB, but you can change it easiliy via PersistentAdapter API to another data persistence service such as Amazon S3.
Thank you for reading until the end. I would be happy if you could share this article with SNS. I'm waiting for comments too!